实现一个多线程的文件下载器,每个线程分别下载文件的不同部分,最后将所有部分合并成一个完整的文件。java实例代码
时间: 2023-09-06 22:05:07 浏览: 109
### 回答1:
实现一个多线程文件下载器可以使用Java的多线程技术,比如使用Java中的Thread类或者使用线程池等技术。具体实现可以参考以下代码示例:
```java
import java.io.*;
import java.net.*;
import java.util.concurrent.*;
public class MultiThreadDownloader {
private static final int NUM_THREADS = 5;
private static final int BUFFER_SIZE = 1024;
public static void download(String url, String fileName) throws Exception {
URL u = new URL(url);
URLConnection conn = u.openConnection();
int contentLength = conn.getContentLength();
if (contentLength < 1) {
throw new IOException("无法获取文件长度");
}
ExecutorService executor = Executors.newFixedThreadPool(NUM_THREADS);
int partSize = contentLength / NUM_THREADS;
int start = 0;
for (int i = 0; i < NUM_THREADS; i++) {
int end = start + partSize - 1;
if (i == NUM_THREADS - 1) {
end = contentLength - 1;
}
DownloadThread thread = new DownloadThread(url, start, end, fileName);
executor.execute(thread);
start = end + 1;
}
executor.shutdown();
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.DAYS);
}
private static class DownloadThread implements Runnable {
private String url;
private int start;
private int end;
private String fileName;
public DownloadThread(String url, int start, int end, String fileName) {
this.url = url;
this.start = start;
this.end = end;
this.fileName = fileName;
}
public void run() {
try {
URL u = new URL(url);
URLConnection conn = u.openConnection();
InputStream is = conn.getInputStream();
RandomAccessFile raf = new RandomAccessFile(fileName, "rw");
raf.seek(start);
int len = 0;
byte[] buffer = new byte[BUFFER_SIZE];
while ((len = is.read(buffer)) != -1) {
raf.write(buffer, 0, len);
}
raf.close();
is.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) throws Exception {
download("https://www.example.com/example.zip", "example.zip");
}
}
```
上面的代
### 回答2:
实现一个多线程的文件下载器可以使用Java中的多线程和文件操作功能。
首先,我们需要创建一个代表文件下载的类,例如FileDownloader。在该类中,我们需要定义要下载的文件的URL、下载线程数、单个线程下载的文件块大小以及要存储下载文件的目标路径。
然后,我们需要创建一个下载任务线程类,例如DownloadTaskThread。每个线程将负责下载文件的一部分数据。在DownloadTaskThread中,我们需要定义文件块的起始位置和结束位置,并在run方法中使用流来从服务器下载文件数据,并将其保存到指定的目标路径中。
在FileDownloader类中,我们需要使用继承Thread类的DownloadTaskThread创建并启动下载线程。每个线程都会负责下载文件的一部分。
最后,当所有线程都完成下载后,我们需要将文件块合并为一个完整的文件。我们可以通过读取每个文件块的数据并写入一个输出流中来实现文件合并。
下面是一个简单的Java示例代码:
```java
import java.io.*;
import java.net.URL;
public class FileDownloader {
private String fileUrl;
private int threadCount;
private int blockSize;
private String targetPath;
public FileDownloader(String fileUrl, int threadCount, int blockSize, String targetPath) {
this.fileUrl = fileUrl;
this.threadCount = threadCount;
this.blockSize = blockSize;
this.targetPath = targetPath;
}
public void startDownload() throws IOException {
URL url = new URL(fileUrl);
long fileSize = url.openConnection().getContentLength();
RandomAccessFile outputFile = new RandomAccessFile(targetPath, "rw");
outputFile.setLength(fileSize);
outputFile.close();
long startPos, endPos;
for (int i = 0; i < threadCount; i++) {
startPos = i * blockSize;
endPos = (i + 1) * blockSize - 1;
if (i == threadCount - 1) {
endPos = fileSize - 1;
}
DownloadTaskThread thread = new DownloadTaskThread(fileUrl, startPos, endPos, targetPath);
thread.start();
}
}
public static void mergeFiles(String targetPath, int threadCount) throws IOException {
RandomAccessFile outputFile = new RandomAccessFile(targetPath, "rw");
for (int i = 0; i < threadCount; i++) {
String blockPath = getBlockPath(targetPath, i);
FileInputStream blockFileInput = new FileInputStream(blockPath);
byte[] buffer = new byte[blockFileInput.available()];
blockFileInput.read(buffer);
blockFileInput.close();
outputFile.seek(i * buffer.length);
outputFile.write(buffer);
}
outputFile.close();
}
private static String getBlockPath(String targetPath, int blockIndex) {
return targetPath + ".tmp" + blockIndex;
}
private class DownloadTaskThread extends Thread {
private String fileUrl;
private long startPos;
private long endPos;
private String targetPath;
public DownloadTaskThread(String fileUrl, long startPos, long endPos, String targetPath) {
this.fileUrl = fileUrl;
this.startPos = startPos;
