int CR_RegisterEventCallback (int nDetrIdx, ICallback∗ pCallback ) ; class CCallbackImp : public ICallback { public: virtual void Process (int nEventID, CR Event∗ pEvent); void SetFrmBuf(char∗ pFrmBuf); void SetFrmHeaderLen(int nLen); private : int m nFrmHeaderLen; // In bytes char∗ m pFrmBuf; }; void CCallbackImp::Process(int nEventID, CR Event∗ pEvent) { if (CR EVT NEW FRAME == nEventID) { int nFrmIdxInBuf = ∗(int∗)pEvent−>pData; int nFrmSize = m nFrmHeaderLen + pEvent−>nPixelDepth ∗ pEvent−>nWidth ∗ pEvent−>nHeight / 8; if (m pFrmBuf != NULL) { char∗ pCurrFrm = (char∗)m pFrmBuf + nFrmIdxInBuf ∗ nFrmSize; memcpy(pDst, pCurrFrm, nFrmSize); } } } void CCallbackImp::SetFrmBuf(char∗ pFrmBuf) { m pFrmBuf = pFrmBuf; } void CCallbackImp::SetFrmHeaderLen(int nLen) { m nFrmHeaderLen = nLen; } enum CR_EventID { CR EVT SERVER DISCONNECTED, // dropped connection with server CR EVT DETR DISCONNECTED, // dropped connection with detector CR EVT TEMPERATURE INFO, // temperature of the detector CR EVT NEW FRAME, // Arrival of a new frame CR EVT CALIBRATION IN PROGRESS, // Calibration in progress CR EVT CALIBRATION FINISHED, // Completion of calibration CR EVT ACQ STAT INFO // Acquisition of statistical summary }; struct CR_Event { int nDetrIdx; int nWidth; // Same as CR ModeInfo.nImageWidth, see A.4 int nHeight; int nPixelDepth; void∗ pData; }; struct CR_AcquisitionStatInfo { int nTotalFrameNum; // Total number of frames acquired int nLostFrameNum; // Number of lost frames float fStatFrameRate; float fTransmissionSpeed; long long nAcqDuration; // Duration of image acquisition } python调用
时间: 2024-04-15 14:30:10 浏览: 189
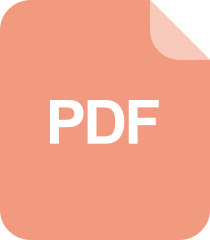
59丨模板模式(下):模板模式与Callback回调函数有何区别和联系?1
要在Python中调用C语言函数并创建CCallbackImp类的实例对象,你可以使用ctypes库。以下是一个示例:
```python
import ctypes
import struct
# 定义回调函数类型
CallbackFuncType = ctypes.CFUNCTYPE(None, ctypes.c_int, ctypes.POINTER(ctypes.c_void_p))
# 定义结构体
class CR_Event(ctypes.Structure):
_fields_ = [
("nDetrIdx", ctypes.c_int),
("nWidth", ctypes.c_int),
("nHeight", ctypes.c_int),
("nPixelDepth", ctypes.c_int),
("pData", ctypes.c_void_p),
]
class CR_AcquisitionStatInfo(ctypes.Structure):
_fields_ = [
("nTotalFrameNum", ctypes.c_int),
("nLostFrameNum", ctypes.c_int),
("fStatFrameRate", ctypes.c_float),
("fTransmissionSpeed", ctypes.c_float),
("nAcqDuration", ctypes.c_longlong),
]
# 加载共享库
lib = ctypes.CDLL("your_library.so") # 替换为你的共享库文件路径
# 定义函数参数和返回类型
lib.CR_RegisterEventCallback.argtypes = [ctypes.c_int, CallbackFuncType]
lib.CR_RegisterEventCallback.restype = ctypes.c_int
# 定义回调函数
def callback_func(nEventID, pEvent):
event = ctypes.cast(pEvent, ctypes.POINTER(CR_Event)).contents
if nEventID == 3:
# 处理 CR_EVT_TEMPERATURE_INFO 事件
temperature = struct.unpack("f", ctypes.string_at(event.pData, 4))[0]
print("Temperature:", temperature)
elif nEventID == 4:
# 处理 CR_EVT_NEW_FRAME 事件
nFrmIdxInBuf = ctypes.cast(event.pData, ctypes.POINTER(ctypes.c_int)).contents.value
nFrmSize = callback_obj.nFrmHeaderLen + event.nPixelDepth * event.nWidth * event.nHeight // 8
if callback_obj.pFrmBuf is not None:
pCurrFrm = ctypes.cast(callback_obj.pFrmBuf, ctypes.POINTER(ctypes.c_char))
pCurrFrm += nFrmIdxInBuf * nFrmSize
# 处理新帧数据
# ...
# 创建回调函数
callback_func_type = CallbackFuncType(callback_func)
# 定义CCallbackImp类
class CCallbackImp(ctypes.Structure):
_fields_ = [
("pCallback", ctypes.c_void_p),
("nFrmHeaderLen", ctypes.c_int),
("pFrmBuf", ctypes.c_char_p),
]
def __init__(self):
self.pCallback = ctypes.cast(callback_func_type, ctypes.c_void_p)
self.nFrmHeaderLen = 0
self.pFrmBuf = None
# 封装为Python函数
def CR_RegisterEventCallback(nDetrIdx):
callback_obj = CCallbackImp()
result = lib.CR_RegisterEventCallback(nDetrIdx, ctypes.byref(callback_obj))
# 处理返回值
return result
# 调用封装的Python函数
nDetrIdx = 123 # 替换为你的参数值
result = CR_RegisterEventCallback(nDetrIdx)
# 处理返回值
print("CR_RegisterEventCallback result:", result)
```
请将 `your_library.so` 替换为你实际的共享库文件路径,并根据你的情况修改回调函数中的事件处理逻辑。
这样,你就可以在Python中通过调用封装的CR_RegisterEventCallback函数来调用C语言的CR_RegisterEventCallback函数了,同时创建了CCallbackImp类的实例对象,并在回调函数中处理不同的事件类型。注意要根据事件类型解析pData中的数据。
阅读全文
相关推荐
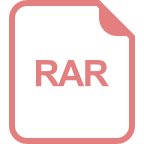
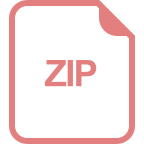






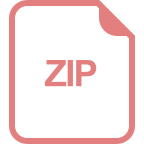
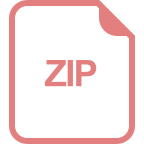
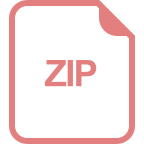