用python实现一个3*3卷积核,对一幅512*512*3的原始图像进行卷积操作。其中512*512*3分别表示图像的宽、高和通道数。
时间: 2023-08-14 08:02:13 浏览: 110
好的,我可以为您提供一个示例代码。在这个示例中,我们将使用Python中的NumPy库实现卷积操作。
首先,我们需要导入需要的库:
```python
import numpy as np
import cv2
```
然后,我们可以读取原始图像并将其转换为NumPy数组:
```python
img = cv2.imread('image.jpg')
img = np.array(img)
```
接下来,我们需要定义卷积核和卷积操作。在这个示例中,我们将使用一个随机生成的3 x 3的卷积核:
```python
kernel = np.array([[0.08, 0.12, -0.15],
[0.02, -0.05, 0.09],
[-0.07, 0.03, 0.01]])
```
然后,我们可以定义卷积操作:
```python
def convolution(img, kernel):
# 获取图像和卷积核大小
img_height, img_width, num_channels = img.shape
kernel_height, kernel_width = kernel.shape
# 创建一个空的输出图像
output = np.zeros((img_height - kernel_height + 1, img_width - kernel_width + 1, num_channels))
# 对于每个通道,从左到右、从上到下遍历图像
for channel in range(num_channels):
for i in range(img_height - kernel_height + 1):
for j in range(img_width - kernel_width + 1):
# 在图像上选取一个区域,与卷积核对应位置相乘,并将结果相加
output[i, j, channel] = np.sum(img[i:i+kernel_height, j:j+kernel_width, channel] * kernel)
return output.astype(np.uint8)
```
最后,我们可以调用卷积操作并保存结果:
```python
output = convolution(img, kernel)
cv2.imwrite('output.jpg', output)
```
完整示例代码如下:
```python
import numpy as np
import cv2
def convolution(img, kernel):
# 获取图像和卷积核大小
img_height, img_width, num_channels = img.shape
kernel_height, kernel_width = kernel.shape
# 创建一个空的输出图像
output = np.zeros((img_height - kernel_height + 1, img_width - kernel_width + 1, num_channels))
# 对于每个通道,从左到右、从上到下遍历图像
for channel in range(num_channels):
for i in range(img_height - kernel_height + 1):
for j in range(img_width - kernel_width + 1):
# 在图像上选取一个区域,与卷积核对应位置相乘,并将结果相加
output[i, j, channel] = np.sum(img[i:i+kernel_height, j:j+kernel_width, channel] * kernel)
return output.astype(np.uint8)
# 读取原始图像
img = cv2.imread('image.jpg')
img = np.array(img)
# 定义卷积核
kernel = np.array([[0.08, 0.12, -0.15],
[0.02, -0.05, 0.09],
[-0.07, 0.03, 0.01]])
# 进行卷积操作
output = convolution(img, kernel)
# 保存结果
cv2.imwrite('output.jpg', output)
```
请注意,这只是一个简单的示例,实际应用中可能需要更复杂的卷积核和卷积操作。同时,为了提高卷积操作的效率,可以使用更高效的算法和库。
阅读全文
相关推荐
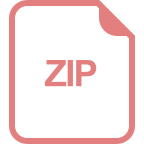











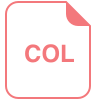
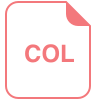
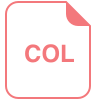
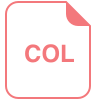
