如何用C语言编写一个函数来检查一个字符串是否为回文?
时间: 2024-12-10 08:29:34 浏览: 15
在C语言中,你可以通过比较字符串的第一个字符和最后一个字符,然后逐个向前移动直到两个指针相遇,同时检查对应位置的字符是否相等来判断一个字符串是否为回文。这里是一个简单的示例函数:
```c
#include <stdio.h>
#include <string.h>
// 定义一个函数,判断字符串是否为回文
int isPalindrome(char str[]) {
int len = strlen(str); // 获取字符串长度
for (int i = 0; i < len / 2; i++) { // 只需要比较一半的字符
if (str[i] != str[len - 1 - i]) { // 如果当前字符和对应反向字符不相等,则不是回文
return 0;
}
}
return 1; // 所有字符都匹配,说明是回文
}
int main() {
char test_str[] = "madam";
if (isPalindrome(test_str)) {
printf("%s 是回文\n", test_str);
} else {
printf("%s 不是回文\n", test_str);
}
return 0;
}
相关问题
如何在C语言中实现一个函数来检查一个字符串是否是回文串?
在C语言中,要实现一个函数来检查一个字符串是否是回文串,你可以创建一个名为`isPalindrome`的函数,它接受一个指向字符数组的指针,并通过比较字符串的第一个和最后一个字符、第二个和倒数第二个字符,直到中间位置,判断它们是否相等。如果整个过程所有对应的字符都匹配,则该字符串是回文。
下面是一个简单的示例:
```c
#include <stdbool.h>
#include <string.h>
// 函数声明
bool isPalindrome(const char* str);
// 实现
bool isPalindrome(const char* str) {
int start = 0;
int end = strlen(str) - 1; // 获取字符串长度
while (start < end) { // 只需比较到字符串中心
if (str[start] != str[end]) {
return false; // 如果任意一对对应字符不相等,立即返回false
}
start++;
end--;
}
return true; // 所有字符匹配,说明是回文
}
int main() {
const char* testStr1 = "madam";
const char* testStr2 = "hello";
printf("%s is a palindrome? %d\n", testStr1, isPalindrome(testStr1));
printf("%s is a palindrome? %d\n", testStr2, isPalindrome(testStr2));
return 0;
}
```
在这个例子中,`isPalindrome`函数会分别检查`testStr1`和`testStr2`,并输出结果。
c语言编写函数判断一个字符串是否为回文运用指针函数并判断输入字符串是否为回文
在C语言中,我们可以利用指针和循环结构来编写一个函数来判断一个字符串是否是回文。回文是指正读反读都一样的字符串,比如"level"、"racecar"等。
下面是一个简单的C函数示例,它接受两个指向字符数组的指针作为参数,通过比较首尾字符是否相等来判断字符串是否为回文:
```c
#include <stdbool.h>
#include <stdio.h>
#include <string.h>
bool is_palindrome(char *str1, char *str2) {
// 如果两个指针交叉点到达结束位置,则说明是回文
if (str1 >= str2)
return true;
// 比较两个指针所指的字符是否相等
while (*str1 == *str2) {
str1++;
str2--;
}
// 如果有不相等的字符,说明不是回文
return false;
}
int main() {
char input[] = "madam";
if (is_palindrome(input, input + strlen(input) - 1)) {
printf("%s is a palindrome.\n", input);
} else {
printf("%s is not a palindrome.\n", input);
}
return 0;
}
```
在这个例子中,`str1` 和 `str2` 分别指向字符串的开始和结束,然后向中心移动,如果发现有不匹配的字符则返回 `false`。如果没有找到不匹配的字符,说明整个字符串是回文,返回 `true`。
阅读全文
相关推荐
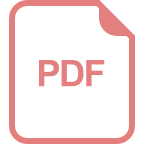
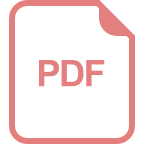
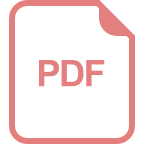













