首页
使用python给我写一个读取qq邮箱邮件的代码
使用python给我写一个读取qq邮箱邮件的代码
时间: 2023-03-14 09:08:02
浏览: 134
python-qq邮件发送小代码
立即下载
python-qq邮件发送小代码
我可以提供一些建议:1.使用Python标准库中的IMAPlib模块读取QQ邮箱。 2.使用Python SMTPlib模块发送邮件。 3.使用Python email模块来解析邮件内容和头部数据。 4.使用Python库poplib来读取POP3邮箱。
阅读全文
相关推荐
python实现QQ邮箱发送邮件
本文实例为大家分享了python实现QQ邮箱发送邮件的具体代码,供大家参考,具体内容如下 1、代码: #!/usr/bin/python # -*- coding: utf-8 -*- import smtplib from email.mime.text import MIMEText from email.header import Header mail_host=smtp.qq.com#设置的邮件服务器host必须是发送邮箱的服务器,与接收邮箱无关。 mail_user=**************#qq邮箱登陆名 mail_pass=*****************
Python利用QQ邮箱发送邮件的实现方法(分享)
下面小编就为大家带来一篇Python利用QQ邮箱发送邮件的实现方法(分享)。小编觉得挺不错的,现在就分享给大家,也给大家做个参考。一起跟随小编过来看看吧
python读取qq邮箱邮件
在Python中,我们可以使用第三方库如imaplib和email模块来读取QQ邮箱的邮件。以下是简单的步骤: 1. 首先,你需要安装这两个库,如果还没有安装,可以使用pip进行安装: pip install imaplib email ...
python读取qq邮箱邮件归档文件夹
您可以使用Python的imaplib模块来读取QQ邮箱邮件归档文件夹。以下是一个简单的示例代码: python import imaplib # 邮箱登录信息 email = 'your_email@qq.com' password = 'your_password' # 连接到QQ邮箱的...
python发送邮箱代码 qq邮箱 微信企业邮箱 163邮箱
python发送邮箱代码 qq邮箱 微信企业邮箱 163邮箱 适用人群python发送邮箱 ,如有问题请私信我。+适用人群python+其他方面不能使用请联系我
python3通过qq邮箱发送邮件以及附件
这篇教程主要讲解如何使用Python 3通过QQ邮箱发送带有附件的邮件。QQ邮箱提供了SMTP(Simple Mail Transfer Protocol)服务,允许开发者通过编程方式发送邮件。下面我们将深入探讨这个过程。 首先,确保你已经开启...
使用python群发邮件
port = 587 # QQ邮箱和163邮箱通常使用此端口 sender = "your_email@qq.com" # 发送者的邮箱地址 password = "your_password" # 发送者的邮箱授权码 receivers = ["receiver1@example.com", "receiver2@example....
Python自动化邮箱处理教程:发送、读取、定时和删除邮件
- **发送带附件的电子邮件**:创建一个带有MIMEBase附件的MIMEMultipart对象,将附件数据以二进制形式添加。 - **以邮件的内容发送,而不是作为附件形式发送**:使用MIMEApplication来发送非文本内容(如...
Python QQ邮箱自动化发邮件教程:带文本与附件示例
在Python编程中,利用QQ邮箱实现自动发送邮件是一个常见的任务,特别是在需要自动化处理邮件流程的场景下。本文将详细介绍如何使用Python的smtplib和email库来实现这一功能,这两个库是Python标准库中的邮件处理...
python代码生成微信机器人获取QQ邮箱邮件
以下是一个基本的 Python 代码示例,可用于生成微信机器人,以获取 QQ 邮箱邮件: python import itchat import poplib from email.parser import Parser # 登录微信账号 itchat.auto_login(hotReload=True) #...
python代码生成微信机器人实时获取QQ邮箱邮件标题和正文
以下是一个可以实时获取 QQ 邮箱邮件标题和正文的 Python 代码示例,用于生成微信机器人: python import itchat import poplib from email.parser import Parser # 登录微信账号 itchat.auto_login(hotReload=...
我想自动发送邮件,我是QQ邮箱账号,请给我一个python代码与伪代码流程,并告诉我每个指令的含义、参数和可实现的功能,并给这些指令和参数举一个输入值和输出值的例子
好的,以下是一份基于 Python 的发送邮件的代码和伪代码流程: ## 代码 python import smtplib from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart from email.mime....
