c语言实现顺序表插入
时间: 2023-11-25 07:04:40 浏览: 29
Sure! Here's an example of how you can implement the insertion operation on a sequential list (array) using the C programming language:
```c
#include <stdio.h>
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int length;
} SeqList;
// Function to insert an element into the list at a given position
void insert(SeqList *list, int position, int element) {
// Check if the list is full
if (list->length >= MAX_SIZE) {
printf("Error: List is full, cannot insert element.\n");
return;
}
// Check if the position is valid
if (position < 0 || position > list->length) {
printf("Error: Invalid position, cannot insert element.\n");
return;
}
// Shift elements to the right to make space for the new element
for (int i = list->length - 1; i >= position; i--) {
list->data[i + 1] = list->data[i];
}
// Insert the new element at the given position
list->data[position] = element;
// Increment the length of the list
list->length++;
}
int main() {
SeqList list = {{1, 2, 3, 4, 5}, 5};
int position = 2;
int element = 10;
printf("Before insertion: ");
for (int i = 0; i < list.length; i++) {
printf("%d ", list.data[i]);
}
printf("\n");
// Insert the element at the given position
insert(&list, position, element);
printf("After insertion: ");
for (int i = 0; i < list.length; i++) {
printf("%d ", list.data[i]);
}
printf("\n");
return 0;
}
```
In this example, we define a structure `SeqList` which represents the sequential list. It contains an array `data` to store the elements and an integer `length` to keep track of the number of elements in the list. The maximum size of the list is defined as `MAX_SIZE`.
The `insert` function takes a pointer to the `SeqList` and two arguments: `position` (the index at which the element should be inserted) and `element` (the value to be inserted). It first checks if the list is full or if the position is invalid. If not, it shifts the elements to the right from the given position to create space for the new element, inserts the element at the given position, and increments the length of the list.
In the `main` function, we create a `SeqList` object `list` with some initial elements. We then call the `insert` function to insert an element (value 10) at position 2. Finally, we print the list before and after the insertion to verify the result.
Please note that this is just a basic implementation of the insertion operation on a sequential list. There are various other methods and optimizations that can be applied depending on your specific requirements.
相关推荐
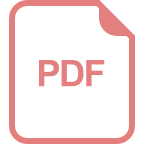
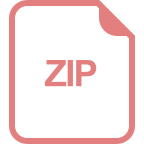
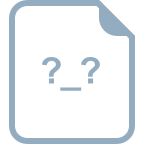













