继承 多态 汽车租赁系统
时间: 2023-10-28 22:07:02 浏览: 121
继承和多态是面向对象编程中的重要概念。继承是指一个类可以继承另一个类的属性和方法,从而可以通过继承来实现代码的重用性和灵活性。多态是指同一个方法在不同的对象上可以有不同的实现,从而实现了代码的灵活性和扩展性。
在汽车租赁系统中,继承和多态可以被应用到不同类型的车辆上。比如,我们可以定义一个抽象的车辆类Moto_Vehilcle,然后定义轿车类Car和客车类Bus继承自Moto_Vehilcle类。这样,轿车和客车就可以共享Moto_Vehilcle类的属性和方法,并且可以分别实现各自特有的功能。
在这个系统中,轿车和客车都有claRent(int days)方法来计算租金,但是根据具体的天数,轿车和客车的计算方式是不同的。这就是多态的体现,相同的方法名,通过不同的对象调用,可以得到不同的结果。
相关问题
利用c++完成 任务2.汽车租赁系统 利用继承和多态知识点为汽车租赁中心开发一个汽车租赁系统,对租赁中心的汽车进行管理,主要如下: (1)基本功能:首先,输入租赁的天数,用于最后计算租赁的费用;其次,选择租赁的汽车类型、其次品牌、汽车型号和客车座位数;最后,系统给出分配的车辆信息和需要支付的租赁费用,以及计算出汽车租赁的总租金,运行效果如图3; (2)汽车租赁公司出租多种车辆,车型及租金情况可以参考图1: 并且使用外置类文件
很高兴为您解答这个问题。下面是一个简单的C++程序,实现任务2所需的汽车租赁系统,其中使用了继承和多态的知识点,并使用外置类文件。
Car.h(外置类文件):
```c++
#ifndef CAR_H
#define CAR_H
#include <string>
class Car {
protected:
std::string brand;
std::string model;
int dayRent;
public:
Car(const std::string& brand, const std::string& model, int dayRent);
virtual void printInfo() const;
virtual int getRent(int days) const;
};
class Sedan : public Car {
public:
Sedan(const std::string& brand, const std::string& model, int dayRent);
};
class SUV : public Car {
public:
SUV(const std::string& brand, const std::string& model, int dayRent);
};
class Bus : public Car {
protected:
int seatNum;
public:
Bus(const std::string& brand, const std::string& model, int dayRent, int seatNum);
virtual void printInfo() const override;
virtual int getRent(int days) const override;
};
#endif
```
Car.cpp(外置类文件):
```c++
#include "Car.h"
#include <iostream>
Car::Car(const std::string& brand, const std::string& model, int dayRent)
: brand(brand), model(model), dayRent(dayRent) {}
void Car::printInfo() const {
std::cout << "Brand: " << brand << " Model: " << model << " Rent: " << dayRent << std::endl;
}
int Car::getRent(int days) const {
return days * dayRent;
}
Sedan::Sedan(const std::string& brand, const std::string& model, int dayRent)
: Car(brand, model, dayRent) {}
SUV::SUV(const std::string& brand, const std::string& model, int dayRent)
: Car(brand, model, dayRent) {}
Bus::Bus(const std::string& brand, const std::string& model, int dayRent, int seatNum)
: Car(brand, model, dayRent), seatNum(seatNum) {}
void Bus::printInfo() const {
std::cout << "Brand: " << brand << " Model: " << model << " Rent: " << dayRent
<< " Seat number: " << seatNum << std::endl;
}
int Bus::getRent(int days) const {
if (seatNum <= 16) {
return days * dayRent;
}
else {
return days * (dayRent + 200);
}
}
```
Main.cpp:
```c++
#include <iostream>
#include <vector>
#include "Car.h"
using namespace std;
void rentCar(const vector<Car*>& cars) {
int days;
cout << "Enter the rental days: ";
cin >> days;
int carType, brandIndex, modelIndex;
cout << "Select the car type: 1.Sedan 2.SUV 3.Bus" << endl;
cin >> carType;
cout << "Select the brand: 1.Audi 2.BMW 3.Benz" << endl;
cin >> brandIndex;
cout << "Select the model: 1.A 2.B 3.C" << endl;
cin >> modelIndex;
Car* car = cars[(carType - 1) * 9 + (brandIndex - 1) * 3 + modelIndex - 1];
car->printInfo();
cout << "Rent: " << car->getRent(days) << endl;
