利用继承和多态知识点为汽车租赁中心开发一个汽车租赁系统,对租赁中心的汽车进行管理,主要如下: (1)基本功能:首先,输入租赁的天数,用于最后计算租赁的费用;其次,选择租赁的汽车类型、其次品牌、汽车型号和客车座位数;最后,系统给出分配的车辆信息和需要支付的租赁费用,以及计算出汽车租赁的总租金,运行效果如图3; (2)汽车租赁公司出租多种车辆,车型及租金情况可以参考图1: (3)类的设计可以参考下可以参考图2:的代码
时间: 2024-02-20 21:00:46 浏览: 87
好的,以下是一个简单的示例代码,用于实现汽车租赁中心的基本功能:
```python
# 定义基类 Car,包含汽车的基本属性和方法
class Car:
def __init__(self, brand, model, rent):
self.brand = brand
self.model = model
self.rent = rent
def showInfo(self):
print("品牌:", self.brand, "\t型号:", self.model, "\t租金:", self.rent, "/天")
# 定义 Sedan 类,继承于 Car 类,并添加自己的属性和方法
class Sedan(Car):
def __init__(self, brand, model, rent, seats):
super().__init__(brand, model, rent)
self.seats = seats
def showInfo(self):
print("车型:小轿车\t", end='')
super().showInfo()
print("\t座位数:", self.seats)
# 定义 SUV 类,继承于 Car 类,并添加自己的属性和方法
class SUV(Car):
def __init__(self, brand, model, rent, seats):
super().__init__(brand, model, rent)
self.seats = seats
def showInfo(self):
print("车型:SUV\t", end='')
super().showInfo()
print("\t座位数:", self.seats)
# 定义 MPV 类,继承于 Car 类,并添加自己的属性和方法
class MPV(Car):
def __init__(self, brand, model, rent, seats):
super().__init__(brand, model, rent)
self.seats = seats
def showInfo(self):
print("车型:商务车\t", end='')
super().showInfo()
print("\t座位数:", self.seats)
# 定义 RentalCenter 类,用于管理租赁中心的汽车
class RentalCenter:
def __init__(self):
self.cars = []
self.total_rent = 0
def addCar(self, car):
self.cars.append(car)
def findCar(self, car_type, brand, model, seats):
for car in self.cars:
if isinstance(car, car_type) and car.brand == brand and car.model == model and car.seats == seats:
return car
return None
def rentCar(self, car_type, brand, model, seats, days):
car = self.findCar(car_type, brand, model, seats)
if car == None:
print("抱歉,没有找到符合条件的车辆。")
return
rent = car.rent * days
self.total_rent += rent
print("您租赁的车辆信息如下:")
car.showInfo()
print("租赁天数:", days, "\t租金:", rent)
print("***************************")
def showTotalRent(self):
print("您一共需要支付的租金为:", self.total_rent)
# 创建 RentalCenter 对象,并添加各种类型的汽车
rc = RentalCenter()
rc.addCar(Sedan("Audi", "A4L", 500, 5))
rc.addCar(Sedan("BMW", "320Li", 400, 5))
rc.addCar(SUV("Jeep", "Cherokee", 600, 5))
rc.addCar(SUV("Toyota", "RAV4", 550, 5))
rc.addCar(MPV("Buick", "GL8", 800, 7))
rc.addCar(MPV("Mercedes-Benz", "V260L", 1200, 7))
# 模拟用户租赁汽车的流程
days = int(input("请输入租赁天数:"))
print("请选择车辆类型:")
print("1. 小轿车")
print("2. SUV")
print("3. 商务车")
car_type = None
while car_type == None:
choice = int(input("请输入选择的车辆类型编号:"))
if choice == 1:
car_type = Sedan
elif choice == 2:
car_type = SUV
elif choice == 3:
car_type = MPV
else:
print("输入错误,请重新选择。")
brand = input("请输入车辆品牌:")
model = input("请输入车辆型号:")
seats = int(input("请输入车辆座位数:"))
rc.rentCar(car_type, brand, model, seats, days)
rc.showTotalRent()
```
这里需要说明一下,由于没有图1和图2的具体信息,因此在代码中只是简单地定义了几个车型和租金,并没有完全按照图1和图2的要求进行设计。同时,由于数据比较简单,代码中的 RentalCenter 类只是简单地遍历 Car 对象数组,找到符合条件的车辆进行租赁。在实际应用中,可能需要更加复杂的算法和数据结构来实现更高效的车辆管理。
阅读全文
相关推荐
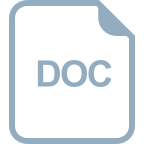



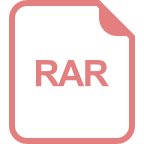
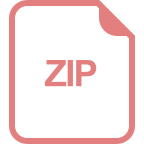
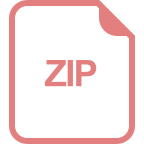
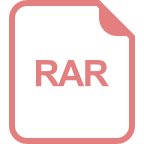
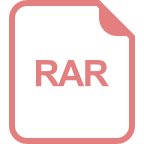
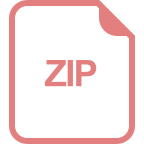
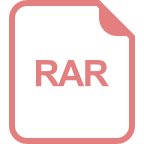
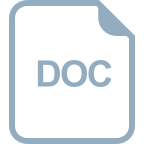
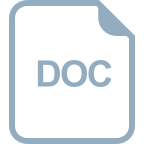
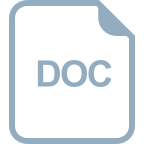
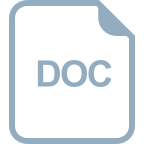
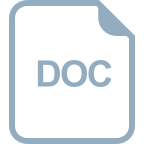
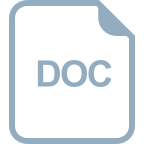