在Keil中读取18B20的数据,在LCD1602上显示
时间: 2024-02-13 11:02:33 浏览: 83
好的,我可以为您提供一些参考代码,但需要先确认您使用的具体硬件平台和接口方式。
假设您使用的是STM32单片机,18B20温度传感器采用单总线协议连接,LCD1602液晶屏采用I2C总线协议连接,那么以下是基本的代码实现流程:
1. 引入相关库文件:
```c
#include "stm32f10x.h"
#include "delay.h" // 延时函数库
#include "i2c.h" // I2C总线协议库
#include "lcd1602.h" // LCD1602液晶屏库
```
2. 定义相关宏定义:
```c
#define DS18B20_GPIO_PORT GPIOB
#define DS18B20_GPIO_PIN GPIO_Pin_8
```
3. 初始化GPIO口:
```c
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DS18B20_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
```
4. 初始化I2C总线和LCD1602液晶屏:
```c
I2C_Configuration();
LCD1602_Init();
```
5. 读取18B20温度传感器数据:
```c
void DS18B20_Read_Temperature(float *temp)
{
u8 buf[2];
u16 temp_integer, temp_decimal;
DS18B20_Reset();
DS18B20_Write_Byte(0xCC);
DS18B20_Write_Byte(0x44);
delay_us(750000);
DS18B20_Reset();
DS18B20_Write_Byte(0xCC);
DS18B20_Write_Byte(0xBE);
buf[0] = DS18B20_Read_Byte();
buf[1] = DS18B20_Read_Byte();
temp_integer = buf[1] << 8 | buf[0];
*temp = temp_integer * 0.0625;
}
```
6. 显示温度数据到LCD1602液晶屏:
```c
float temp;
char temp_str[16];
DS18B20_Read_Temperature(&temp);
sprintf(temp_str, "Temperature: %.1fC", temp);
LCD1602_Write_String(0, 0, temp_str);
```
以上代码仅供参考,具体实现需要根据您的具体硬件平台和接口方式进行调整。
阅读全文
相关推荐










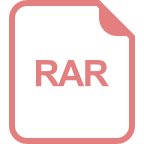




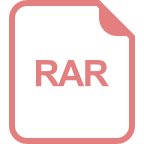