this.endPos = endPos;
this.targetPath = targetPath;
}
public void run() {
try {
String blockPath = getBlockPath(targetPath, (int) (startPos / blockSize));
URL url = new URL(fileUrl);
RandomAccessFile outputFile = new RandomAccessFile(blockPath, "rw");
outputFile.seek(startPos);
InputStream inputStream = url.openStream();
inputStream.skip(startPos);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1 && startPos < endPos) {
outputFile.write(buffer, 0, bytesRead);
startPos += bytesRead;
}
inputStream.close();
outputFile.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
// 使用方法示例
public class Main {
public static void main(String[] args) {
String fileUrl = "http://example.com/file-to-download.txt";
int threadCount = 4;
int blockSize = 1024;
String targetPath = "C:/path-to-save/downloaded-file.txt";
try {
FileDownloader downloader = new FileDownloader(fileUrl, threadCount, blockSize, targetPath);
downloader.startDownload();
// 等待所有线程完成下载
for (int i = 0; i < threadCount; i++) {
Thread.currentThread().sleep(1000); // 休眠1秒
}
// 合并文件块
FileDownloader.mergeFiles(targetPath, threadCount);
System.out.println("文件下载完成!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上是一个简单的多线程文件下载器的Java实例代码,其中包括了下载线程和文件合并的具体实现。代码可以从指定的URL下载文件,并将下载的文件分为多个线程并行下载,最后将所有文件块合并为一个完整的文件。
### 回答3:
下面是一个使用Java实现多线程文件下载器的示例代码:
```java
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URL;
import java.util.concurrent.CountDownLatch;
public class MultiThreadFileDownloader {
private static final int NUM_THREADS = 4; // 线程数量
private static final String FILE_URL = "http://example.com/file.pdf"; // 文件URL
private static final String OUTPUT_FILE = "output.pdf"; // 输出文件名
public static void main(String[] args) {
try {
downloadFile(FILE_URL, OUTPUT_FILE);
} catch (IOException e) {
e.printStackTrace();
}
}
private static void downloadFile(String fileUrl, String outputFileName) throws IOException {
URL url = new URL(fileUrl);
long fileSize = url.openConnection().getContentLength();
long partSize = fileSize / NUM_THREADS; // 计算每个线程下载的文件部分大小
long remainingBytes = fileSize - (partSize * NUM_THREADS); // 剩余未分配的字节数
CountDownLatch latch = new CountDownLatch(NUM_THREADS); // 使用CountDownLatch来等待所有线程完成
for (int i = 0; i < NUM_THREADS; i++) {
long startByte = i * partSize;
long endByte = (i + 1) * partSize - 1;
if (i == NUM_THREADS - 1) {
endByte += remainingBytes; // 最后一个线程处理剩余的字节
}
Thread thread = new Thread(() -> {
try {
downloadPart(url, startByte, endByte, outputFileName);
} catch (IOException e) {
e.printStackTrace();
} finally {
latch.countDown(); // 任务完成后减少CountDownLatch的计数
}
});
thread.start();
}
try {
latch.await(); // 等待所有线程完成
} catch (InterruptedException e) {
e.printStackTrace();
}
// 合并下载的文件部分
mergeFileParts(outputFileName);
}
private static void downloadPart(URL url, long startByte, long endByte, String outputFileName) throws IOException {
try (BufferedInputStream in = new BufferedInputStream(url.openStream());
FileOutputStream out = new FileOutputStream(outputFileName, true)) {
byte[] buffer = new byte[4096]; // 缓冲区大小
int bytesRead;
// 定位到所需的文件部分起始字节位置
in.skip(startByte);
while ((bytesRead = in.read(buffer)) != -1 && in.available() <= (endByte - startByte + 1)) {
out.write(buffer, 0, bytesRead);
}
}
}
private static void mergeFileParts(String outputFileName) {
try (FileOutputStream out = new FileOutputStream(new File(outputFileName), true)) {
for (int i = 0; i < NUM_THREADS; i++) {
String partFileName = "part" + i + ".tmp"; // 拼接临时文件名
File partFile = new File(partFileName);
try (BufferedInputStream in = new BufferedInputStream(partFile.toURI().toURL().openStream())) {
byte[] buffer = new byte[4096]; // 缓冲区大小
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
}
partFile.delete(); // 删除临时文件
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个示例使用了4个线程来并行下载文件的不同部分,并将它们合并成一个完整的文件。该程序首先计算每个线程应该下载的文件部分的起始字节和结束字节位置,然后创建一个线程来下载相应的部分。每个线程下载完成后,使用CountDownLatch来进行线程同步,以确保所有线程都完成后进行文件合并的操作。合并文件部分时,使用临时文件来存储每个线程下载的部分内容,并最终将它们合并到输出文件中。
相关推荐
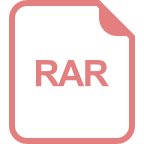
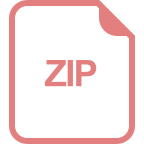
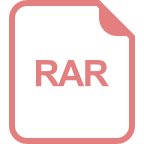















