vue 怎么在父组件的a方法中触发子组件的b方法?
时间: 2024-11-11 07:14:01 浏览: 9
在Vue.js中,为了在父组件的`a`方法中触发子组件的`b`方法,你可以通过`v-on`指令绑定事件监听以及`$emit`方法来实现父子组件间的通信。以下是步骤:
1. 父组件部分,假设`aMethod`是父组件的一个方法:
```html
<template>
<div>
<child-component @myEvent="aMethod"></child-component>
</div>
</template>
<script>
export default {
methods: {
aMethod() {
this.$refs.childComponent.bMethod();
}
},
components: {
ChildComponent: // 子组件标签
}
};
</script>
```
这里,我们使用了`@myEvent`来监听子组件传递的自定义事件`myEvent`。
2. 子组件部分,在`bMethod`方法上添加`emit`:
```html
<template>
<button @click="$emit('myEvent')">触发父组件的b方法</button>
</template>
<script>
export default {
name: 'ChildComponent',
// ...
};
</script>
```
当用户点击子组件内的按钮时,会触发`myEvent`事件,并将其传递给父组件,进而调用父组件的`aMethod`方法。
相关问题
vue 怎么在父组件的方法中触发子组件的b方法?
在Vue.js中,为了在父组件的方法中触发子组件的`b`方法,你可以通过事件监听或者`v-on`指令来实现。这里有两种常见的做法:
1. **使用自定义事件(emit)**:
- 父组件内,创建一个方法并发射一个自定义事件,例如:
```html
<template>
<div>
<child-component @custom-event="handleBMethod"></child-component>
</div>
</template>
<script>
export default {
methods: {
handleBMethod() {
this.$refs.childComponent.bMethod();
}
},
components: { ChildComponent }
};
</script>
```
- 在子组件`ChildComponent`中,定义`bMethod`并触发该事件:
```js
import { Component } from 'vue';
export default class ChildComponent extends Component {
methods: {
bMethod() {
// 执行具体的b方法逻辑
this.$emit('custom-event');
}
}
}
```
2. **使用 prop 和回调函数**:
如果`bMethod`是需要从父组件传递数据给子组件的情况,可以使用prop将一个函数作为参数传入子组件,然后在子组件内部调用这个函数:
```html
<template>
<child-component :call-b-method="callBMethod"></child-component>
</template>
<script>
export default {
methods: {
callBMethod() {
// 在这里执行其他操作,然后调用子组件的bMethod
this.$refs.childComponent.bMethod();
}
},
components: { ChildComponent }
};
</script>
```
子组件接收`call-b-method`属性,并在其需要的时候执行:
```js
export default {
props: ['callBMethod'],
methods: {
bMethod() {
this.callBMethod();
}
}
}
```
vue在子组件中触发父组件的方法
在Vue中,子组件可以通过`$emit`方法来触发父组件中的自定义事件,从而实现调用父组件的方法。具体步骤如下:
1. 在父组件中定义一个方法,用于处理子组件触发的自定义事件。
```javascript
methods: {
handleCustomEvent() {
// 处理逻辑
}
}
```
2. 在父组件中使用子组件,并绑定自定义事件。
```html
<template>
<div>
<ChildComponent @customEvent="handleCustomEvent"></ChildComponent>
</div>
</template>
```
3. 在子组件中通过`$emit`方法触发自定义事件。
```javascript
this.$emit('customEvent');
```
当子组件执行`this.$emit('customEvent')`时,就会触发父组件中的`handleCustomEvent`方法。注意,自定义事件的名称需要与父组件中绑定的事件名称一致。
阅读全文
相关推荐
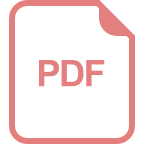
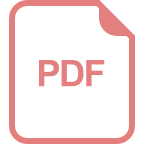
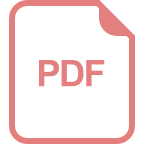













