1. 某学校教学系统中可以对学生的分数及绩点进行计算,张三同学的成绩如下表 课程 课程ID 学分 考试成绩 平时成绩 成绩比例 (考试:平时) 高数 1001 4 80 90 6:4 大学英语 1002 3 80 60 7:3 C语言 1003 3 100 90 5:5 (1)设计一个基类Course,包含private数据成员课程ID、学分credit;public函数get_credit用于读取credit的值,两个纯虚函数:总分计算totalS,课程绩点计算GPA (2)编写三个子类:高数AdvanceMathematic、英语English、C语言Cprogram公有继承基类Course。每个类都两个私有属性考试分数exam,平时分数dailywork。 (3)每门课程绩点的计算方法为:GPA=总分/10.0-5;平均绩点计算方法为: 平均绩点=Σ(学分*GPA)/Σ学分 (4)设计一个main函数,要求使用基类指针,并实现每门课程总分及平均绩点的计算并输出。 (20分)
时间: 2023-07-16 19:15:34 浏览: 51
```cpp
#include <iostream>
#include <string>
using namespace std;
class Course {
protected:
string courseID;
int credit;
public:
Course(string id, int c) : courseID(id), credit(c) {}
virtual double totalS() = 0;
virtual double GPA() = 0;
int get_credit() { return credit; }
};
class AdvanceMathematic : public Course {
private:
int exam;
int dailywork;
public:
AdvanceMathematic(string id, int c, int e, int d) : Course(id, c), exam(e), dailywork(d) {}
double totalS() {
return exam * 0.6 + dailywork * 0.4;
}
double GPA() {
return totalS() / 10.0 - 5;
}
};
class English : public Course {
private:
int exam;
int dailywork;
public:
English(string id, int c, int e, int d) : Course(id, c), exam(e), dailywork(d) {}
double totalS() {
return exam * 0.7 + dailywork * 0.3;
}
double GPA() {
return totalS() / 10.0 - 5;
}
};
class Cprogram : public Course {
private:
int exam;
int dailywork;
public:
Cprogram(string id, int c, int e, int d) : Course(id, c), exam(e), dailywork(d) {}
double totalS() {
return exam * 0.5 + dailywork * 0.5;
}
double GPA() {
return totalS() / 10.0 - 5;
}
};
int main() {
Course* courses[3];
courses[0] = new AdvanceMathematic("1001", 4, 80, 90);
courses[1] = new English("1002", 3, 80, 60);
courses[2] = new Cprogram("1003", 3, 100, 90);
double total_GPA = 0;
int total_credit = 0;
for (int i = 0; i < 3; i++) {
cout << "课程ID: " << courses[i]->courseID << endl;
cout << "学分: " << courses[i]->get_credit() << endl;
cout << "总分: " << courses[i]->totalS() << endl;
cout << "绩点: " << courses[i]->GPA() << endl << endl;
total_GPA += courses[i]->GPA() * courses[i]->get_credit();
total_credit += courses[i]->get_credit();
}
double avg_GPA = total_GPA / total_credit;
cout << "平均绩点: " << avg_GPA << endl;
return 0;
}
```
相关推荐
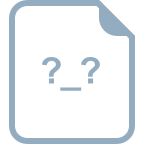
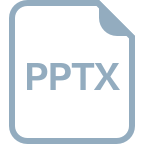
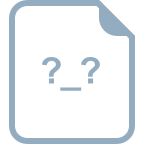