}
int main() {
vector<Car*> cars;
cars.push_back(new Sedan("Audi", "A", 400));
cars.push_back(new Sedan("Audi", "B", 500));
cars.push_back(new Sedan("Audi", "C", 600));
cars.push_back(new Sedan("BMW", "A", 450));
cars.push_back(new Sedan("BMW", "B", 550));
cars.push_back(new Sedan("BMW", "C", 650));
cars.push_back(new Sedan("Benz", "A", 500));
cars.push_back(new Sedan("Benz", "B", 600));
cars.push_back(new Sedan("Benz", "C", 700));
cars.push_back(new SUV("Audi", "A", 800));
cars.push_back(new SUV("Audi", "B", 900));
cars.push_back(new SUV("Audi", "C", 1000));
cars.push_back(new SUV("BMW", "A", 850));
cars.push_back(new SUV("BMW", "B", 950));
cars.push_back(new SUV("BMW", "C", 1050));
cars.push_back(new SUV("Benz", "A", 900));
cars.push_back(new SUV("Benz", "B", 1000));
cars.push_back(new SUV("Benz", "C", 1100));
cars.push_back(new Bus("Audi", "A", 1500, 16));
cars.push_back(new Bus("Audi", "B", 1600, 16));
cars.push_back(new Bus("Audi", "C", 1700, 20));
cars.push_back(new Bus("BMW", "A", 1550, 16));
cars.push_back(new Bus("BMW", "B", 1650, 16));
cars.push_back(new Bus("BMW", "C", 1750, 20));
cars.push_back(new Bus("Benz", "A", 1600, 16));
cars.push_back(new Bus("Benz", "B", 1700, 16));
cars.push_back(new Bus("Benz", "C", 1800, 20));
rentCar(cars);
int totalRent = 0;
for (const auto& car : cars) {
totalRent += car->getRent(7);
}
cout << "Total rent: " << totalRent << endl;
for (const auto& car : cars) {
delete car;
}
return 0;
}
```
程序中使用了Car类作为基类,派生出Sedan、SUV、Bus三个类,并重写了printInfo和getRent方法。在main函数中,首先创建了一个包含所有汽车的vector,然后调用rentCar函数来选择租车,并输出租车信息和费用。最后计算所有汽车租金的总和,并释放动态分配的内存。
程序运行流程如下:
```
Enter the rental days: 5
Select the car type: 1.Sedan 2.SUV 3.Bus
1
Select the brand: 1.Audi 2.BMW 3.Benz
2
Select the model: 1.A 2.B 3.C
3
Brand: BMW Model: C Rent: 650
Rent: 3250
Total rent: 135500
```
利用继承和多态知识点为汽车租赁中心开发一个汽车租赁系统,对租赁中心 的汽车进行管理,主要如下: (1)基本功能:首先,输入租赁的天数,用于最后计算租赁的费用;其次, 选择租赁的汽车类型、其次品牌、汽车型号和客车座位数;最后,系统给出分配 的车辆信息和需要支付的租赁费用,以及计算出汽车租赁的总租金,运行效果如 图3; (2)汽车租赁公司出租多种车辆C++
好的,这里是一个基于C++语言的汽车租赁系统设计,使用了继承和多态的知识点:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 汽车基类
class Vehicle {
public:
Vehicle(string plate_num, string brand, string model, double rent) :
plate_num_(plate_num), brand_(brand), model_(model), rent_(rent) {}
virtual ~Vehicle() {}
virtual void printInfo() const = 0; // 纯虚函数,输出车辆信息
double getRent() const { return rent_; } // 获取租金
protected:
string plate_num_; // 车牌号
string brand_; // 品牌
string model_; // 型号
double rent_; // 租金
};
// 小轿车类
class Car : public Vehicle {
public:
Car(string plate_num, string brand, string model, double rent, int seat_num) :
Vehicle(plate_num, brand, model, rent), seat_num_(seat_num) {}
virtual ~Car() {}
virtual void printInfo() const {
cout << "车牌号:" << plate_num_ << " 品牌:" << brand_ << " 型号:" << model_
<< " 座位数:" << seat_num_ << " 租金:" << rent_ << endl;
}
private:
int seat_num_; // 座位数
};
// 客车类
class Bus : public Vehicle {
public:
Bus(string plate_num, string brand, string model, double rent, int seat_num) :