我想利用python 读取企业邮箱的收件箱的邮件
要读取企业邮箱的收件箱的邮件,需要使用IMAP协议,通过Python中的IMAP库实现。以下是一个基本的示例代码: python import imaplib import email from email.header import decode_header # 邮箱账号信息 ...
如何高效地使用Python脚本自动化管理QQ邮箱中的邮件,涵盖发送、定时任务、读取、筛选、删除等操作?
例如,以下是一个Python脚本示例,展示了如何使用smtplib和imaplib库来自动化处理QQ邮箱中的邮件: 参考资源链接:[Python自动化邮箱处理教程:发送、读取、定时和删除邮件]...
请描述如何利用Python脚本自动化处理QQ邮箱中的邮件,包括定时发送、读取、筛选和删除邮件。
邮件的读取需要使用imaplib模块连接到QQ邮箱的IMAP服务器。通过相应的命令,我们可以读取全部邮件内容、未读邮件、标记邮件等。筛选邮件时,可以使用IMAP协议的搜索功能,根据发件人、收件人、主题等信息进行筛选...
如何编写Python脚本以自动化QQ邮箱中的邮件处理任务,包括定时发送、读取、筛选和删除邮件?
为了帮助你掌握这一技能,我推荐查阅《Python自动化邮箱处理教程:发送、读取、定时和删除邮件》。 参考资源链接:[Python自动化邮箱处理教程:发送、读取、定时和删除邮件]...
如何使用Python结合QQ邮箱SMTP服务实现带有附件的邮件自动发送?请提供详细的代码示例。
实现使用Python结合QQ邮箱SMTP服务发送带有附件的邮件,首先需要确保已经开启了QQ邮箱的SMTP服务,并获取授权码。接着利用Python标准库中的smtplib和email模块来构建和发送邮件。以下是详细步骤和代码示例: ...
python qq邮箱发文件
下面是一个简单的示例代码,演示如何使用Python发送文件到QQ邮箱: python import smtplib from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart from email.mime.application...
邮箱电子发票下载脚本 使用python3开发,通过pop3或者imap来获取指定邮箱的指定日期的邮件列表,然后根据发件人和标题判断邮件是否是京东或者sarm的电子发票,如果是的话,读取邮件内容,并把邮件里面的发票下载下来【发票是个链接】,存到程序当前fp目录。 要求:程序要能支持各类常见邮箱,如qq,163,hotmail等等。 程序要能设置指定的日期时间段,如20230401-20230531。程序源码交付,要有注释,要有详细的使用说明。
根据您的要求,我可以提供一个Python脚本来实现您的需求。以下是一个示例脚本,通过使用IMAP协议从指定邮箱中获取指定日期范围内的电子发票并下载到本地。 请注意,由于涉及到不同类型的邮箱,您可能需要安装相应的...
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
海量
VIP免费资源
千本
正版电子书
商城
会员专享价
千门
课程&专栏
全年可省5,000元
立即开通
全年可省5,000元
立即开通
最新推荐
Python3使用SMTP发送带附件邮件
在上面的代码中,我们首先创建了一个MIMEMultipart对象,然后添加了邮件的发送者、接收者和主题。接着,我们创建了MIMEText对象来添加邮件正文。对于每个附件,我们读取文件内容,创建MIMEText对象,并设置其Content...
Python之csv文件从MySQL数据库导入导出的方法
2. 然后,使用`cursor()`方法创建一个游标对象,用于执行SQL查询。 3. 定义一个`query_all()`函数,接收游标和SQL查询语句,执行查询并返回所有结果。 4. 使用`read_mysql_to_csv()`函数,创建一个CSV写入器,并...
