发生错误TypeError: forward() missing 1 required positional argument: 'x_size'如何解决
时间: 2024-12-21 15:24:29 浏览: 5
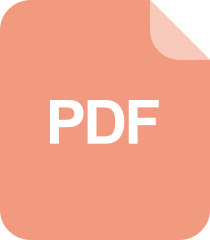
TypeError: missing 1 required keyword-only argument-python中的报错问题

在 `FeedForward` 类的 `forward` 方法中,`dwconv` 需要一个额外的参数 `x_size`。这个参数在调用 `dwconv` 时必须提供。你可以通过以下几种方式来解决这个问题:
### 方案一:修改 `FeedForward` 类的 `forward` 方法
确保在调用 `dwconv` 时传递 `x_size` 参数。例如:
```python
class FeedForward(nn.Module):
def __init__(self, dim, hidden_dim, act_layer=nn.GELU, dropout=0.):
super().__init__()
self.fc1 = nn.Linear(dim, hidden_dim)
self.act = act_layer()
self.before_add = emptyModule()
self.after_add = emptyModule()
self.dwconv = dwconv(hidden_dim=hidden_dim)
self.fc2 = nn.Linear(dim, hidden_dim)
self.dropout = nn.Dropout(dropout)
def forward(self, x, x_size):
x = self.fc1(x)
x = self.act(x)
x = self.before_add(x)
x = x + self.dwconv(x, x_size) # 添加 x_size 参数
x = self.after_add(x)
x = self.dropout(x)
x = self.fc2(x)
x = self.dropout(x)
return x
```
### 方案二:在调用 `FeedForward` 时传递 `x_size`
确保在调用 `FeedForward` 的 `forward` 方法时传递 `x_size` 参数。例如,在 `Transformer` 类的 `forward` 方法中:
```python
class Transformer(nn.Module):
def __init__(self, dim, depth, heads, dim_head, mlp_dim, dropout=0.):
super().__init__()
self.conv8 = nn.Conv2d(in_channels=1, out_channels=1, kernel_size=3, stride=1, padding=1)
self.layers = nn.ModuleList([])
for _ in range(depth):
self.layers.append(nn.ModuleList([
PreNorm(dim, Attention(dim, heads=heads, dim_head=dim_head, dropout=dropout)),
PreNorm(dim, FeedForward(dim, mlp_dim, dropout=dropout))
]))
def forward(self, x):
for attn, ff in self.layers:
b, h, w = x.size()
x7 = x.reshape(b, 1, 7, 64)
x8 = self.conv8(x7)
x = attn(x) + x
x = ff(x, (h, w)) + x # 传递 x_size 参数
x8 = x8.reshape(b, 7, 64)
x = x + x8
return x
```
### 方案三:在 `ViT` 类的 `forward` 方法中传递 `x_size`
确保在调用 `Transformer` 的 `forward` 方法时传递 `x_size` 参数。例如:
```python
class ViT(nn.Module):
def __init__(self, *, image_height, image_width, patch_height, patch_width, num_classes, dim, depth, heads, mlp_dim, channels, pool='mean', dim_head=64, dropout=0., emb_dropout=0.):
super().__init__()
assert image_height % patch_height == 0 and image_width % patch_width == 0, 'Image dimensions must be divisible by the patch size.'
num_patches = (image_height // patch_height) * (image_width // patch_width)
patch_dim = channels * patch_height * patch_width
assert pool in {'cls', 'mean'}, 'pool type must be either cls (cls token) or mean (mean pooling)'
self.to_patch_embedding = nn.Sequential(
Rearrange('b c (h p1) (w p2) -> b (h w) (p1 p2 c)', p1=patch_height, p2=patch_width),
nn.Linear(patch_dim, dim),
)
self.pos_embedding = nn.Parameter(torch.randn(1, num_patches + 1, dim))
self.cls_token = nn.Parameter(torch.randn(1, 1, dim))
self.dropout = nn.Dropout(emb_dropout)
self.transformer = Transformer(dim, depth, heads, dim_head, mlp_dim, dropout=0.)
self.pool = pool
self.to_latent = nn.Identity()
self.mlp_head = nn.Sequential(
nn.LayerNorm(dim),
nn.Linear(dim, num_classes)
)
def forward(self, img):
x = self.to_patch_embedding(img)
b, n, _ = x.shape
cls_tokens = repeat(self.cls_token, '() n d -> b n d', b=b)
x = torch.cat((cls_tokens, x), dim=1)
x += self.pos_embedding[:, :(n + 1)]
x_0 = self.dropout(x)
x_1 = self.transformer(x_0, (int(n**0.5), int(n**0.5))) # 传递 x_size 参数
x_2 = self.transformer(x_1, (int(n**0.5), int(n**0.5)))
diff_1 = x_2 - x_1
diff_1_1 = diff_1 + x_2
x_3 = self.transformer(diff_1_1, (int(n**0.5), int(n**0.5)))
diff_2 = x_3 - x_2
diff_2_2 = diff_2 + x_3
x_4 = self.transformer(diff_2_2, (int(n**0.5), int(n**0.5))) * 0.2
x = x_0 + x_4
x = x.mean(dim=1) if self.pool == 'mean' else x[:, 0]
x = self.to_latent(x)
return self.mlp_head(x)
```
以上三种方案都可以解决 `TypeError: forward() missing 1 required positional argument: 'x_size'` 错误。选择适合你代码结构的一种进行修改即可。
阅读全文
相关推荐
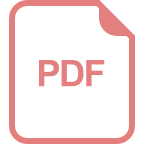
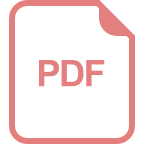















