用Python画一只HelloKitty
时间: 2024-10-23 07:05:28 浏览: 88
要使用Python来画一只简单的Hello Kitty,我们可以借助PIL(Pillow)库,这是一个用于处理图像的强大库。以下是一个简化的Hello Kitty形状示例,我们不会绘制非常精细的细节,但能提供基本的概念:
```python
from PIL import Image, ImageDraw
# 定义Hello Kitty的基本形状
def draw_hello_kitty(width=80, height=60):
img = Image.new('RGB', (width, height), color='white')
draw = ImageDraw.Draw(img)
# Hello Kitty的眼睛
eye_size = 10
eye_color = 'black'
left_eye_pos = (width // 4, height // 2 - eye_size)
right_eye_pos = (width * 3 // 4, height // 2 - eye_size)
draw.ellipse(left_eye_pos + (eye_size, eye_size), fill=eye_color)
draw.ellipse(right_eye_pos + (eye_size, eye_size), fill=eye_color)
# 嘴巴
mouth_width = 5
mouth_height = 10
mouth_left = width // 2 - mouth_width // 2
mouth_top = height // 2 + mouth_height // 2
mouth_right = mouth_left + mouth_width
mouth_bottom = mouth_top - mouth_height
draw.rectangle((mouth_left, mouth_top, mouth_right, mouth_bottom), outline=eye_color)
# 耳朵
ear_size = 15
ear_offset = ear_size / 2
ear_left = width // 4 - ear_offset
ear_right = width // 4 + ear_offset
ear_top = height // 4 - ear_offset
ear_bot = height // 4 + ear_offset
draw.polygon([(ear_left, ear_top), (ear_right, ear_top), (ear_right, ear_bot)], fill='black')
return img
# 创建并显示Hello Kitty
hello_kitty = draw_hello_kitty()
hello_kitty.show()
```
运行这段代码后,你会看到一个简单的Hello Kitty图形。请注意,这只是一个基础版本,如果你想让它更像真正的Hello Kitty,可能需要添加更多的细节,如身体、头发等。
阅读全文
相关推荐
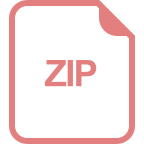
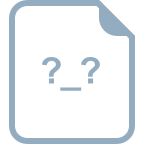
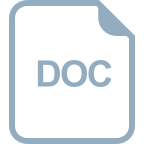

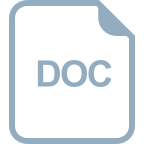

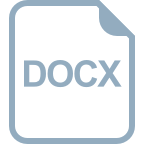
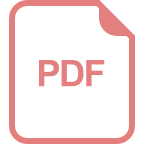
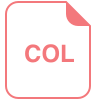
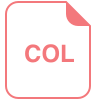
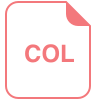
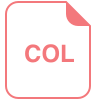
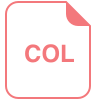
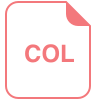
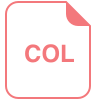
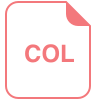
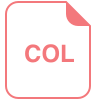