vue中用three写一个开关柜
时间: 2024-05-03 21:22:46 浏览: 83
由于开关柜是一个比较复杂的模型,需要一定的三维建模技巧和经验,因此在此只提供一个简单的示例,以便理解如何在Vue中使用Three.js来创建3D模型。
首先,需要安装Three.js和OrbitControls库:
```
npm install three orbit-controls
```
然后,在Vue组件中引入Three.js和OrbitControls库:
```
import * as THREE from 'three';
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls';
```
接着,在Vue组件的mounted钩子函数中创建场景、相机、渲染器和控制器:
```
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, this.$refs.canvas.clientWidth / this.$refs.canvas.clientHeight, 0.1, 1000);
this.camera.position.set(0, 0, 10);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer({ canvas: this.$refs.canvas, antialias: true });
this.renderer.setSize(this.$refs.canvas.clientWidth, this.$refs.canvas.clientHeight);
this.renderer.setClearColor(0xffffff);
// 创建控制器
this.controls = new OrbitControls(this.camera, this.renderer.domElement);
this.controls.enableDamping = true;
this.controls.dampingFactor = 0.25;
this.controls.rotateSpeed = 0.35;
this.controls.enableZoom = false;
this.controls.enablePan = false;
this.controls.enableKeys = false;
// 绑定动画函数
this.renderScene();
}
```
接下来,可以创建一个简单的开关柜模型,如下所示:
```
const cabinetGeometry = new THREE.BoxGeometry(2, 3, 1.5);
const cabinetMaterial = new THREE.MeshStandardMaterial({ color: 0x808080 });
const cabinet = new THREE.Mesh(cabinetGeometry, cabinetMaterial);
this.scene.add(cabinet);
```
最后,在动画函数中更新控制器和渲染器,并在每帧更新开关柜的旋转角度:
```
renderScene() {
requestAnimationFrame(this.renderScene);
this.controls.update();
const time = performance.now() / 1000;
const angle = time * Math.PI * 0.5;
this.scene.children[0].rotation.y = angle;
this.renderer.render(this.scene, this.camera);
}
```
完整代码如下:
```
<template>
<div>
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
import * as THREE from 'three';
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls';
export default {
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, this.$refs.canvas.clientWidth / this.$refs.canvas.clientHeight, 0.1, 1000);
this.camera.position.set(0, 0, 10);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer({ canvas: this.$refs.canvas, antialias: true });
this.renderer.setSize(this.$refs.canvas.clientWidth, this.$refs.canvas.clientHeight);
this.renderer.setClearColor(0xffffff);
// 创建控制器
this.controls = new OrbitControls(this.camera, this.renderer.domElement);
this.controls.enableDamping = true;
this.controls.dampingFactor = 0.25;
this.controls.rotateSpeed = 0.35;
this.controls.enableZoom = false;
this.controls.enablePan = false;
this.controls.enableKeys = false;
// 创建开关柜模型
const cabinetGeometry = new THREE.BoxGeometry(2, 3, 1.5);
const cabinetMaterial = new THREE.MeshStandardMaterial({ color: 0x808080 });
const cabinet = new THREE.Mesh(cabinetGeometry, cabinetMaterial);
this.scene.add(cabinet);
// 绑定动画函数
this.renderScene();
},
methods: {
renderScene() {
requestAnimationFrame(this.renderScene);
this.controls.update();
const time = performance.now() / 1000;
const angle = time * Math.PI * 0.5;
this.scene.children[0].rotation.y = angle;
this.renderer.render(this.scene, this.camera);
},
},
};
</script>
<style>
canvas {
width: 100%;
height: 100%;
display: block;
}
</style>
```
阅读全文
相关推荐
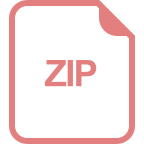
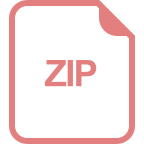
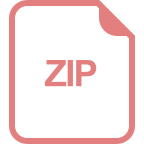
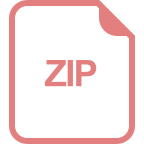
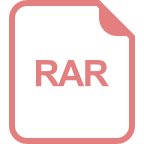
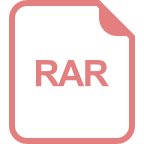
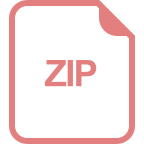
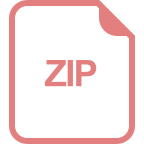
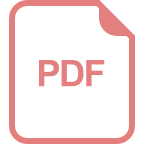
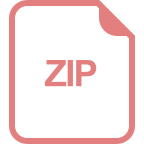
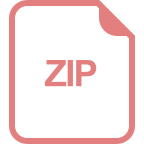
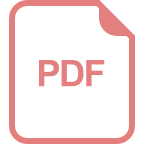
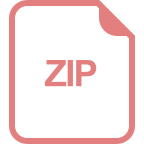
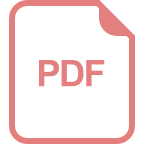
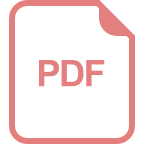