c语言输入年月日输出它是该年的第几天
时间: 2024-09-29 17:01:29 浏览: 11
C语言可以通过编写程序来输入年月日,然后输出这一天是该年的第几天。具体实现方法如下:
```
#include <stdio.h>
int main() {
int year, month, day;
int days[] = {0,31,59,90,120,151,181,212,243,273,304,334};
// 用数组保存每个月份前面的天数,不考虑闰年
printf("请输入日期(年 月 日):");
scanf("%d %d %d", &year, &month, &day);
int total_days = days[month-1] + day; // 计算该日期到当年1月1日的天数
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) { // 判断是否为闰年
if (month > 2) {
total_days++; // 如果是闰年且输入的月份大于2月,则要加上1天
}
}
printf("这是%d年的第%d天\n", year, total_days);
return 0;
}
```
相关问题
C语言输出输入年月日是这一年的第几天。
在C语言中,要计算给定日期是一年中的第几天,可以使用蔡勒公式(Zeller's congruence),它是一个古老的算法,用于确定儒略日(Julian day number)。这是一个常见的编程练习,涉及到对闰年规则的理解以及模运算。以下是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
int zeller_congruence(int year, int month, int day) {
if (month < 3) { // 如果是1月或2月,调整年份和月份
year -= 1;
month += 12;
}
int q = day;
int m = month;
int k = year % 100; // 年份的最后两位数
int j = year / 100; // 去掉最后两位后的年份
int result = (q + ((13 * (m + 1)) / 5) + k + (k / 4) + (j / 4) - (2 * j));
if (j >= 1900) {
result -= (j / 100) * 5; // 纠正20世纪的额外天数
}
return result;
}
int main() {
int year, month, day;
printf("请输入年月日(格式:YYYY MM DD): ");
scanf("%d %d %d", &year, &month, &day);
int julian_day = zeller_congruence(year, month, day);
printf("该日期是一年中的第%d天.\n", julian_day);
return 0;
}
```
在这个程序中,`zeller_congruence`函数接受年、月、日作为参数,返回对应的儒略日。然后在`main`函数中获取用户输入并计算结果。
c语言输入一个年月日输出是这一年的第几天
以下是一种实现方法:
```c
#include <stdio.h>
int main() {
int year, month, day;
printf("请输入年月日,以空格分隔:\n");
scanf("%d %d %d", &year, &month, &day);
// 每月的天数,注意二月根据是否为闰年而不同
int daysInMonth[] = {31, 28 + ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int dayOfYear = 0;
// 先累加该月之前的天数
for (int i = 0; i < month - 1; i++) {
dayOfYear += daysInMonth[i];
}
// 再加上当月的天数
dayOfYear += day;
printf("该日期在这一年的第 %d 天\n", dayOfYear);
return 0;
}
```
该程序首先在屏幕上提示用户输入年月日,然后使用 `scanf()` 函数读取用户输入的值。随后定义一个整型数组 `daysInMonth[]`,存储每个月的天数。注意二月的天数需要根据是否为闰年而不同。接下来使用一个循环累加该月之前的天数,最后加上当月的天数就可以得到这一年的第几天了。最后将结果输出到屏幕上。
相关推荐
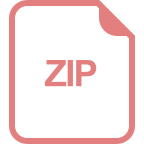
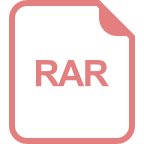












