c语言在单链表指定位置删除元素
时间: 2024-12-10 20:13:04 浏览: 49
在C语言中,单链表是一种常见的数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。要在单链表的指定位置删除元素,可以按照以下步骤进行:
1. **定义链表节点结构**:首先定义一个链表节点的结构体,包含数据和指向下一个节点的指针。
2. **创建链表**:编写函数来创建链表,插入节点等操作。
3. **删除指定位置的节点**:编写函数来删除指定位置的节点。
下面是一个示例代码,展示了如何在单链表中删除指定位置的元素:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (!newNode) {
printf("Memory allocation error\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在链表末尾插入节点
void append(struct Node** headRef, int data) {
struct Node* newNode = createNode(data);
if (*headRef == NULL) {
*headRef = newNode;
return;
}
struct Node* temp = *headRef;
while (temp->next != NULL)
temp = temp->next;
temp->next = newNode;
}
// 删除指定位置的节点
void deleteNode(struct Node** headRef, int position) {
if (*headRef == NULL)
return;
struct Node* temp = *headRef;
// 如果头节点需要被删除
if (position == 0) {
*headRef = temp->next;
free(temp);
return;
}
// 找到要删除节点的前一个节点
for (int i = 0; temp != NULL && i < position - 1; i++)
temp = temp->next;
// 如果位置超出范围
if (temp == NULL || temp->next == NULL) {
printf("Position out of range\n");
return;
}
// 节点将被删除
struct Node* next = temp->next->next;
free(temp->next);
temp->next = next;
}
// 打印链表
void printList(struct Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
// 主函数
int main() {
struct Node* head = NULL;
// 创建链表 1->2->3->4->5
for (int i = 1; i <= 5; i++)
append(&head, i);
printf("Original list: \n");
printList(head);
// 删除位置2的节点
deleteNode(&head, 2);
printf("\nList after deleting node at position 2: \n");
printList(head);
return 0;
}
```
在这个示例中,我们首先定义了一个链表节点结构 `Node`,然后编写了 `createNode` 函数来创建新节点,`append` 函数来在链表末尾插入节点,`deleteNode` 函数来删除指定位置的节点,最后是 `printList` 函数来打印链表。
在 `main` 函数中,我们创建了一个链表并插入了5个节点,然后删除了位置2的节点并打印了结果。
阅读全文
相关推荐
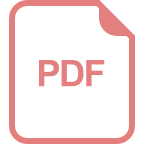
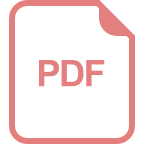
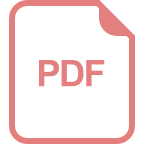




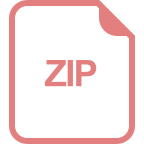
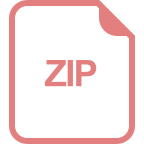
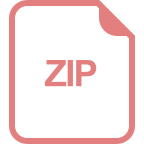
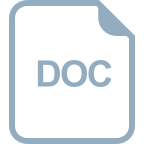






