黄金矿工 C++ 多文件 easyx
时间: 2023-08-05 11:07:31 浏览: 35
好的,以下是黄金矿工的 C++ 多文件版 easyx 实现,文件分别为 main.cpp、game.h、game.cpp、object.h、object.cpp、resource.h、resource.cpp。代码注释较详细,希望能帮到你。
main.cpp:
```cpp
#include "game.h"
int main()
{
Game game;
game.run();
return 0;
}
```
game.h:
```cpp
#ifndef GAME_H
#define GAME_H
#include <vector>
#include "object.h"
#include "resource.h"
class Game
{
public:
Game();
~Game();
void run();
private:
void init(); // 初始化游戏
void update(); // 更新游戏
void render(); // 渲染游戏
void handleInput(); // 处理输入
void spawnObject(); // 生成物体
void checkCollision(); // 检测碰撞
void gameOver(); // 游戏结束
bool isCollided(Object& a, Object& b); // 判断两个物体是否碰撞
static const int WIDTH = 800; // 窗口宽度
static const int HEIGHT = 600; // 窗口高度
Resource resource; // 资源管理器
std::vector<Object*> objects; // 物体数组
Object* player; // 玩家
bool isRunning; // 是否正在运行
};
#endif // GAME_H
```
game.cpp:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include "game.h"
// 构造函数
Game::Game()
{
init();
}
// 析构函数
Game::~Game()
{
for (Object* object : objects)
{
delete object;
}
objects.clear();
delete player;
}
// 初始化游戏
void Game::init()
{
// 初始化随机数种子
srand((unsigned)time(NULL));
// 初始化资源
resource.load();
// 创建玩家
player = new Object(WIDTH / 2, HEIGHT - 80, Object::PLAYER, &resource);
// 设置游戏状态
isRunning = true;
// 生成初始物体
for (int i = 0; i < 5; i++)
{
spawnObject();
}
}
// 运行游戏
void Game::run()
{
while (isRunning)
{
// 处理输入
handleInput();
// 更新游戏
update();
// 渲染游戏
render();
// 等待一段时间
Sleep(10);
}
}
// 更新游戏
void Game::update()
{
// 更新玩家
player->update();
// 更新物体
for (Object* object : objects)
{
object->update();
}
// 检测碰撞
checkCollision();
// 生成新物体
if (rand() % 100 == 0)
{
spawnObject();
}
// 游戏结束
if (player->getHealth() <= 0)
{
gameOver();
}
}
// 渲染游戏
void Game::render()
{
// 清空屏幕
cleardevice();
// 绘制玩家
player->render();
// 绘制物体
for (Object* object : objects)
{
object->render();
}
// 绘制生命值
settextcolor(WHITE);
settextstyle(20, 0, _T("Consolas"));
TCHAR healthText[10];
_stprintf_s(healthText, _T("Health: %d"), player->getHealth());
outtextxy(10, 10, healthText);
// 更新屏幕
flushbatch();
}
// 处理输入
void Game::handleInput()
{
// 检测按键
if (GetAsyncKeyState(VK_LEFT) & 0x8000)
{
player->setDir(Object::LEFT);
}
else if (GetAsyncKeyState(VK_RIGHT) & 0x8000)
{
player->setDir(Object::RIGHT);
}
else
{
player->setDir(Object::NONE);
}
}
// 生成物体
void Game::spawnObject()
{
int type = rand() % Object::COUNT;
int x = rand() % (WIDTH - 40) + 20;
int y = rand() % 200 - 300;
objects.push_back(new Object(x, y, (Object::Type)type, &resource));
}
// 检测碰撞
void Game::checkCollision()
{
// 判断玩家与物体是否碰撞
for (auto it = objects.begin(); it != objects.end();)
{
Object* object = *it;
if (isCollided(*player, *object))
{
player->setHealth(player->getHealth() + object->getHealth());
player->setScore(player->getScore() + object->getScore());
delete object;
it = objects.erase(it);
}
else
{
it++;
}
}
}
// 判断两个物体是否碰撞
bool Game::isCollided(Object& a, Object& b)
{
