Use python Count the integer that appears most frequently in an integer sequence and its frequency
时间: 2024-09-29 08:06:58 浏览: 33
To count the most frequently occurring integer in an integer sequence and its frequency using Python, you can use the `collections.Counter` class. Here's a step-by-step explanation:
1. **Import necessary libraries**
```python
from collections import Counter
```
2. **Create your integer sequence**
```python
# Replace this with your actual integer sequence
integer_sequence = [1, 2, 3, 2, 4, 2, 5, 6, 2, 1, 1, 3, 4, 4]
```
3. **Count occurrences with Counter**
```python
counter = Counter(integer_sequence)
```
4. **Find the most common integer and its frequency**
```python
most_common_integer, frequency = counter.most_common(1)[0]
```
Here, `most_common(1)` returns a list with one tuple containing the most common item and its count, so we unpack it into separate variables.
**Full example:**
```python
from collections import Counter
# Integer sequence
integer_sequence = [1, 2, 3, 2, 4, 2, 5, 6, 2, 1, 1, 3, 4, 4]
# Count occurrences
counter = Counter(integer_sequence)
# Find most common integer and frequency
most_common_integer, frequency = counter.most_common(1)[0]
print(f"The most frequent integer is {most_common_integer} with a frequency of {frequency}.")
```
Remember to replace `integer_sequence` with your actual data if needed.
阅读全文
相关推荐
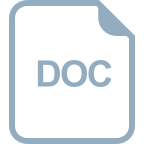
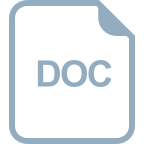
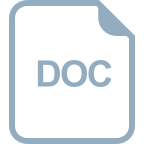















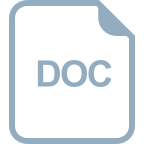