(1)设计一个基类 Course,包含 private 数据成员课程 ID、学分 credit;public 函数 get_credit 用于读取 credit 的值,两个纯虚函数:总分计算 totalS,课程绩点 计算 GPA (2)编写三个子类:高数 AdvanceMathematic、英语 English、C 语言 Cprogram 课程 课程 ID 学分 考试成绩 平时成绩 成绩比例 (考试:平时) 高数 1001 4 80 90 6:4 大学英语 1002 3 80 60 7:3 C 语言 1003 3 100 90 5:5 公有继承基类 Course。每个类都两个私有属性考试分数 exam,平时分数 dailywork。 (3)每门课程绩点的计算方法为:GPA=总分/10.0-5;平均绩点计算方法为: 平均绩点=Σ(学分*GPA)/Σ学分 (4)设计一个 main 函数,要求使用基类指针,并实现每门课程总分及平均绩点 的计算并输出
时间: 2024-03-10 16:49:33 浏览: 83
好的,我已经了解了你的问题。下面是一个基于你的要求编写的 C++ 代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 基类 Course
class Course {
protected:
int course_id; // 课程 ID
int credit; // 学分
public:
Course(int id, int c) : course_id(id), credit(c) {}
virtual ~Course() {}
int get_credit() { return credit; } // 读取学分
virtual int totalS() = 0; // 纯虚函数,计算总分
virtual double GPA() = 0; // 纯虚函数,计算课程绩点
};
// 子类 AdvanceMathematic
class AdvanceMathematic : public Course {
private:
int exam; // 考试成绩
int dailywork; // 平时成绩
double ratio; // 成绩比例
public:
AdvanceMathematic(int id, int c, int e, int d, double r)
: Course(id, c), exam(e), dailywork(d), ratio(r) {}
virtual int totalS() { return exam * ratio + dailywork * (1 - ratio); }
virtual double GPA() { return totalS() / 10.0 - 5; }
};
// 子类 English
class English : public Course {
private:
int exam; // 考试成绩
int dailywork; // 平时成绩
double ratio; // 成绩比例
public:
English(int id, int c, int e, int d, double r)
: Course(id, c), exam(e), dailywork(d), ratio(r) {}
virtual int totalS() { return exam * ratio + dailywork * (1 - ratio); }
virtual double GPA() { return totalS() / 10.0 - 5; }
};
// 子类 Cprogram
class Cprogram : public Course {
private:
int exam; // 考试成绩
int dailywork; // 平时成绩
double ratio; // 成绩比例
public:
Cprogram(int id, int c, int e, int d, double r)
: Course(id, c), exam(e), dailywork(d), ratio(r) {}
virtual int totalS() { return exam * ratio + dailywork * (1 - ratio); }
virtual double GPA() { return totalS() / 10.0 - 5; }
};
int main() {
// 创建三个课程的对象
AdvanceMathematic math(1001, 4, 80, 90, 0.6);
English eng(1002, 3, 80, 60, 0.7);
Cprogram c(1003, 3, 100, 90, 0.5);
// 使用基类指针,将三个对象存入 vector 中
vector<Course*> courses;
courses.push_back(&math);
courses.push_back(&eng);
courses.push_back(&c);
int total_score = 0; // 所有课程总分
double total_GPA = 0; // 所有课程绩点总和
int total_credit = 0; // 所有课程学分总和
// 遍历 vector 中的每个课程
for (auto c : courses) {
total_score += c->totalS(); // 计算总分
total_GPA += c->GPA() * c->get_credit(); // 计算绩点总和
total_credit += c->get_credit(); // 计算学分总和
}
double avg_GPA = total_GPA / total_credit; // 计算平均绩点
// 输出结果
cout << "所有课程总分:" << total_score << endl;
cout << "所有课程平均绩点:" << avg_GPA << endl;
return 0;
}
```
这段代码定义了一个基类 Course 和三个子类 AdvanceMathematic、English、Cprogram,每个子类都公开继承基类 Course,并重写了基类中的纯虚函数 totalS 和 GPA,实现了每门课程总分和绩点的计算。在 main 函数中,创建了三个课程对象,并将它们存储在一个 vector 中。然后,遍历 vector 中的每个课程,计算所有课程的总分和平均绩点,并输出结果。
阅读全文
相关推荐
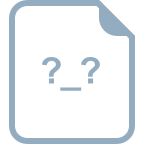
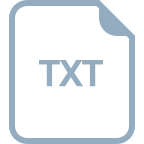
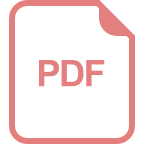
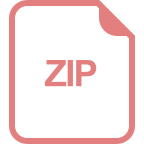
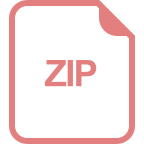
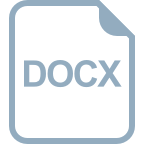
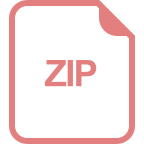
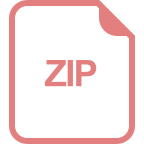
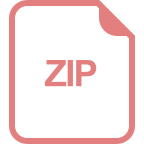
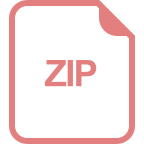
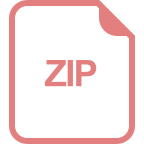
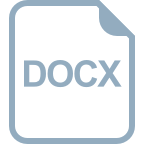