数据库基础测验20241113.doc
数据库基础测验20241113.doc
微信小程序下拉选择组件
微信小程序下拉选择组件
黑板风格计算机毕业答辩PPT模板下载
资源摘要信息:"创意经典黑板风格毕业答辩论文课题报告动态ppt模板" 在当前数字化教学与展示需求日益增长的背景下,PPT模板成为了表达和呈现学术成果及教学内容的重要工具。特别针对计算机专业的学生而言,毕业设计的答辩PPT不仅仅是一个展示的平台,更是其设计能力、逻辑思维和审美观的综合体现。因此,一个恰当且创意十足的PPT模板显得尤为重要。 本资源名为“创意经典黑板风格毕业答辩论文课题报告动态ppt模板”,这表明该模板具有以下特点: 1. **创意设计**:模板采用了“黑板风格”的设计元素,这种风格通常模拟传统的黑板书写效果,能够营造一种亲近、随性的学术氛围。该风格的模板能够帮助展示者更容易地吸引观众的注意力,并引发共鸣。 2. **适应性强**:标题表明这是一个毕业答辩用的模板,它适用于计算机专业及其他相关专业的学生用于毕业设计课题的汇报。模板中设计的版式和内容布局应该是灵活多变的,以适应不同课题的展示需求。 3. **动态效果**:动态效果能够使演示内容更富吸引力,模板可能包含了多种动态过渡效果、动画效果等,使得展示过程生动且充满趣味性,有助于突出重点并维持观众的兴趣。 4. **专业性质**:由于是毕业设计用的模板,因此该模板在设计时应充分考虑了计算机专业的特点,可能包括相关的图表、代码展示、流程图、数据可视化等元素,以帮助学生更好地展示其研究成果和技术细节。 5. **易于编辑**:一个良好的模板应具备易于编辑的特性,这样使用者才能根据自己的需要进行调整,比如替换文本、修改颜色主题、更改图片和图表等,以确保最终展示的个性和专业性。 结合以上特点,模板的使用场景可以包括但不限于以下几种: - 计算机科学与技术专业的学生毕业设计汇报。 - 计算机工程与应用专业的学生论文展示。 - 软件工程或信息技术专业的学生课题研究成果展示。 - 任何需要进行学术成果汇报的场合,比如研讨会议、学术交流会等。 对于计算机专业的学生来说,毕业设计不仅仅是完成一个课题,更重要的是通过这个过程学会如何系统地整理和表述自己的思想。因此,一份好的PPT模板能够帮助他们更好地完成这个任务,同时也能够展现出他们的专业素养和对细节的关注。 此外,考虑到模板是一个压缩文件包(.zip格式),用户在使用前需要解压缩,解压缩后得到的文件为“创意经典黑板风格毕业答辩论文课题报告动态ppt模板.pptx”,这是一个可以直接在PowerPoint软件中打开和编辑的演示文稿文件。用户可以根据自己的具体需要,在模板的基础上进行修改和补充,以制作出一个具有个性化特色的毕业设计答辩PPT。
管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
提升点阵式液晶显示屏效率技术
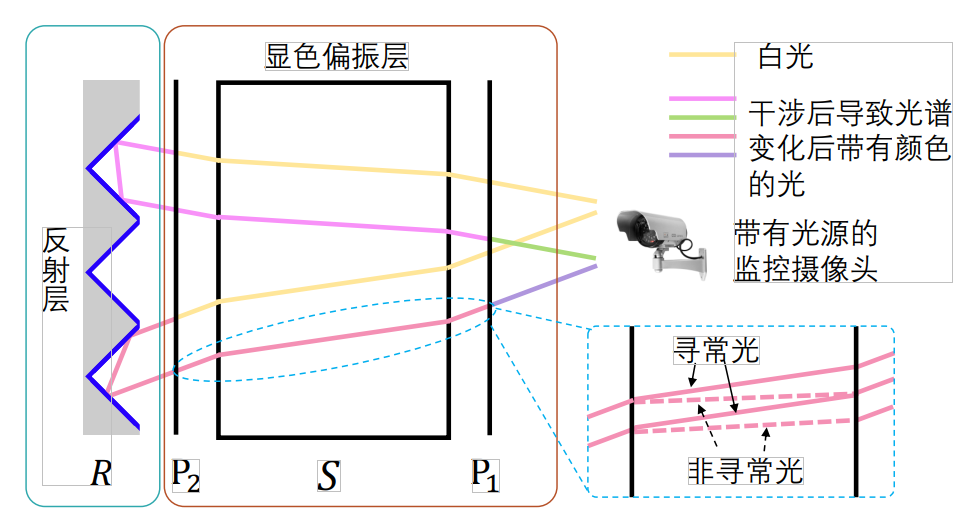 # 1. 点阵式液晶显示屏基础与效率挑战 在现代信息技术的浪潮中,点阵式液晶显示屏作为核心显示技术之一,已被广泛应用于从智能手机到工业控制等多个领域。本章节将介绍点阵式液晶显示屏的基础知识,并探讨其在提升显示效率过程中面临的挑战。 ## 1.1 点阵式显
在SoC芯片的射频测试中,ATE设备通常如何执行系统级测试以保证芯片量产的质量和性能一致?
