C++编写迷宫游戏
时间: 2023-07-01 15:13:33 浏览: 66
好的,下面是一个简单的 C++ 编写的迷宫游戏示例,供参考:
```c++
#include <iostream>
#include <string>
using namespace std;
const int ROW = 5; // 迷宫行数
const int COL = 5; // 迷宫列数
// 迷宫地图
char map[ROW][COL] = {
{'#', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', '#'},
{'#', '#', '#', ' ', '#'},
{'#', ' ', ' ', ' ', '#'},
{'#', '#', '#', '#', '#'}
};
// 当前位置
int cur_row = 1;
int cur_col = 1;
// 打印迷宫地图
void print_map() {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
cout << map[i][j] << " ";
}
cout << endl;
}
}
// 移动
bool move(int row, int col) {
if (map[row][col] == ' ') { // 如果可以移动
map[cur_row][cur_col] = ' '; // 重置原位置
cur_row = row;
cur_col = col;
map[cur_row][cur_col] = 'o'; // 设置新位置
return true;
}
return false;
}
int main() {
map[cur_row][cur_col] = 'o'; // 设置起点
print_map();
while (true) {
string input;
cout << "请输入方向:";
cin >> input;
if (input == "w") { // 上
if (move(cur_row - 1, cur_col)) {
print_map();
}
} else if (input == "s") { // 下
if (move(cur_row + 1, cur_col)) {
print_map();
}
} else if (input == "a") { // 左
if (move(cur_row, cur_col - 1)) {
print_map();
}
} else if (input == "d") { // 右
if (move(cur_row, cur_col + 1)) {
print_map();
}
} else { // 输入错误
cout << "请输入正确的方向!" << endl;
}
if (cur_row == ROW - 2 && cur_col == COL - 2) { // 到达终点
cout << "恭喜您,成功到达终点!" << endl;
break;
}
}
return 0;
}
```
这个迷宫游戏是一个基于控制台的简单实现,玩家通过输入 w/s/a/d 来控制人物移动,最终到达终点。其中 # 表示障碍物,o 表示人物。你可以根据自己的需求对代码进行修改和扩展。
相关推荐
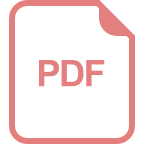
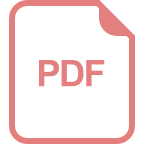
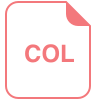
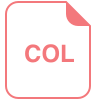
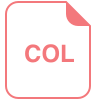











