c#定时器与多线程的使用
时间: 2023-09-05 22:11:46 浏览: 145
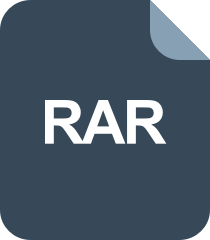
c#定时器+多线程

C#中定时器和多线程是常用的并发编程工具。定时器用于在指定的时间间隔内执行某个任务,而多线程则允许同时执行多个任务。
在C#中,可以使用Timer类来创建定时器。Timer类有两种类型:System.Timers.Timer和System.Threading.Timer。System.Timers.Timer是基于事件的定时器,适用于Windows Forms和WPF应用程序。System.Threading.Timer是基于线程池的定时器,适用于所有类型的应用程序。
下面是一个使用System.Timers.Timer的示例:
```csharp
using System;
using System.Timers;
class Program
{
static void Main()
{
Timer timer = new Timer(1000); // 创建一个1秒的定时器
timer.Elapsed += TimerElapsed; // 绑定定时器事件
timer.Start(); // 启动定时器
Console.WriteLine("按任意键停止定时器...");
Console.ReadKey();
timer.Stop(); // 停止定时器
}
static void TimerElapsed(object sender, ElapsedEventArgs e)
{
Console.WriteLine("定时器触发,当前时间:{0}", e.SignalTime);
}
}
```
多线程在C#中使用Thread类来创建和管理线程。以下是一个使用多线程的示例:
```csharp
using System;
using System.Threading;
class Program
{
static void Main()
{
Thread thread = new Thread(DoWork); // 创建一个新线程
thread.Start(); // 启动线程
Console.WriteLine("按任意键停止线程...");
Console.ReadKey();
thread.Abort(); // 终止线程
}
static void DoWork()
{
while (true)
{
Console.WriteLine("线程执行中...");
Thread.Sleep(1000); // 暂停1秒
}
}
}
```
以上是使用定时器和多线程的基本示例,你可以根据自己的需求进行定制和扩展。请注意,在使用多线程时要小心处理线程安全性和资源共享的问题。
阅读全文
相关推荐
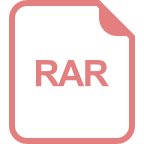
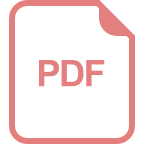
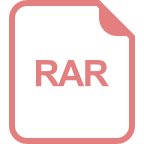
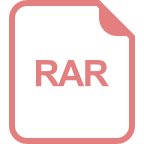
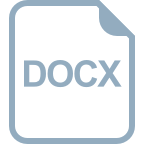
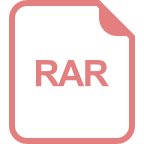
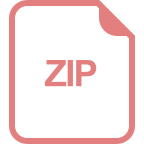
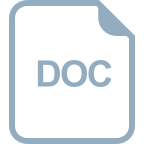
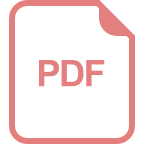
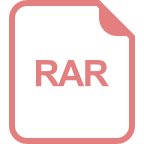
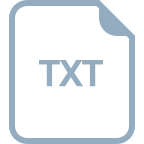
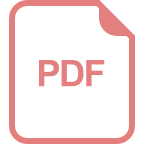
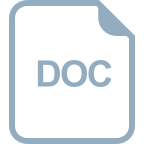
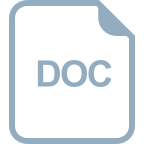
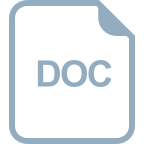

