用C语言设计一个函数判断一个字符串是不是回文字符串
时间: 2024-10-07 18:06:09 浏览: 45
在C语言中,你可以通过设计一个函数来检查一个字符串是否是回文。回文字符串是指正读反读都一样的字符串,例如 "level" 和 "madam" 就是回文字符串。下面是一个简单的C函数示例:
```c
#include <stdio.h>
#include <string.h>
// 函数声明
int isPalindrome(char str []);
// 实现部分
int isPalindrome(char str[]) {
int start = 0;
int end = strlen(str) - 1; // 获取字符串长度
while (start < end) { // 当开始位置小于结束位置
if (str[start] != str[end]) { // 如果对应位置的字符不相等
return 0; // 返回非回文(0)
}
start++; // 移动开始位置
end--; // 移动结束位置
}
return 1; // 所有字符都匹配,返回回文(1)
}
int main() {
char testString[] = "madam";
if (isPalindrome(testString)) {
printf("%s is a palindrome.\n", testString);
} else {
printf("%s is not a palindrome.\n", testString);
}
return 0;
}
```
这个`isPalindrome`函数会逐个比较字符串首尾的字符,直到两端相遇或找到不相等的字符为止。如果整个过程都没有发现不匹配,那么该字符串就是回文。
阅读全文
相关推荐
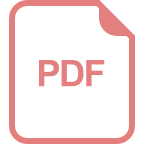
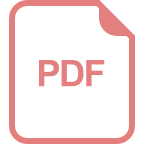
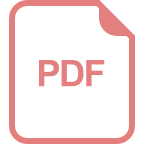















