java实现从某账户取款和存款的操作 1)输人存取款金额时,如果非数值型,捕获异常并进行处理(系统异常)。2)操作账户类时,如果取款数大于金额则作为异常处理(自定义异常)。具体的代码是什么
时间: 2024-10-21 17:10:25 浏览: 16
在Java中,我们可以创建一个简单的`Account`类来表示银行账户,然后定义`withdraw`(取款)和`deposit`(存款)方法。为了满足题目所描述的要求,我们将使用`try-catch`块来捕获`NumberFormatException`(非数值输入)和自定义的异常`InsufficientFundsException`(取款金额超过余额)。下面是示例代码:
```java
public class Account {
private double balance;
public Account(double initialBalance) {
this.balance = initialBalance;
}
// 存款方法
public void deposit(double amount) throws InsufficientFundsException {
if (amount <= 0) {
throw new IllegalArgumentException("Deposit amount must be a positive number.");
}
balance += amount;
System.out.println("Deposited: " + amount + ". New balance: " + balance);
}
// 取款方法
public void withdraw(double amount) throws InsufficientFundsException, NumberFormatException {
try {
double inputAsDouble = Double.parseDouble(String.valueOf(amount));
if (inputAsDouble < 0 || inputAsDouble > balance) {
throw new InsufficientFundsException("Insufficient funds to withdraw that amount.");
}
balance -= inputAsDouble;
System.out.println("Withdrew: " + amount + ". New balance: " + balance);
} catch (NumberFormatException e) {
System.out.println("Invalid input! Please enter a numerical value.");
}
}
// 自定义异常
static class InsufficientFundsException extends Exception {
public InsufficientFundsException(String message) {
super(message);
}
}
}
// 主函数示例
public static void main(String[] args) {
Account account = new Account(1000.0);
try {
account.deposit(500);
account.withdraw(200); // 这里正常操作不会抛出异常
account.withdraw("150"); // 这将触发NumberFormatException
} catch (InsufficientFundsException e) {
System.out.println(e.getMessage());
} catch (NumberFormatException e) {
System.out.println(e.getMessage());
}
}
```
在这个例子中,当用户尝试存款负数或取款超过余额时,会分别抛出自定义异常和`NumberFormatException`。同时,主函数展示了如何捕获并处理这两种异常。
阅读全文
相关推荐
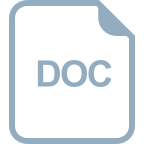
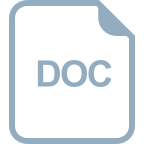
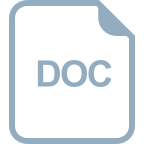



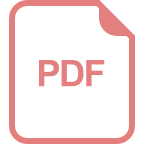
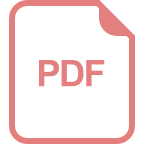
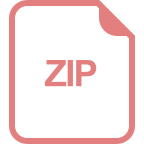
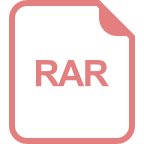
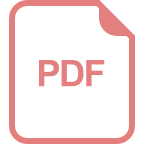
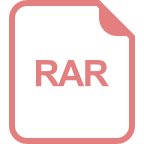
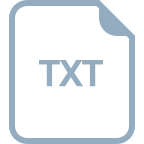
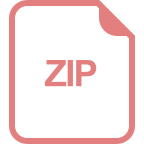
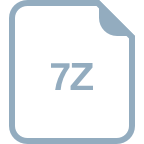
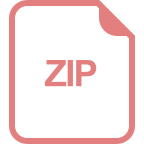
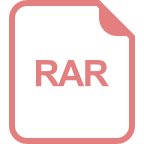