二级残缺棋盘覆盖问题,分治法代码c语言
时间: 2024-06-13 16:01:24 浏览: 21
二级残缺棋盘覆盖问题是指在一个大小为2^n x 2^n的棋盘中,去掉其中一个格子后,如何使用L型骨牌(一种覆盖三个方格的L形图案)将剩下的格子完全覆盖,且每个L型骨牌不重叠、不遗漏地覆盖棋盘中的三个方格。
该问题可以使用分治算法解决。具体思路为:
1. 对于当前的残缺棋盘,找到其中任意一个空白格子,并以该空白格子为中心,将整个棋盘分成四个大小相等的子棋盘,其中包含三个L型骨牌覆盖的方案。
2. 在上述四个子棋盘中,选取其中一个仍然是残缺的子棋盘,重复步骤1,直到所有的空白格子都被L型骨牌覆盖。
以下是C语言的分治算法代码实现,其中board数组代表棋盘,size表示当前棋盘大小,x和y表示当前空白格子的坐标。
```
#include <stdio.h>
#define MAXN 1024
int board[MAXN][MAXN];
int cnt = 0;
void chessboard(int tr, int tc, int dr, int dc, int size, int x, int y) {
if (size == 1) return; // 递归结束条件
int t = ++cnt;
int s = size / 2;
// 当前空白格子在左上角子棋盘中
if (x < tr + s && y < tc + s) {
chessboard(tr, tc, tr + s - 1, tc + s - 1, s, x, y);
} else {
board[tr + s - 1][tc + s - 1] = t;
// 其他三个子棋盘
chessboard(tr, tc, tr + s - 1, tc + s - 1, s, tr + s - 1, tc + s - 1);
chessboard(tr, tc + s, tr + s - 1, tc + s, s, tr + s - 1, tc + s);
chessboard(tr + s, tc, tr + s, tc + s - 1, s, tr + s, tc + s - 1);
chessboard(tr + s, tc + s, dr, dc, s, tr + s, tc + s);
}
}
int main() {
int n = 8; // 棋盘大小为2^n x 2^n
int x = 6; // 空白格子坐标
int y = 5;
board[x][y] = -1;
chessboard(0, 0, n-1, n-1, n, x, y); // 分治算法
for (int i=0; i<n; i++) {
for (int j=0; j<n; j++) {
printf("%3d", board[i][j]);
}
printf("\n");
}
return 0;
}
```
相关推荐
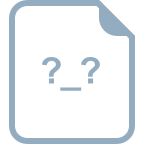