SoC芯片的射频测试是确保无线通信设备性能的关键环节。为了在量产阶段保证芯片的质量和性能一致性,ATE(Automatic Test Equipment)设备通常会执行一系列系统级测试。这些测试不仅关注芯片的电气参数,还包含电磁兼容性和射频信号的完整性检验。在ATE测试中,会根据芯片设计的规格要求,编写定制化的测试脚本,这些脚本能够模拟真实的无线通信环境,检验芯片的射频部分是否能够准确处理信号。系统级测试涉及对芯片基带算法的验证,确保其能够有效执行无线信号的调制解调。测试过程中,ATE设备会自动采集数据并分析结果,对于不符合标准的芯片,系统能够自动标记或剔除,从而提高测试效率和减少故障率。为了
CodeSandbox实现ListView快速创建指南
资源摘要信息:"listview:用CodeSandbox创建" 知识点一:CodeSandbox介绍 CodeSandbox是一个在线代码编辑器,专门为网页应用和组件的快速开发而设计。它允许用户即时预览代码更改的效果,并支持多种前端开发技术栈,如React、Vue、Angular等。CodeSandbox的特点是易于使用,支持团队协作,以及能够直接在浏览器中编写代码,无需安装任何软件。因此,它非常适合初学者和快速原型开发。 知识点二:ListView组件 ListView是一种常用的用户界面组件,主要用于以列表形式展示一系列的信息项。在前端开发中,ListView经常用于展示从数据库或API获取的数据。其核心作用是提供清晰的、结构化的信息展示方式,以便用户可以方便地浏览和查找相关信息。 知识点三:用JavaScript创建ListView 在JavaScript中创建ListView通常涉及以下几个步骤: 1. 创建HTML的ul元素作为列表容器。 2. 使用JavaScript的DOM操作方法(如document.createElement, appendChild等)动态创建列表项(li元素)。 3. 将创建的列表项添加到ul容器中。 4. 通过CSS来设置列表和列表项的样式,使其符合设计要求。 5. (可选)为ListView添加交互功能,如点击事件处理,以实现更丰富的用户体验。 知识点四:在CodeSandbox中创建ListView 在CodeSandbox中创建ListView可以简化开发流程,因为它提供了一个在线环境来编写代码,并且支持实时预览。以下是使用CodeSandbox创建ListView的简要步骤: 1. 打开CodeSandbox官网,创建一个新的项目。 2. 在项目中创建或编辑HTML文件,添加用于展示ListView的ul元素。 3. 创建或编辑JavaScript文件,编写代码动态生成列表项,并将它们添加到ul容器中。 4. 使用CodeSandbox提供的实时预览功能,即时查看ListView的效果。 5. 若有需要,继续编辑或添加样式文件(通常是CSS),对ListView进行美化。 6. 利用CodeSandbox的版本控制功能,保存工作进度和团队协作。 知识点五:实践案例分析——listview-main 文件名"listview-main"暗示这可能是一个展示如何使用CodeSandbox创建基本ListView的项目。在这个项目中,开发者可能会包含以下内容: 1. 使用React框架创建ListView的示例代码,因为React是目前较为流行的前端库。 2. 展示如何将从API获取的数据渲染到ListView中,包括数据的获取、处理和展示。 3. 提供基本的样式设置,展示如何使用CSS来美化ListView。 4. 介绍如何在CodeSandbox中组织项目结构,例如如何分离组件、样式和脚本文件。 5. 包含一个简单的用户交互示例,例如点击列表项时弹出详细信息等。 总结来说,通过标题“listview:用CodeSandbox创建”,我们了解到本资源是一个关于如何利用CodeSandbox这个在线开发环境,来快速实现一个基于JavaScript的ListView组件的教程或示例项目。通过上述知识点的梳理,可以加深对如何创建ListView组件、CodeSandbox平台的使用方法以及如何在该平台中实现具体功能的理解。
"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依