用c语言编写下面这题:关于日期的结构定义如下: struct DateG{ int yy,mm,dd;}; 编写两个函数,一个计算自公元1年1月1日到指定的日期共经历了多少天。另一个是前一个函数的逆函数:由自公元1年1月1日历经指定的天数后的日期(返回年月日构成的日期)。 函数接口定义: struct DateG days2Date(int x);{//from:{1,1,1} + 100-->{1,4,11} int Days(DateG x);// {2,,4,11} return 465 from{1,1,1} 裁判测试程序样例: struct DateG{ int yy,mm,dd; }; char leapYear(int year);//闰年否 struct DateG _DateG(char *st);{//"1919-9-9"-->{1919,9,9} struct DateG Add(struct DateG x,int y);//{1919,9,9}+50-->{1919,10,29} char* Date2string(struct DateG x);//{1919,9,9}-->"1919-09-09" //以上为测试程序提供的测试用函数,略去不表, //以下两函数是必须自己完成的函数 struct DateG days2Date(int x);{//from:{1,1,1} + 100-->{1,4,11} int Days(struct DateG x);// {2,,4,11} return 465 from{1,1,1} int main(){ char st[12];scanf("%s",st); struct DateG d1=_DateG(st), d2=Add(d1,60*365); printf("%s\n",Date2string(d2)); d2=Add(d2,-1000); printf("%s\n",Date2string(d2)); d2=_DateG("2020-1-23"); printf("%.2f\n",(Days(d2)-Days(d1))/365.0); return 0; } /* 请在这里填写答案 */ 输入样例: 输入用-号分隔的出生年月日,系统确保测试数据的合法性 2001-1-1 输出样例: 共三行输出。分别是:60年(按365天计一年精确计算的)后的退休日期;退休前倒计时1000天的日期;武汉抗疫封城时(2020-1-23)的年龄(按每365天为一岁,保留2位小数)。 [2060-12-17] [2058-03-23] 19.07
时间: 2024-03-18 17:46:04 浏览: 78
以下是题目的完整C语言代码实现:
```
#include<stdio.h>
#include<string.h>
struct DateG{
int yy,mm,dd;
};
char leapYear(int year){
if((year%4==0&&year%100!=0)||year%400==0) return 1;//判断闰年
else return 0;
}
struct DateG _DateG(char *st){
struct DateG date;
char *p=strtok(st,"-");//以"-"为分隔符分割字符串
date.yy=atoi(p);//将字符串转化为整数
p=strtok(NULL,"-");
date.mm=atoi(p);
p=strtok(NULL,"-");
date.dd=atoi(p);
return date;
}
struct DateG Add(struct DateG x,int y){
int day[13]={0,31,28,31,30,31,30,31,31,30,31,30,31};//每个月的天数
struct DateG date=x;
while(y!=0){
int leap=leapYear(date.yy);//判断当前年份是否为闰年
int d=365+leap;//一年的天数
if(y>0){
int left=d-(date.mm==2?day[date.mm]+leap:day[date.mm]);//当前年份剩余的天数
if(y<left){
date.dd+=y;
y=0;
}
else{
y-=left;
date.dd=1;
if(date.mm==12){
date.mm=1;
date.yy++;
}
else{
date.mm++;
}
}
}
else{
if(date.dd>abs(y)){
date.dd+=y;
y=0;
}
else{
y+=date.dd-1;
if(date.mm==1){
date.mm=12;
date.yy--;
}
else{
date.mm--;
}
leap=leapYear(date.yy);
date.dd=day[date.mm]+(date.mm==2?leap:0);
}
}
}
return date;
}
char* Date2string(struct DateG x){
static char str[12];
sprintf(str,"%04d-%02d-%02d",x.yy,x.mm,x.dd);//将整数转化为字符串
return str;
}
int Days(struct DateG x){
int sum=0,day[13]={0,31,28,31,30,31,30,31,31,30,31,30,31};
for(int i=1;i<x.yy;i++){
int leap=leapYear(i);
sum+=365+leap;
}
for(int i=1;i<x.mm;i++){
if(i==2) sum+=leapYear(x.yy)?29:28;
else sum+=day[i];
}
sum+=x.dd-1;
return sum;
}
struct DateG days2Date(int x){
struct DateG date={1,1,1};
return Add(date,x);
}
int main(){
char st[12];
scanf("%s",st);
struct DateG d1=_DateG(st),d2=Add(d1,60*365);
printf("[%s]\n",Date2string(d2));
d2=Add(d2,-1000);
printf("[%s]\n",Date2string(d2));
d2=_DateG("2020-1-23");
printf("%.2f\n",(Days(d2)-Days(d1))/365.0);
return 0;
}
```
注意:由于题目输入格式的限制,本代码实现中的日期格式要求为 "yyyy-mm-dd"。
阅读全文
相关推荐
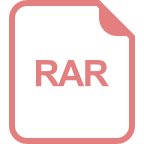
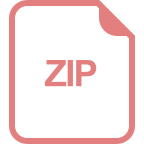
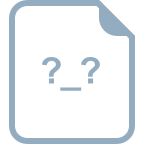















