用C语言求解一般集合的并集问题
时间: 2023-05-30 08:01:20 浏览: 141
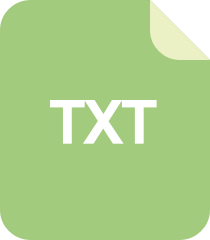
C语言 并集

以下是使用C语言实现一般集合的并集的示例代码:
```c
#include <stdio.h>
#define MAX_SIZE 10 // 集合的最大大小
int main()
{
int set1[MAX_SIZE], set2[MAX_SIZE], unionSet[MAX_SIZE*2]; // 定义两个集合和结果集合
int size1, size2, sizeUnion = 0; // 分别表示两个集合的大小和结果集合的大小
int i, j, flag;
// 输入第一个集合
printf("Enter the size of set1: ");
scanf("%d", &size1);
printf("Enter the elements of set1: ");
for (i = 0; i < size1; i++)
{
scanf("%d", &set1[i]);
}
// 输入第二个集合
printf("Enter the size of set2: ");
scanf("%d", &size2);
printf("Enter the elements of set2: ");
for (i = 0; i < size2; i++)
{
scanf("%d", &set2[i]);
}
// 计算并集
for (i = 0; i < size1; i++)
{
unionSet[sizeUnion++] = set1[i]; // 将第一个集合的元素加入结果集合
}
for (i = 0; i < size2; i++)
{
flag = 1; // 标记是否已存在于结果集合中
for (j = 0; j < sizeUnion; j++)
{
if (set2[i] == unionSet[j])
{
flag = 0;
break;
}
}
if (flag) // 如果不存在,则加入结果集合
{
unionSet[sizeUnion++] = set2[i];
}
}
// 输出结果集合
printf("The union of set1 and set2 is: ");
for (i = 0; i < sizeUnion; i++)
{
printf("%d ", unionSet[i]);
}
printf("\n");
return 0;
}
```
该程序首先输入两个集合,然后计算它们的并集,并将结果输出。其中,使用了一个额外的数组来存储结果集合,使用了两个循环遍历集合并计算并集,其中第二个循环中使用了一个标记来判断一个元素是否已存在于结果集合中。
阅读全文
相关推荐
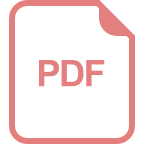


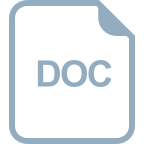


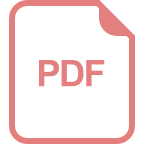






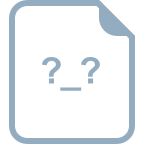
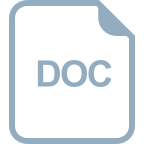
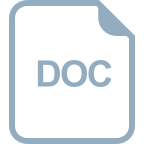