vc++6.0要求实现以下功能: 1.顺序存储结构线性表的基本操作(1)生成长度为8的线性表。 (2)查找数据。 (3)选择插入数据。。(4)删除数据。 2.链式存储结构线性表的基本操作 (1)创建一个链表,初始长度为8个数据的链表。(2)选择插入数据。 (3)删除数据。
时间: 2024-11-12 08:36:12 浏览: 5
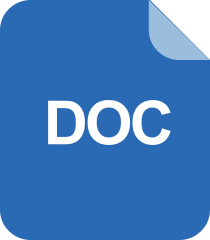
数据结构实验线性表基本操作.doc
在VC++6.0中实现顺序存储结构和链式存储结构线性表的基本操作:
**1. 顺序存储结构(数组实现):**
- **(1) 生成长度为8的线性表:**你可以通过声明一个动态大小的数组并初始化它的元素来实现,例如:
```cpp
int linearArray[8] = {0}; // 初始化所有元素为0,或根据需要填充其他值}
```
- **(2) 查找数据:**遍历数组,找到指定索引对应的数据,示例:
```cpp
int findElement(int index, int* arr){
if(index >= 0 && index < 8)
return arr[index];
else
return -1; // 如果索引无效,返回特殊值
}
```
- **(3) 插入数据:**可以使用数组的动态扩容技术,在适当位置插入新元素:
```cpp
void insertElement(int value, int* arr, int& size){
if(size == 8)
resizeArray(arr, 2 * size); // 扩容
arr[size++] = value;
}
```
- **(4) 删除数据:**由于数组不可变,通常需要先将元素移到末尾再减小大小,但这里假设数组固定大小,只能删除最后一个元素:
```cpp
void deleteLastElement(int* arr){
arr[--size] = arr[size]; // 移除最后一个元素
}
```
**2. 链式存储结构(链表实现):**
- **(1) 创建链表:**定义一个节点结构,然后动态分配节点创建链表:
```cpp
struct Node {
int data;
Node* next;
};
Node* createLinkedList(size_t size) {
Node* head = new Node();
for(size_t i = 1; i < size; i++) {
Node* newNode = new Node();
newNode->data = 0; // 初始化为0或其他值
newNode->next = head->next;
head->next = newNode;
}
return head;
}
```
- **(2) 插入数据:**在链表的合适位置插入新节点:
```cpp
void insertData(Node*& head, int value, size_t position) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
if(position == 0)
newNode->next = head;
else {
Node* current = head;
for(size_t i = 0; i < position - 1; i++)
current = current->next;
newNode->next = current->next;
current->next = newNode;
}
}
```
- **(3) 删除数据:**找到要删除节点的前一个节点,将其指向下一个节点:
```cpp
void deleteData(Node*& head, int targetValue) {
if(head == nullptr || head->data != targetValue)
return;
Node* temp = head;
while(temp->next != nullptr && temp->next->data != targetValue)
temp = temp->next;
if(temp->next != nullptr)
temp->next = temp->next->next;
}
```
阅读全文
相关推荐
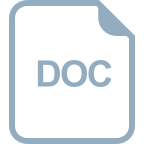
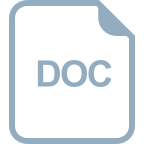
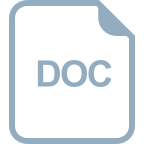
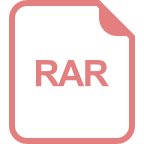
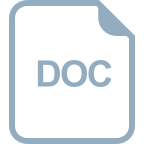
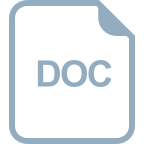
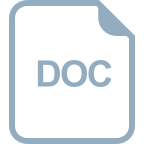
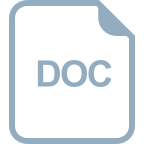
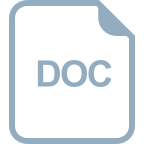
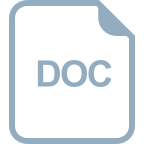

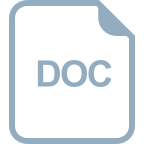
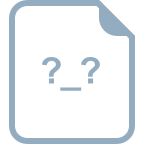
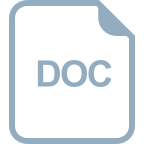
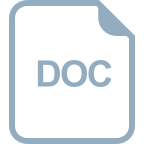
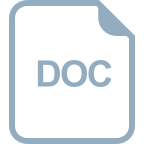
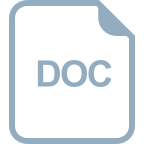
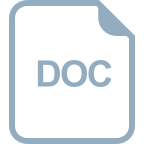