Keil中18B20的数值在LCD18B20上显示
时间: 2023-11-06 14:14:01 浏览: 82
如果您想在Keil中读取18B20温度传感器的值,并将其显示在LCD上,可以按照以下步骤操作:
1. 首先,您需要将18B20传感器连接到您的开发板上,并确保它可以正常工作。您可以通过编写一个简单的程序来读取传感器的值,并在串口终端中打印出来,以确保它可以正常工作。
2. 接下来,您需要编写一个LCD显示程序,该程序可以将数字值转换为字符串,并将其显示在LCD上。您可以使用LCD库来简化这个过程。
3. 最后,您需要将两个程序结合起来,以便在读取传感器值后,将其转换为字符串,并将其显示在LCD上。您可以使用sprintf函数将数字值转换为字符串,并将其传递给LCD显示函数来显示。
下面是一个示例程序,它可以读取18B20传感器的值,并将其显示在LCD上:
```
#include <reg51.h>
#include <stdio.h>
#include <string.h>
#include "lcd.h"
sbit DQ = P1^0; // 18B20数据线连接到P1.0口
void delay_us(unsigned int us)
{
while(us--)
{
_nop_();
_nop_();
_nop_();
_nop_();
}
}
unsigned char read_byte(void)
{
unsigned char i, dat = 0;
for(i=0; i<8; i++)
{
dat >>= 1;
DQ = 0;
delay_us(2);
DQ = 1;
delay_us(1);
if(DQ)
dat |= 0x80;
delay_us(60);
}
return dat;
}
void write_byte(unsigned char dat)
{
unsigned char i;
for(i=0; i<8; i++)
{
DQ = 0;
delay_us(2);
DQ = dat & 0x01;
delay_us(60);
DQ = 1;
dat >>= 1;
}
}
void init_18b20(void)
{
unsigned char flag;
DQ = 1;
delay_us(2);
DQ = 0;
delay_us(500);
DQ = 1;
delay_us(60);
flag = DQ;
delay_us(500);
}
unsigned int read_18b20(void)
{
unsigned char TL, TH;
unsigned int temp;
init_18b20();
write_byte(0xCC); // 跳过ROM操作
write_byte(0x44); // 启动温度转换
delay_us(100);
init_18b20();
write_byte(0xCC); // 跳过ROM操作
write_byte(0xBE); // 读取温度值
TL = read_byte(); // 读取温度低8位
TH = read_byte(); // 读取温度高8位
temp = TH;
temp <<= 8;
temp |= TL;
return temp;
}
void main()
{
unsigned int temp;
char str[16];
lcd_init(); // 初始化LCD
while(1)
{
temp = read_18b20(); // 读取18B20温度值
sprintf(str, "Temp: %d.%dC", temp/16, temp%16*10/16); // 将温度值转换为字符串
lcd_clear(); // 清屏
lcd_puts(str); // 显示温度值
delay_ms(1000); // 延时1秒
}
}
```
注意:这只是一个简单的示例程序,您需要根据您的具体硬件和软件环境进行修改。
阅读全文
相关推荐

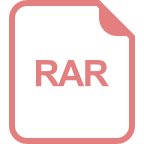

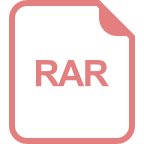











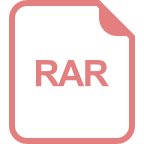