searchData(bool) { this.currentPage = 1; if (bool) { // 前端实现模糊查询--不用对接口 let newListData = []; // 用于存放搜索出来数据的新数组 if (this.inputVal) { this.monitorData.filter((item) => { if (item.name.indexOf(this.inputVal) !== -1) { newListData.push(item); } }); } this.monitorData = newListData; } else { this.refreshData();
时间: 2024-02-14 08:19:26 浏览: 28
这段代码是一个 Vue 组件中的一个方法,名为 searchData。该方法接收一个 bool 类型的参数,用于判断是否需要进行模糊查询。
如果需要进行模糊查询,首先将当前页数 currentPage 置为 1,然后通过前端实现模糊查询,将符合条件的数据存入一个新数组 newListData 中,最后将数据源 monitorData 替换为 newListData。
如果不需要进行模糊查询,则调用 refreshData 方法来刷新数据。
需要注意的是,该方法中涉及到的变量 this.currentPage、this.inputVal、this.monitorData 等都是该组件中的数据属性。
相关问题
下面代码改为vue3的最新代码 data中: inputVal: "", // 输入框的输入值 monitorData: [], // 整个表数据 total: 0, // 总条数,分页时有用 watch中:设置监听,当输入框中的值改变时会实时的查询相应表数据(点击查询按钮也可以) watch: { inputVal(newValue) { if (newValue) { this.searchData(true); } else { this.searchData(false); } }, }, methods中: // 右上角搜索框 searchData(bool) { this.currentPage = 1; if (bool) { let paramName = this.inputVal; selectByName(paramName).then((res) => { this.monitorData = res.data.data; this.total = res.data.data.length; }); } else { this.refreshData(); // 更新表 } }
在Vue3中,代码可以进行一些修改来适配最新的语法和特性。下面是修改后的代码:
```vue
<template>
<div>
<input v-model="inputVal" @input="handleSearch">
<select v-model="selectedOption">
<option v-for="option in options" :key="option.id" :value="option.value">{{ option.label }}</option>
</select>
</div>
</template>
<script>
import { ref, watch } from 'vue';
import axios from 'axios';
export default {
setup() {
const inputVal = ref('');
const options = ref([]);
watch(inputVal, (newValue) => {
if (newValue) {
searchData(true);
} else {
searchData(false);
}
});
async function searchData(bool) {
try {
if (bool) {
let paramName = inputVal.value;
const response = await axios.get('/api/options', {
params: {
search: paramName,
},
});
options.value = response.data;
} else {
refreshData();
}
} catch (error) {
console.error(error);
}
}
async function refreshData() {
// 更新表格数据的逻辑
}
return {
inputVal,
options,
handleSearch: searchData,
};
},
};
</script>
```
在上面的示例中,我们使用了Vue 3的Composition API来重写了代码。使用了`ref`函数来创建响应式数据`inputVal`和`options`,替代了Vue 2中的`data`对象。我们也使用了`watch`函数来设置对`inputVal`的监听,当输入框的值改变时,会调用`searchData`方法进行搜索操作。
注意,在`searchData`方法中,我们使用了`inputVal.value`来获取`inputVal`的值,因为在Vue 3中,响应式数据是通过`.value`访问的。
另外,需要注意的是,上述代码只是一个示例,你需要根据你的实际情况进行适当的修改和调整。确保后端API能够正确处理模糊查询逻辑并返回正确的结果。
start() { this.startShow = true; // let oneDay = 24 * 60 * 60 * 1000; this.startTime = new Date().getFullYear(); this.searchData.startTime = new Date(); this.$nextTick(() => { // 删除月份列 let columns = document.querySelectorAll('.van-picker__columns .van-picker-column') if (columns.length === 2) { columns[1].parentElement.removeChild(columns[1]) } }) },
这段代码是一个函数的开始部分。它包含了一些操作:
1. 设置了一个名为`startShow`的属性为`true`。
2. 创建了一个`Date`对象,将当前的年份赋值给`startTime`。
3. 将一个新的`Date`对象赋值给`searchData.startTime`。
4. 使用`$nextTick`方法,在下一个DOM更新周期执行回调函数。
5. 在回调函数中,通过选择器获取所有具有`.van-picker__columns .van-picker-column`类的元素,并将其存储在`columns`变量中。
6. 如果列的数量为2,则从父元素中删除第二个列。
这段代码的目的可能是初始化某个功能或界面的状态。
相关推荐
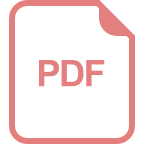
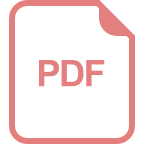













