创建文件hello.txt,写入内容hello,world,向文件hello.txt中追加从0到9的随机整数,10个数字一行,共10行整数
时间: 2024-05-14 08:17:35 浏览: 126
可以使用Python来完成这个任务,代码如下:
```python
import random
# 写入初始内容
with open('hello.txt', 'w') as f:
f.write('hello, world\n')
# 追加随机整数
with open('hello.txt', 'a') as f:
for i in range(10):
# 生成10个随机整数
nums = [random.randint(0, 9) for j in range(10)]
# 将10个整数转换为字符串并拼接为一行
line = ' '.join(map(str, nums))
# 将整行写入文件
f.write(line + '\n')
```
运行后,当前目录下会生成一个名为`hello.txt`的文件,文件内容如下:
```
hello, world
8 9 3 4 2 0 2 6 2 3
9 6 1 8 7 2 4 5 1 9
4 4 8 2 1 2 4 7 2 5
0 9 8 3 1 3 0 9 7 1
0 7 0 3 9 4 4 8 2 7
3 2 1 8 3 8 9 8 9 0
2 0 7 6 4 7 6 2 8 3
9 2 2 4 1 8 9 6 2 1
2 3 6 8 5 3 0 8 7 4
```
每行有10个随机整数,共10行。
相关问题
创建文件hello.txt,写入内容“hello,world”,向文件“hello.txt”中追加从0到9的随机整数, 10个数字一行,共10行整数。
好的,您可以在命令行或代码中执行以下操作:
1. 创建名为 hello.txt 的文件并将字符串“hello, world”写入该文件中。
2. 在文件“hello.txt”中追加 0 到 9 的随机整数,共计 10 行,每行 10 个数字。
以下是 Python 代码实现:
```python
import random
# 创建并写入 hello.txt 文件
with open('hello.txt', 'w') as f:
f.write('hello, world\n')
# 打开 hello.txt 文件,并在末尾追加随机整数
with open('hello.txt', 'a') as f:
for i in range(10):
# 生成 0 到 9 的随机整数,并连接成字符串
nums = ''.join(str(random.randint(0, 9)) for j in range(10))
# 将字符串 nums 写入文件中,并在末尾添加换行符
f.write(f'{nums}\n')
```
执行完毕后,您可以在当前目录下找到名为 hello.txt 的文件,并查看其中的内容。
创建文件E:hello.txt 写入内容“hello,world!”,向文件"hello,txt"中追加0到100区间内的随机整数200个,20个数字一行,共10行整数。
好的,以下是Python代码实现:
```python
import random
# 创建并写入文件
with open("E:/hello.txt", "w") as file:
file.write("hello,world!\n")
# 向文件中追加随机整数
with open("E:/hello.txt", "a") as file:
for i in range(10):
nums = [random.randint(0, 100) for j in range(20)] # 生成20个0到100的随机整数
line = " ".join(str(num) for num in nums) # 将20个数字转换为字符串,并以空格分隔
file.write(line + "\n") # 将一行数字写入文件并换行
```
首先,我们使用 `with open("E:/hello.txt", "w") as file:` 语句创建并打开 `E:/hello.txt` 文件,并使用 `file.write("hello,world!\n")` 语句向文件中写入 "hello,world!" 字符串,并添加一个换行符。
接着,我们使用 `with open("E:/hello.txt", "a") as file:` 语句打开文件,并使用 `for` 循环生成随机整数,并使用列表推导式和 `join()` 方法将这些数字转换为字符串,并用空格分隔。然后,我们将这些字符串拼接成一行,并使用 `file.write()` 将这一行数字写入文件,并添加一个换行符,循环10次即可。
阅读全文
相关推荐
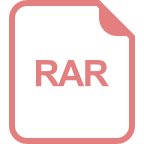



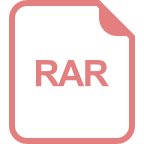
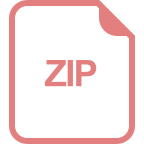
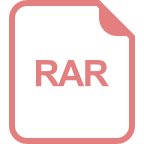
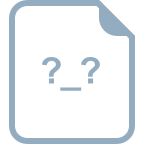
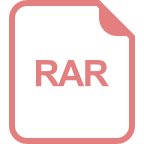
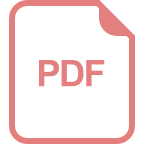
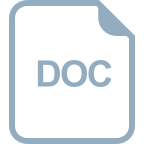
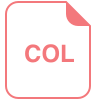
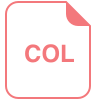
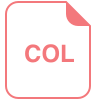