利用 HTML5 拖放 API知识,创建一个简单网页。网页中有几个不同颜色的方块元素作为可拖放元素,还有几个对应的空白区域作为放置目标。把方块拖放到空白区域,当方块进入目标区域时,方块颜色变亮,拖出目标区域则恢复原色,以此体验拖放交互过程。
时间: 2024-12-30 20:41:47 浏览: 35
HTML5的拖放API允许用户通过鼠标或触摸操作在页面上直接拖动和放置元素。下面是一个简单的例子,展示了如何创建这样一个网页:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<style>
.draggable {
width: 50px;
height: 50px;
background-color: red; /* 可以设置不同颜色 */
border: 1px solid black;
cursor: move;
}
.droppable {
width: 75px;
height: 75px;
display: flex;
justify-content: center;
align-items: center;
background-color: #f0f0f0;
border: 1px dashed grey;
}
.droppable-active {
border-color: green;
}
</style>
</head>
<body>
<!-- 可拖放方块 -->
<div class="draggable" id="drag1">Drag me</div>
<!-- 空白目标区域 -->
<div class="droppable" id="drop1"></div>
<div class="droppable" id="drop2"></div>
<script>
// 获取拖放元素和目标元素
const dragElement = document.getElementById('drag1');
const dropAreas = document.querySelectorAll('.droppable');
// 鼠标悬停事件改变边框颜色
dropAreas.forEach(drop => {
drop.addEventListener('mouseover', () => {
drop.classList.add('droppable-active');
});
drop.addEventListener('mouseout', () => {
drop.classList.remove('droppable-active');
});
});
// 监听拖动元素的mousedown、mousemove和mouseup事件
dragElement.addEventListener('mousedown', dragStart);
dragElement.addEventListener('mousemove', dragMove);
dragElement.addEventListener('mouseup', dragEnd);
function dragStart(e) {
e.dataTransfer.setData('text/html', this.innerHTML); // 设置数据
this.style.backgroundColor = 'yellow'; // 颜色变化
document.body.style.cursor = 'move';
}
function dragMove(e) {
// 计算鼠标相对于文档的位置,并将拖动元素移动到相应位置
const x = e.clientX - this.getBoundingClientRect().left;
const y = e.clientY - this.getBoundingClientRect().top;
this.style.left = x + 'px';
this.style.top = y + 'px';
// 检查是否落入任何一个目标区域内
dropAreas.forEach(drop => {
if (drop.contains(this)) {
drop.classList.add('droppable-active'); // 当进入目标区域时变亮
} else {
drop.classList.remove('droppable-active');
}
});
}
function dragEnd() {
document.body.style.cursor = 'default';
this.style.backgroundColor = 'red'; // 恢复原色
}
</script>
</body>
</html>
```
在这个示例中,我们首先设置了几个方块元素作为可拖放源,和几个空区域作为目标。通过JavaScript监听`mousedown`、`mousemove`和`mouseup`事件,实现了拖放功能。当鼠标移到目标区域内,方块会变亮;离开目标区域则恢复原色。
阅读全文
相关推荐



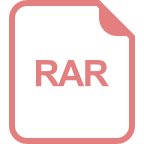
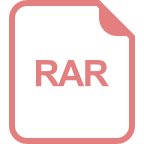
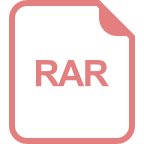








