currentPage.value=val;先把val强制转型为int型,再赋值给currentPage.value
时间: 2024-09-20 11:10:21 浏览: 36
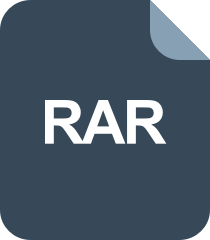
vue分页插件_vue.js分页插件下载
在JavaScript中,如果你想把一个变量`val`赋值给另一个表示整数的`currentPage.value`,通常你会先确保`val`是可转换成整数的类型,因为直接赋值可能会导致类型不匹配错误。以下是具体的步骤:
```javascript
// 假设val是一个字符串或者其他非整数类型
let val = '5'; // 或者其他值
// 使用parseInt()函数将val转换为整数,如果val不能转换为整数,则结果将是NaN
let intVal = parseInt(val);
// 检查转换后的intVal是否是Number类型的并且不是一个特殊的“非数字”值(例如 NaN)
if (typeof intVal === 'number' && !isNaN(intVal)) {
// 如果转换成功,就赋值给currentPage.value
currentPage.value = intVal;
} else {
// 如果转换失败,可能会抛出异常或者处理错误
console.error('Failed to convert value to integer.');
}
```
这样做的目的是为了保证数据类型的一致性和避免运行时错误。
阅读全文
相关推荐
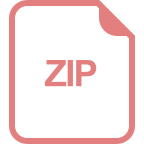
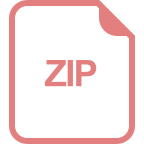

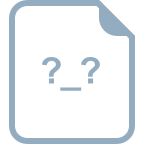
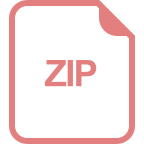
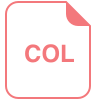











