年月日加一天的c语言
时间: 2024-09-30 12:10:52 浏览: 30
在C语言中,如果你想将当前日期加一天并保持年月日的形式,你可以使用`<time.h>`库中的`struct tm`结构和`localtime`、`strftime`函数。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 将时间戳转换为结构体tm
void add_one_day(time_t *timestamp) {
struct tm *new_time = localtime(timestamp);
++(*new_time); // 自增表示增加一天
// 将调整后的tm结构体转换回字符串格式的时间
char formatted_date[30];
strftime(formatted_date, sizeof(formatted_date), "%Y-%m-%d", new_time);
printf("New date: %s\n", formatted_date);
}
int main() {
time_t current_time = time(0); // 获取当前时间戳
add_one_day(¤t_time);
return 0;
}
```
在这个程序中,我们首先获取当前时间(`time(0)`),然后通过`localtime`将其转换为`struct tm`以便于操作。接着我们将日期部分自增1,最后使用`strftime`格式化输出加一天后的日期。
相关问题
c语言输入年月日判断哪一天
C语言可以通过输入年、月、日来判断是星期几。首先,我们需要将输入的年月日存储在相应的变量中。然后,通过一些计算来判断这一天是星期几。
首先,我们可以使用以下公式将输入的年份、月份和天数转换为一个较小的数值(以星期几的顺序计算):
Zeller公式:h = (q + (13*(m+1))/5 + K + K/4 - K/100 + K/400) % 7
其中,h代表星期几,q代表天数,m代表月份(3代表3月,4代表4月,... 12代表12月,1代表1月,2代表2月),K代表年份的最后两位数。
最后,根据公式计算的结果,我们可以用一个switch语句来确定星期几:
switch (h) {
case 0:
printf("这一天是星期日。\n");
break;
case 1:
printf("这一天是星期一。\n");
break;
case 2:
printf("这一天是星期二。\n");
break;
case 3:
printf("这一天是星期三。\n");
break;
case 4:
printf("这一天是星期四。\n");
break;
case 5:
printf("这一天是星期五。\n");
break;
case 6:
printf("这一天是星期六。\n");
break;
default:
printf("输入无效。\n");
break;
}
这样,根据输入的年月日,我们就可以判断出这一天是星期几了。当然,以上只是一种方法,还有其他的算法和方法也可以实现这个功能。
用c语言编写年月日的下一天
在C语言中,要编写一个函数来计算给定日期的下一天,可以使用`struct`来存储日期,并处理闰年的情况。首先,创建一个结构体表示日期:
```c
#include <stdio.h>
#include <stdbool.h>
// 定义日期结构体
typedef struct {
int day;
int month;
int year;
} Date;
// 判断是否为闰年的辅助函数
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 != 0)
return true;
else if (year % 400 != 0)
return false;
else
return true;
}
// 计算日期的下一天
Date next_day(Date date) {
// 检查月份
switch(date.month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
date.day += 1;
break;
case 2: // 转换到平年后的二月天数
if (!is_leap_year(date.year)) {
date.day = 28;
} else {
date.day = 29; // 闰年的二月有29天
}
break;
case 4: case 6: case 9: case 11:
date.day += 1;
break;
default:
// 不合法的月份,保持不变
break;
}
// 如果是闰年并且当前月份是2月,检查是否超出一年的天数
if (date.month == 2 && is_leap_year(date.year) && date.day > 29)
date.day = 1, date.month++;
// 如果是12月,则切换到次年1月
if (date.month == 12) {
date.month = 1;
date.year++;
}
return date;
}
int main() {
Date today = {1, 1, 2023}; // 示例输入日期
Date tomorrow = next_day(today);
printf("今天是%d-%d-%d,明天是%d-%d-%d.\n", today.day, today.month, today.year,
tomorrow.day, tomorrow.month, tomorrow.year);
return 0;
}
```
阅读全文
相关推荐
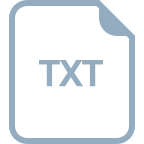
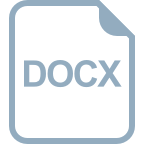