int ax = a.getX(), ay = a.getY(), aw = a.getWidth(), ah = a.getHeight();
int bx = b.getX(), by = b.getY(), bw = b.getWidth(), bh = b.getHeight();
if (ax + aw < bx || ax > bx + bw || ay + ah < by || ay > by + bh)
{
return false;
}
return true;
}
// 游戏结束
void Game::gameOver()
{
isRunning = false;
cleardevice();
settextcolor(WHITE);
settextstyle(50, 0, _T("Consolas"));
outtextxy(200, 250, _T("Game Over"));
flushbatch();
_getch();
}
```
object.h:
```cpp
#ifndef OBJECT_H
#define OBJECT_H
#include "resource.h"
class Object
{
public:
enum Type { GOLD, ROCK, DIAMOND, COUNT };
enum Dir { LEFT, RIGHT, NONE };
Object(int x, int y, Type type, Resource* resource);
~Object();
void update();
void render();
void setDir(Dir dir) { this->dir = dir; }
int getX() const { return x; }
int getY() const { return y; }
int getWidth() const { return width; }
int getHeight() const { return height; }
int getHealth() const { return health; }
int getScore() const { return score; }
private:
int x, y;
int width, height;
int health, score;
Type type;
Dir dir;
Resource* resource;
};
#endif // OBJECT_H
```
object.cpp:
```cpp
#include <graphics.h>
#include "object.h"
// 构造函数
Object::Object(int x, int y, Type type, Resource* resource)
{
this->x = x;
this->y = y;
this->type = type;
this->resource = resource;
// 设置属性
switch (type)
{
case GOLD:
width = 40;
height = 40;
health = 10;
score = 10;
break;
case ROCK:
width = 60;
height = 60;
health = -20;
score = 0;
break;
case DIAMOND:
width = 30;
height = 30;
health = 20;
score = 20;
break;
default:
break;
}
// 设置方向
dir = NONE;
}
// 析构函数
Object::~Object()
{
}
// 更新物体
void Object::update()
{
// 根据方向移动
switch (dir)
{
case LEFT:
x -= 5;
break;
case RIGHT:
x += 5;
break;
default:
break;
}
// 如果超出屏幕,删除物体
if (y > 600 || y < -100 || x > 800 || x < -100)
{
delete this;
}
}
// 绘制物体
void Object::render()
{
switch (type)
{
case GOLD:
resource->draw(x, y, Resource::GOLD);
break;
case ROCK:
resource->draw(x, y, Resource::ROCK);
break;
case DIAMOND:
resource->draw(x, y, Resource::DIAMOND);
break;
default:
break;
}
}
```
resource.h:
```cpp
#ifndef RESOURCE_H
#define RESOURCE_H
#include <graphics.h>
#include <map>
class Resource
{
public:
enum Type { GOLD, ROCK, DIAMOND, COUNT };
Resource();
~Resource();
void load();
void draw(int x, int y, Type type);
private:
std::map<Type, IMAGE*> images;
};
#endif // RESOURCE_H
```
resource.cpp:
```cpp
#include "resource.h"
// 构造函数
Resource::Resource()
{
}
// 析构函数
Resource::~Resource()
{
for (auto it = images.begin(); it != images.end(); it++)
{
delimage(it->second);
}
images.clear();
}
// 加载资源
void Resource::load()
{
images[GOLD] = newimage();
getimage(images[GOLD], _T("res\\gold.png"));
images[ROCK] = newimage();
getimage(images[ROCK], _T("res\\rock.png"));
images[DIAMOND] = newimage();
getimage(images[DIAMOND], _T("res\\diamond.png"));
}
// 绘制图片
void Resource::draw(int x, int y, Type type)
{
putimage(x, y, images[type]);
}
```
相关推荐
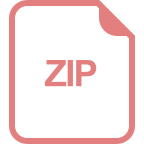
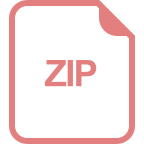





