python 条件变量
时间: 2023-10-12 16:09:17 浏览: 77
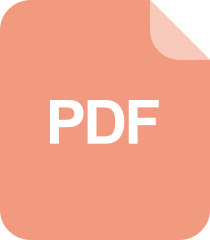
Python中的变量
条件变量是一种同步机制,用于线程之间的通信和协调。在 Python 中,条件变量可以用 threading 模块的 Condition 类来实现。
Condition 类有两个主要方法:wait() 和 notify()。wait() 方法用于等待条件变量的发生,如果条件不满足,线程就会阻塞在这里等待。notify() 方法用于通知等待条件变量的线程,让它们继续执行。
下面是一个简单的例子,演示了如何使用条件变量:
```python
import threading
class SharedCounter:
def __init__(self, value=0):
self.value = value
self.cv = threading.Condition()
def increment(self):
with self.cv:
self.value += 1
self.cv.notify_all()
def decrement(self):
with self.cv:
while self.value == 0:
self.cv.wait()
self.value -= 1
counter = SharedCounter()
def worker():
print('Starting worker')
for i in range(10):
counter.increment()
print('Incremented: {}'.format(counter.value))
print('Exiting worker')
threads = []
for i in range(5):
t = threading.Thread(target=worker)
t.start()
threads.append(t)
for t in threads:
t.join()
print('Final value: {}'.format(counter.value))
```
在这个例子中,我们创建了一个 SharedCounter 类,它包含一个整数值和一个条件变量。increment() 和 decrement() 方法分别用于增加和减少计数器的值。当计数器的值为 0 时,decrement() 方法会阻塞等待条件变量的发生。当 increment() 方法执行完成后,它会通知所有等待条件变量的线程,让它们继续执行。
我们创建了 5 个线程来同时访问 SharedCounter 对象,并在每个线程中调用 increment() 方法 10 次。由于 increment() 方法使用了条件变量,线程之间可以协调和同步,保证计数器的值始终正确。最后,我们输出了计数器的最终值。
总的来说,条件变量是一种非常有用的同步机制,可以用于解决多线程程序中的一些复杂问题。在 Python 中,使用 threading 模块的 Condition 类来实现条件变量非常简单。
阅读全文
相关推荐
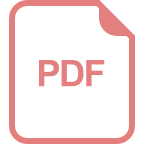
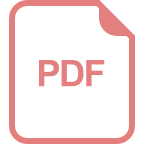
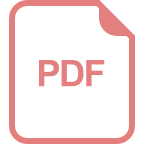
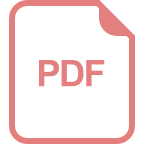
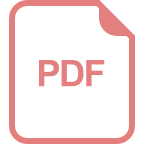
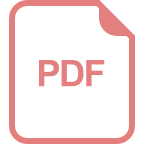
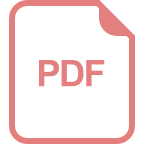
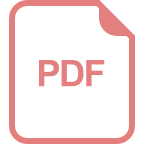
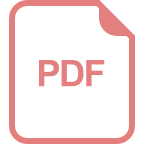
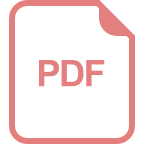
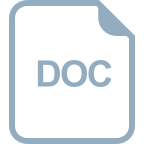
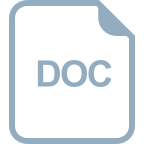
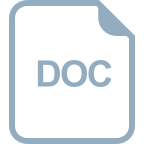
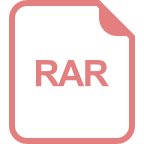