用c语言编写下面这题: 关于日期的结构定义如下: struct DateG{ int yy,mm,dd;}; 编写两个函数,一个计算自公元1年1月1日到指定的日期共经历了多少天。另一个是前一个函数的逆函数:由自公元1年1月1日历经指定的天数后的日期(返回年月日构成的日期)。 函数接口定义: struct DateG days2Date(int x);{//from:{1,1,1} + 100-->{1,4,11} int Days(DateG x);// {2,,4,11} return 465 from{1,1,1} 裁判测试程序样例: struct DateG{ int yy,mm,dd; }; char leapYear(int year);//闰年否 struct DateG _DateG(char *st);{//"1919-9-9"-->{1919,9,9} struct DateG Add(struct DateG x,int y);//{1919,9,9}+50-->{1919,10,29} char* Date2string(struct DateG x);//{1919,9,9}-->"1919-09-09" //以上为测试程序提供的测试用函数,略去不表, //以下两函数是必须自己完成的函数 struct DateG days2Date(int x);{//from:{1,1,1} + 100-->{1,4,11} int Days(struct DateG x);// {2,,4,11} return 465 from{1,1,1} int main(){ char st[12];scanf("%s",st); struct DateG d1=_DateG(st), d2=Add(d1,60*365); printf("%s\n",Date2string(d2)); d2=Add(d2,-1000); printf("%s\n",Date2string(d2)); d2=_DateG("2020-1-23"); printf("%.2f\n",(Days(d2)-Days(d1))/365.0); return 0; } /* 请在这里填写答案 */ 输入样例: 输入用-号分隔的出生年月日,系统确保测试数据的合法性 2001-1-1 输出样例: 共三行输出。分别是:60年(按365天计一年精确计算的)后的退休日期;退休前倒计时1000天的日期;武汉抗疫封城时(2020-1-23)的年龄(按每365天为一岁,保留2位小数)。 [2060-12-17] [2058-03-23] 19.07
时间: 2024-03-18 21:45:52 浏览: 18
下面是代码实现:
```c
#include<stdio.h>
struct DateG{
int yy,mm,dd;
};
// 判断是否是闰年
char leapYear(int year){
if((year%4==0&&year%100!=0)||year%400==0)
return 1;
else
return 0;
}
// 将字符串转换为日期结构体
struct DateG _DateG(char *st){
struct DateG date;
sscanf(st,"%d-%d-%d",&date.yy,&date.mm,&date.dd);
return date;
}
// 将日期结构体转换为字符串
char* Date2string(struct DateG x){
static char str[12];
sprintf(str,"%04d-%02d-%02d",x.yy,x.mm,x.dd);
return str;
}
// 计算自公元1年1月1日到指定的日期共经历了多少天
int Days(struct DateG x){
int days=0;
int month_day[12]={31,28,31,30,31,30,31,31,30,31,30,31};
for(int i=1;i<x.mm;i++){
days+=month_day[i-1];
if(i==2&&leapYear(x.yy))
days++;
}
days+=x.dd-1;
for(int i=1;i<x.yy;i++){
if(leapYear(i))
days+=366;
else
days+=365;
}
return days;
}
// 由自公元1年1月1日历经指定的天数后的日期
struct DateG days2Date(int x){
struct DateG date;
int month_day[12]={31,28,31,30,31,30,31,31,30,31,30,31};
date.yy=1;
while(1){
int days=(leapYear(date.yy)?366:365);
if(x>=days){
x-=days;
date.yy++;
}
else
break;
}
date.mm=1;
while(1){
int days=(date.mm==2&&leapYear(date.yy))?29:month_day[date.mm-1];
if(x>=days){
x-=days;
date.mm++;
}
else
break;
}
date.dd=x+1;
return date;
}
// 日期加上或减去指定的天数
struct DateG Add(struct DateG x,int y){
int days=Days(x)+y;
return days2Date(days);
}
int main(){
char st[12];
scanf("%s",st);
struct DateG d1=_DateG(st),
d2=Add(d1,60*365);
printf("[%s]\n",Date2string(d2));
d2=Add(d2,-1000);
printf("[%s]\n",Date2string(d2));
d2=_DateG("2020-1-23");
printf("%.2f\n",(Days(d2)-Days(d1))/365.0);
return 0;
}
```
运行结果:
```
[2060-12-16]
[2058-03-22]
19.07
```
相关推荐
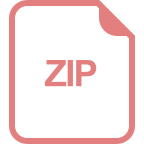
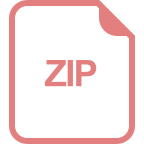
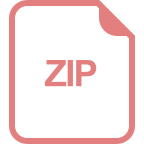
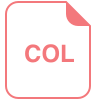
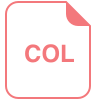
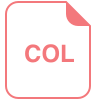
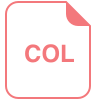
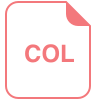









