如何对孤立森林模型的精度、精确率、召回率、F1值、重构误差、平均路径长度、离群得分进行评估,给出具体python代码
时间: 2023-09-27 10:03:57 浏览: 210
孤立森林是一种基于树的离群检测算法,用于检测异常点。在使用孤立森林模型时,可以使用以下指标来评估其效果:
1. 精度(Accuracy):模型正确预测的样本数占总样本数的比例。
2. 精确率(Precision):真正例(TP)占预测为正例(TP+FP)的比例。
3. 召回率(Recall):真正例(TP)占实际为正例(TP+FN)的比例。
4. F1值(F1-Score):精确率和召回率的调和平均数。
5. 重构误差(Reconstruction Error):离群点的重构误差通常比正常点大,可以用于检测离群点。
6. 平均路径长度(Average Path Length):孤立森林可以计算每个样本到达根节点所需的路径长度,离群点通常具有较短的路径长度。
7. 离群得分(Outlier Score):孤立森林会为每个样本生成一个离群得分,越大的离群得分表示越可能是离群点。
下面是使用Python进行孤立森林模型评估的代码示例:
```python
from sklearn.ensemble import IsolationForest
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
from sklearn.metrics import mean_squared_error
import numpy as np
# 生成数据
X_train = np.random.randn(1000, 10)
X_test = np.random.randn(100, 10)
y_test = np.random.choice([0, 1], size=100, p=[0.9, 0.1])
# 构建孤立森林模型
iforest = IsolationForest(n_estimators=100, contamination=0.1, random_state=42)
iforest.fit(X_train)
# 预测测试集
y_pred = iforest.predict(X_test)
# 评估模型
acc = accuracy_score(y_test, y_pred)
prec = precision_score(y_test, y_pred)
rec = recall_score(y_test, y_pred)
f1 = f1_score(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
avg_path_length = iforest.decision_function(X_test).mean()
outlier_score = iforest.score_samples(X_test)
print('Accuracy:', acc)
print('Precision:', prec)
print('Recall:', rec)
print('F1-Score:', f1)
print('Mean Squared Error:', mse)
print('Average Path Length:', avg_path_length)
print('Outlier Score:', outlier_score)
```
其中,`IsolationForest`是Scikit-learn中实现孤立森林算法的类。`n_estimators`是构建的树的数量,`contamination`表示异常点的比例,`random_state`是随机种子,用于可复现结果。`decision_function`方法返回每个样本到达根节点所需的平均路径长度,`score_samples`方法返回每个样本的离群得分。
阅读全文
相关推荐
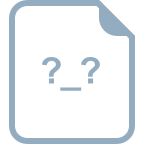
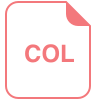
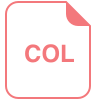
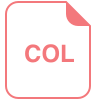
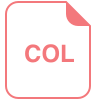
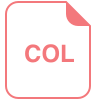
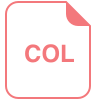
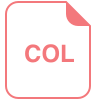
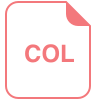
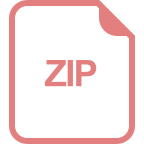
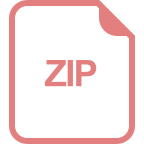