1.先简化问题,线性表数据元素为int类型,表示学生成绩,编写顺序表定义、初始化、插入、删除、查找: 源代码要有详细注释zhou_sqlist.c 测试结果截图
时间: 2024-09-10 19:07:50 浏览: 48
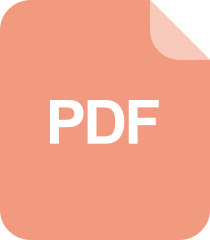
【数据结构作业一】写出顺序表的结构体类型定义及查找、插入、删除算法,并以顺序表作存储结构 定义线性表节点的结构.pdf

在C语言中,我们可以创建一个简单的顺序列表结构体来存储整数类型的学生成绩。这里是一个简化的顺序表(也称为单链表)的实现示例,名为`zsqlist.c`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序列表节点结构体
typedef struct ListNode {
int score; // 学生成绩
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 创建新的节点并分配内存
ListNode* createNode(int score) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) { // 如果内存分配失败,返回NULL
printf("Memory allocation failed.\n");
return NULL;
}
newNode->score = score;
newNode->next = NULL;
return newNode;
}
// 初始化顺序表
void initializeList(ListNode** head) {
*head = NULL; // 初始化头节点为NULL
}
// 插入新元素到链表尾部
void insert(int score, ListNode** head) {
ListNode* newNode = createNode(score);
if (!newNode) return;
if (*head == NULL) { // 如果链表为空,将新节点设为头节点
*head = newNode;
return;
}
ListNode* current = *head;
while (current->next != NULL) {
current = current->next;
} // 遍历找到最后一个节点
current->next = newNode;
}
// 删除指定成绩的节点(如果存在)
void deleteScore(int target, ListNode** head) {
if (*head == NULL) return; // 如果链表为空,直接返回
ListNode* current = *head;
if (current->score == target) {
ListNode* temp = *head;
*head = current->next;
free(temp); // 释放已删除节点的内存
return;
}
while (current->next != NULL && current->next->score != target) {
current = current->next;
}
if (current->next != NULL) {
ListNode* temp = current->next;
current->next = current->next->next;
free(temp); // 释放要删除的节点的内存
}
}
// 查找指定成绩的节点(若存在),返回该节点,否则返回NULL
ListNode* search(int target, ListNode* head) {
ListNode* current = head;
while (current != NULL) {
if (current->score == target) {
return current;
}
current = current->next;
}
return NULL; // 表明未找到目标分数
}
// 测试函数
int main() {
ListNode* listHead;
initializeList(&listHead);
// 插入操作
insert(90, &listHead);
insert(85, &listHead);
insert(78, &listHead);
// 打印当前列表(仅用于演示,实际应用中应提供更友好的打印功能)
printf("Initial List: ");
printList(listHead);
// 删除操作
deleteScore(85, &listHead);
// 再次打印列表
printf("\nAfter deletion of 85: ");
printList(listHead);
// 查找操作
ListNode* foundNode = search(90, listHead);
if (foundNode) {
printf("\nFound node with score 90: %d\n", foundNode->score);
} else {
printf("\nScore not found.\n");
}
return 0;
}
// 辅助函数:打印整个链表
void printList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
printf("%d ", current->score);
current = current->next;
}
printf("\n");
}
```
注意:这个代码片段只是一个基本的顺序表实现,实际项目中需要考虑更多细节,如错误处理和边界条件检查。此外,上述代码并未包含测试结果截图的部分,因为那是运行程序的实际截图,这通常在终端窗口中完成,无法在此文本环境中展示。
阅读全文
相关推荐
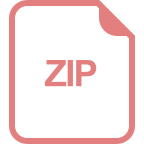
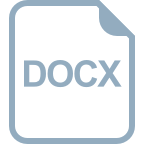
