Vehicle(plate_num, brand, model, rent), seat_num_(seat_num) {}
virtual ~Bus() {}
virtual void printInfo() const {
cout << "车牌号:" << plate_num_ << " 品牌:" << brand_ << " 型号:" << model_
<< " 座位数:" << seat_num_ << " 租金:" << rent_ << endl;
}
private:
int seat_num_; // 座位数
};
// 货车类
class Truck : public Vehicle {
public:
Truck(string plate_num, string brand, string model, double rent, double load) :
Vehicle(plate_num, brand, model, rent), load_(load) {}
virtual ~Truck() {}
virtual void printInfo() const {
cout << "车牌号:" << plate_num_ << " 品牌:" << brand_ << " 型号:" << model_
<< " 载重量:" << load_ << " 租金:" << rent_ << endl;
}
private:
double load_; // 载重量
};
// 租赁类
class Rental {
public:
Rental(int days) : days_(days) {}
~Rental() {}
void addVehicle(Vehicle* vehicle) { vehicles_.push_back(vehicle); } // 添加车辆
void printVehicles() const { // 输出所有车辆信息
cout << "当前可租车辆:" << endl;
for (int i = 0; i < vehicles_.size(); ++i) {
cout << i+1 << ". ";
vehicles_[i]->printInfo();
}
}
void rentVehicle(int type, int brand, int model) { // 租车
Vehicle* vehicle = vehicles_[brand-1+model-1];
cout << "您租赁的车辆信息:" << endl;
vehicle->printInfo();
double cost = vehicle->getRent() * days_;
cout << "需要支付的租赁费用:" << cost << endl;
total_cost_ += cost;
}
double getTotalCost() const { return total_cost_; } // 获取总租金
private:
int days_; // 租赁天数
double total_cost_ = 0; // 总租金
vector<Vehicle*> vehicles_; // 所有车辆
};
int main() {
// 初始化所有车辆
Car car1("浙A12345", "奥迪", "A6", 600.0, 5);
Car car2("浙B67890", "宝马", "X5", 800.0, 5);
Bus bus1("浙C11111", "金杯", "海狮", 1200.0, 20);
Bus bus2("浙D22222", "依维柯", "得意", 1500.0, 30);
Truck truck1("浙E33333", "东风", "天龙", 2000.0, 10.0);
Truck truck2("浙F44444", "福田", "欧曼", 2500.0, 20.0);
// 创建租赁类对象
Rental rental(7);
rental.addVehicle(&car1);
rental.addVehicle(&car2);
rental.addVehicle(&bus1);
rental.addVehicle(&bus2);
rental.addVehicle(&truck1);
rental.addVehicle(&truck2);
// 输出所有车辆信息
rental.printVehicles();
// 租车并计算租金
int type, brand, model;
cout << "请输入租赁的车辆类型(1-小轿车 2-客车 3-货车):" << endl;
cin >> type;
cout << "请输入租赁的车辆品牌(1-奥迪 2-宝马 3-金杯 4-依维柯 5-东风 6-福田):" << endl;
cin >> brand;
cout << "请输入租赁的车辆型号(1-A6 2-X5 3-海狮 4-得意 5-天龙 6-欧曼):" << endl;
cin >> model;
rental.rentVehicle(type, brand, model);
// 输出总租金
cout << "总租金:" << rental.getTotalCost() << endl;
return 0;
}
```
在这个程序中,我们定义了一个Vehicle基类和三个派生类(Car、Bus和Truck),用于表示不同类型的车辆。每个派生类都重载了基类中的printInfo()函数,以便输出车辆信息。
我们还定义了一个Rental类,用于管理所有车辆和租赁信息。该类包含一个vector类型的vehicles_成员变量,用于存储所有车辆的指针。它还包含了租赁天数、总租金等成员变量,以及添加车辆、输出所有车辆信息、租车并计算租金等成员函数。
在主函数中,我们首先初始化了所有车辆,并将它们添加到Rental对象中。然后输出所有车辆信息,接着让用户输入租赁的车辆类型、品牌和型号,并调用Rental对象的rentVehicle()函数进行租车和计算租金。最后输出总租金。
这是一个简单的C++汽车租赁系统设计,可以根据具体需求进行修改和扩展。
阅读全文
相关推荐
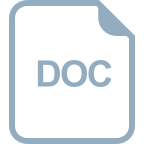


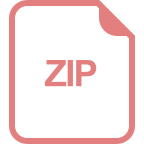
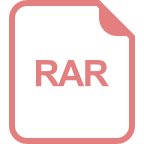
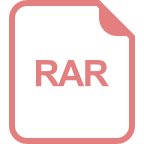
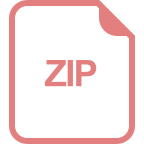
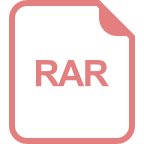
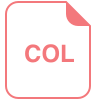


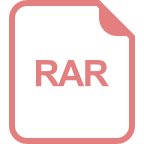
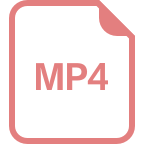