在Keil中51读取18B20的数据,在LCD1602上显示
时间: 2024-02-13 12:02:34 浏览: 18
好的,以下是基于Keil中51单片机的18B20温度传感器数据读取和LCD1602液晶屏显示的代码实现流程:
1. 定义相关宏定义:
```c
#define DS18B20_GPIO_PORT P3
#define DS18B20_GPIO_PIN 6
```
2. 函数定义:
```c
void delay_us(u16 i)
{
while(i--);
}
void DS18B20_Reset()
{
DS18B20_GPIO_PORT &= ~(1<<DS18B20_GPIO_PIN);
delay_us(750);
DS18B20_GPIO_PORT |= (1<<DS18B20_GPIO_PIN);
delay_us(15);
}
void DS18B20_Write_Byte(u8 dat)
{
u8 i;
for(i=0; i<8; i++)
{
DS18B20_GPIO_PORT &= ~(1<<DS18B20_GPIO_PIN);
DS18B20_GPIO_PORT |= ((dat>>i)&0x01)<<DS18B20_GPIO_PIN;
delay_us(5);
DS18B20_GPIO_PORT |= (1<<DS18B20_GPIO_PIN);
delay_us(5);
}
}
u8 DS18B20_Read_Byte()
{
u8 i, dat = 0;
for(i=0; i<8; i++)
{
DS18B20_GPIO_PORT &= ~(1<<DS18B20_GPIO_PIN);
delay_us(5);
dat |= ((DS18B20_GPIO_PORT>>DS18B20_GPIO_PIN)&0x01)<<i;
DS18B20_GPIO_PORT |= (1<<DS18B20_GPIO_PIN);
delay_us(5);
}
return dat;
}
void DS18B20_Read_Temperature(float *temp)
{
u8 buf[2];
u16 temp_integer, temp_decimal;
DS18B20_Reset();
DS18B20_Write_Byte(0xCC);
DS18B20_Write_Byte(0x44);
delay_us(750);
DS18B20_Reset();
DS18B20_Write_Byte(0xCC);
DS18B20_Write_Byte(0xBE);
buf[0] = DS18B20_Read_Byte();
buf[1] = DS18B20_Read_Byte();
temp_integer = buf[1] << 8 | buf[0];
*temp = temp_integer * 0.0625;
}
void LCD1602_Write_Command(u8 command)
{
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_EN = 1;
LCD1602_DB = command;
delay_us(5);
LCD1602_EN = 0;
}
void LCD1602_Write_Data(u8 data)
{
LCD1602_RS = 1;
LCD1602_RW = 0;
LCD1602_EN = 1;
LCD1602_DB = data;
delay_us(5);
LCD1602_EN = 0;
}
void LCD1602_Write_String(u8 x, u8 y, u8 *str)
{
u8 i;
if(x<16)
{
if(y==0)
{
LCD1602_Write_Command(0x80+x);
}
else if(y==1)
{
LCD1602_Write_Command(0xC0+x);
}
for(i=0; str[i]!='\0'; i++)
{
LCD1602_Write_Data(str[i]);
}
}
}
void LCD1602_Init()
{
LCD1602_Write_Command(0x38);
delay_us(50);
LCD1602_Write_Command(0x38);
delay_us(50);
LCD1602_Write_Command(0x38);
delay_us(50);
LCD1602_Write_Command(0x0C);
delay_us(50);
LCD1602_Write_Command(0x01);
delay_ms(5);
LCD1602_Write_Command(0x06);
delay_us(50);
}
```
3. 主程序:
```c
float temp;
char temp_str[16];
DS18B20_Read_Temperature(&temp);
sprintf(temp_str, "Temperature: %.1fC", temp);
LCD1602_Write_String(0, 0, temp_str);
```
以上代码仅供参考,具体实现需要根据您的具体硬件平台和接口方式进行调整。另外,需要注意的是,51单片机的LCD1602液晶屏接口方式一般为并行口,需要进行相应的调整。
相关推荐
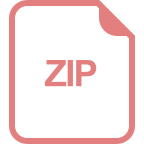
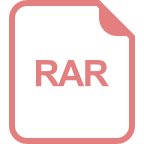














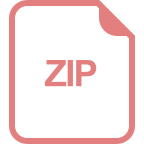