输入一个日期(年月日),输出该日期的前一天。用C语言实现
时间: 2024-10-12 15:04:37 浏览: 63
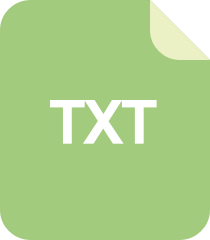
c语言输入年月日且能防止错误输入
在C语言中,我们可以使用`<time.h>`头文件中的`struct tm`数据结构来处理日期时间,并利用`localtime`函数将其转换为便于操作的格式。下面是一个简单的程序,它接受用户输入的日期(如"20230305"表示2023年3月5日),然后返回前一天的日期:
```c
#include <stdio.h>
#include <time.h>
void get_previous_day(char date[10]) {
// 将字符串转换为时间戳(struct tm)
struct tm time_struct;
sscanf(date, "%d%d%d", &time_struct.tm_year, &time_struct.tm_mon, &time_struct.tm_mday);
// 减去一天
time_struct.tm_mday--;
// 如果新日期小于1,则将月份减一(注意:月份是从0开始计数的)
if (time_struct.tm_mday == 0) {
time_struct.tm_mday = 31;
time_struct.tm_mon--;
// 如果月份变为负数,表示已经到上一年了,加回365
if (time_struct.tm_mon < 0)
time_struct.tm_year -= 1;
}
// 设置时分秒为0
time_struct.tm_hour = 0;
time_struct.tm_min = 0;
time_struct.tm_sec = 0;
// 将struct tm转换回本地时间
time_t timestamp = mktime(&time_struct);
// 格式化输出日期
char output_date[10];
strftime(output_date, sizeof(output_date), "%Y%m%d", &time_struct);
printf("前一天的日期是:%s\n", output_date);
}
int main() {
char input_date[10];
printf("请输入日期(格式:YYYYMMDD): ");
fgets(input_date, sizeof(input_date), stdin);
input_date[strcspn(input_date, "\n")] = '\0'; // 去除换行符
get_previous_day(input_date);
return 0;
}
```
当你运行这个程序并输入一个日期后,它会打印出前一天的日期。
阅读全文
相关推荐
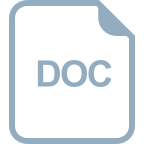
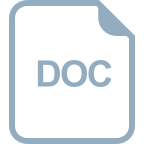






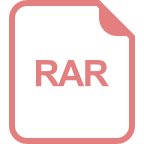
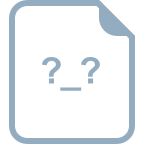
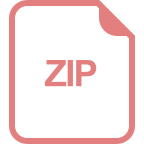
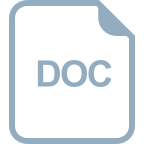





