用汇编语言实现C=A÷B。设A为3字节未组合BCD整数,B为1字节未组合BCD整数,C为3字节未组合BCD整数,三个数均为存储器数。
时间: 2024-10-29 11:24:54 浏览: 28
在汇编语言中,实现将A除以B并把结果存入C这样的算术操作,需要考虑如何处理BCD(二进制编码的十进制)数的运算。由于BCD表示法每个数字有4位,我们需要对每一位分别进行处理。以下是一个简单的示例,假设x86架构,使用MIPS汇编语言:
```assembly
.data
A: .word 0xABCDEF ; 存储3字节BCD数 A
B: .byte 0x07 ; 存储1字节BCD数 B
C: .word 0x0000 ; 存储3字节BCD数 C
.text
.globl main
main:
# Load the values from memory
lw $t0, A ; $t0 = A (低字)
lw $t1, A+2 ; $t1 = A (高字)
lb $t2, B ; $t2 = B
# Divide each digit of A by B
divu $t0, $t2 ; $t0 = floor($t0 / B), HI
mfhi $t3 ; Save high part in C
sll $t3, 1 ; Shift left for place value
sw $t3, C ; Store the high part of quotient
addiu $t0, $t0, -1 ; Adjust for low word
bdnz continue_div ; Skip if not zero
# If there's no remainder, store a zero
li $t3, 0
sw $t3, C+2
continue_div:
# Divide the remaining digits and update C
lw $t4, C+2 ; Load low part of C
add $t4, $t4, $t0 ; Add the high part to low part
divu $t4, $t2 ; $t4 = floor($t4 / B), HI
mfhi $t5 ; Save high part
sll $t5, 1
sw $t5, C+2 ; Update C with new quotient
# Check if there's still a remainder
bltz $t0, end_div ; Jump out if there's no more digits
# Handle the last digit as a special case
xor $t6, $t6, $t0 ; Create mask for the last bit
and $t0, $t0, $t6 ; Get just the last digit of A
divu $t0, $t2 ; $t0 = last digit / B
mfhi $t7 ; Save the result
or $t7, $t7, $t4 ; Combine with previous quotient
sw $t7, C+2 ; Store the final quotient
end_div:
jr $ra ; Return from main
```
这只是一个基本示例,实际的BCD除法可能涉及更复杂的算法来处理进位。注意,这个代码并不是完整的程序,你需要将其放入一个完整项目并链接适当的库以运行。
阅读全文
相关推荐
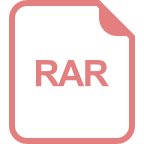
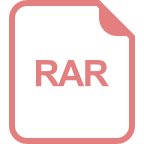
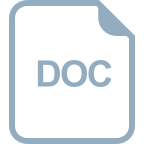
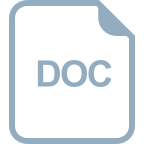
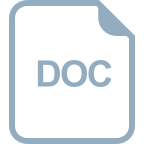
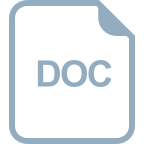


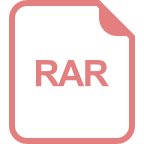
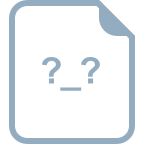
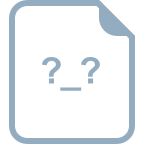





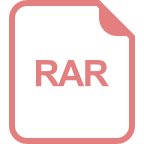
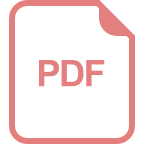
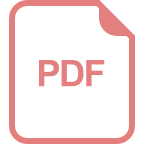