图书管理系统设计代码
时间: 2024-06-21 19:02:07 浏览: 6
图书管理系统设计代码一般包括前端页面和后端逻辑处理。前端页面包括登录页面、主页面、图书查询页面、图书借阅页面、图书归还页面等,而后端逻辑处理则包括用户验证、图书查询、借阅、归还等功能。
具体实现上,可以采用MVC设计模式,将前端页面与后端逻辑分离。后端采用Java语言,使用Spring框架、Mybatis框架搭建。前端采用HTML、CSS和JavaScript技术,使用jQuery框架来操作DOM。
下面是简单的代码示例:
登录页面:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书管理系统登录</title>
</head>
<body>
<h1>图书管理系统登录</h1>
<form method="post" action="/login">
<label for="username">用户名:</label>
<input type="text" name="username" id="username">
<br>
<label for="password">密码:</label>
<input type="password" name="password" id="password">
<br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
后端逻辑处理:
```
@Controller
public class LoginController {
@Autowired
private UserService userService;
@RequestMapping("/login")
public String login(String username, String password, Model model) {
User user = userService.getUserByUsername(username);
if(user != null && user.getPassword().equals(password)) {
return "redirect:/main";
} else {
model.addAttribute("msg", "用户名或密码错误");
return "login";
}
}
}
```
图书查询页面:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书查询</title>
</head>
<body>
<h1>图书查询</h1>
<form method="post" action="/search">
<label for="name">图书名称:</label>
<input type="text" name="name" id="name">
<input type="submit" value="查询">
</form>
<table>
<tr>
<th>图书编号</th>
<th>图书名称</th>
<th>作者</th>
<th>出版社</th>
<th>借阅状态</th>
<th></th>
</tr>
<c:forEach items="${books}" var="book">
<tr>
<td>${book.id}</td>
<td>${book.name}</td>
<td>${book.author}</td>
<td>${book.press}</td>
<td>${book.status}</td>
<td><a href="/borrow?id=${book.id}">借阅</a></td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
后端逻辑处理:
```
@Controller
public class BookController {
@Autowired
private BookService bookService;
@RequestMapping("/search")
public String search(String name, Model model) {
List<Book> books = bookService.getBooksByName(name);
model.addAttribute("books", books);
return "search";
}
@RequestMapping("/borrow")
public String borrow(int id) {
if(bookService.borrowBook(id)) {
return "borrow_success";
} else {
return "borrow_fail";
}
}
}
```
相关推荐
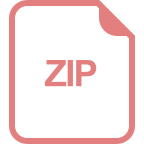
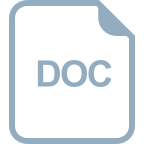
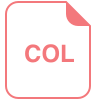
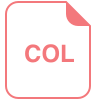
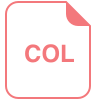
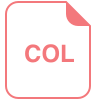
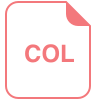









