请用stm32f103zet6单片机生成驱动ws2812代码
时间: 2023-09-19 19:07:14 浏览: 107
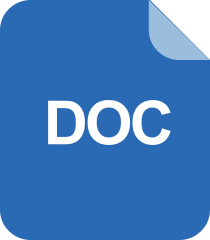
基于STM32F103ZET6单片机的PWM调速、循迹、避障、跟随、红外遥控智能小车设计.doc

当然可以!以下是一个简单的示例代码,用于在 STM32F103ZET6 上驱动 WS2812 LED。
```c
#include "stm32f10x.h"
#include <stdbool.h>
#define LED_PIN GPIO_Pin_13
#define LED_PORT GPIOC
#define NUM_LEDS 8
void ws2812_sendByte(uint8_t byte) {
for (uint8_t i = 0; i < 8; i++) {
if (byte & 0x80) {
GPIO_SetBits(LED_PORT, LED_PIN);
__NOP();
__NOP();
__NOP();
__NOP();
__NOP();
GPIO_ResetBits(LED_PORT, LED_PIN);
__NOP();
__NOP();
} else {
GPIO_SetBits(LED_PORT, LED_PIN);
__NOP();
GPIO_ResetBits(LED_PORT, LED_PIN);
__NOP();
}
byte <<= 1;
}
}
void ws2812_sendRGB(uint8_t r, uint8_t g, uint8_t b) {
ws2812_sendByte(g);
ws2812_sendByte(r);
ws2812_sendByte(b);
}
void ws2812_sendData(uint8_t *data, uint16_t len) {
for (uint16_t i = 0; i < len; i += 3) {
ws2812_sendRGB(data[i], data[i + 1], data[i + 2]);
}
}
int main(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
uint8_t leds[NUM_LEDS * 3] = {0}; // 每个 LED 三个字节(RGB)
while (1) {
// 设置颜色,这里简单演示将所有 LED 设置为红色
for (uint16_t i = 0; i < NUM_LEDS; i++) {
leds[i * 3] = 255; // 红色分量
leds[i * 3 + 1] = 0; // 绿色分量
leds[i * 3 + 2] = 0; // 蓝色分量
}
// 发送数据到 WS2812 LED
ws2812_sendData(leds, NUM_LEDS * 3);
// 延时一段时间
for (uint32_t i = 0; i < 1000000; i++) {
__NOP();
}
}
}
```
这段代码使用了 STM32 的 GPIO 控制 WS2812 LED。其中,`LED_PIN` 和 `LED_PORT` 定义了使用的引脚和端口。`NUM_LEDS` 定义了使用的 LED 数量。
`ws2812_sendByte` 函数用于发送一个字节的数据到 WS2812 LED。它通过位操作控制引脚状态,并进行适当的延时来生成正确的 WS2812 信号。
`ws2812_sendRGB` 函数用于发送一个 RGB 值到 WS2812 LED,它调用 `ws2812_sendByte` 函数发送红色、绿色和蓝色分量。
`ws2812_sendData` 函数用于发送多个 RGB 数据到 WS2812 LED。
在 `main` 函数中,示例将所有 LED 设置为红色,并循环发送数据。
请注意,该示例代码供参考使用,具体的引脚和端口配置可能需要根据实际情况进行修改。
希望能对你有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
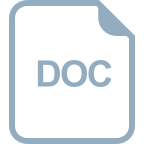
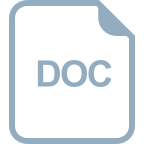

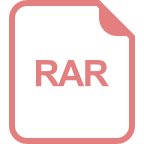
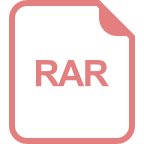
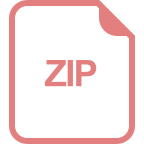
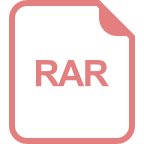
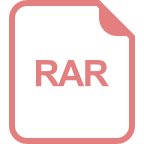
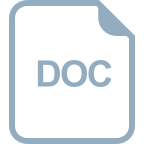
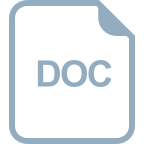
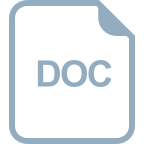
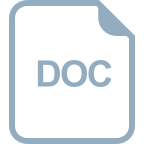




