C语言写输入年月日输出下一天的日期
时间: 2024-09-30 09:11:24 浏览: 7
在C语言中,处理日期通常涉及到一些特定的库或者手动计算,因为C标准库本身并不直接提供日期处理功能。以下是一个简单的示例,使用`struct`来存储日期,并通过加一来计算下一天:
```c
#include <stdio.h>
// 定义日期结构
typedef struct {
int day;
int month;
int year;
} Date;
// 计算下一天
Date next_day(Date date) {
if (date.day == 31 && date.month == 12) { // 如果是闰年的12月31日
if ((date.year % 4 == 0 && date.year % 100 != 0) || date.year % 400 == 0) {
return (Date){1, 1, date.year + 1}; // 新的一年第一天
} else {
return (Date){1, 1, date.year}; // 平年的12月31日后就是新年
}
} else if (date.month == 12) {
return (Date){1, 1, date.year + 1}; // 12月最后一天之后是新一年的第一天
} else {
++date.day; // 其他月份只需加一天
return date;
}
}
int main() {
Date today = {__DATE__, __MONTH__, __YEAR__};
Date tomorrow = next_day(today);
printf("Today is: %d-%d-%d\n", tomorrow.day, tomorrow.month, tomorrow.year);
return 0;
}
```
请注意,这个例子使用了预处理器宏`__DATE__`、`__MONTH__`和`__YEAR__`来获取当前日期,这需要编译环境支持这些特性。在实际项目中,你可以选择使用更专业的日期处理库如`dateutil`或者编写自定义函数来处理日期。
相关推荐
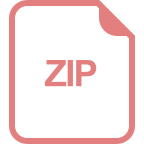
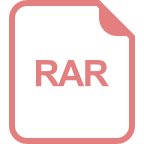













