vue3 setup使用store的数据但是不要更新
时间: 2024-12-10 19:49:53 浏览: 12
在Vue 3中,`setup` 函数是用来声明式地设置组件内部状态的,而Vuex store是管理全局应用状态的好地方。如果你只想在 `setup` 中读取store的数据,但不想让store的状态因为这个组件的变化而更新,你可以通过`reactive`或`ref`函数来创建响应式的局部变量,并将其绑定到store的相应数据。
例如:
```javascript
import { ref, onMounted } from 'vue';
import { useStore } from '@/store';
const store = useStore();
const myData = ref(store.state.myData); // 创建只读的ref
onMounted(() => {
// 初始化时获取store的数据
console.log(myData.value);
});
// 在setup中使用myData,不会触发store的更新
myData.value.someProperty; // 读取数据
```
这里,`myData`是一个只读的响应式值,它会直接从store中获取初始值,但在 `setup` 中对它的操作并不会自动同步回store,所以实现了你想要的效果。
相关问题
vue3如何使用store
Vue3中使用Vuex作为状态管理库时,Store是用来集中存储应用全局状态的地方。以下是设置和使用Vuex store的基本步骤:
1. 安装依赖:首先确保已经安装了`vue-class-component`和`vuex`,如果还没有,可以运行`npm install vue-class-component vuex`。
2. 创建store:创建一个名为store.js的新文件,并导入`createStore`函数,它是Vuex的核心函数。例如:
```javascript
import { createStore } from 'vuex'
export default createStore({
state: {
count: 0,
},
mutations: {
increment(state) {
state.count++
},
decrement(state) {
state.count--
},
},
actions: {
increment(context) {
context.commit('increment')
},
decrement(context) {
context.commit('decrement')
},
},
})
```
3. 在组件中引用store:在需要使用store的地方,通过`setup`钩子注入store实例:
```vue
<template>
<div>
<button @click="increment">+1</button>
<button @click="decrement">-1</button>
<p>Count: {{ count }}</p>
</div>
</template>
<script lang="ts">
import { ref, onMounted, computed, defineComponent } from 'vue'
import store from './store'
export default defineComponent({
setup() {
const count = ref(store.state.count)
onMounted(() => {
// 当store的状态改变时,会自动更新count
watch(store.state.count, (newVal) => (count.value = newVal))
})
const increment = () => store.dispatch('increment')
const decrement = () => store.dispatch('decrement')
return {
count,
increment,
decrement
}
}
})
</script>
```
4. 使用actions:当你想在组件之外的地方触发state的变化时,你可以使用actions,它们通常包含异步操作。
记得在项目结构中,store应该位于项目的`src/store`目录下,而组件应尽量避免直接修改store的状态,保持“纯粹”的视图层。
vue3 setup中使用mapActions
在 Vue3 的 setup 函数中,可以通过 `useStore` 函数获取 Vuex 的 store 对象,然后通过 `mapActions` 函数将 actions 映射到当前组件的作用域内,以便在模板中调用。
以下是一个示例:
```javascript
import { useStore, mapActions } from 'vuex'
export default {
setup() {
const store = useStore()
const { myAction } = mapActions(['myAction'])
// 在组件中调用 myAction
myAction()
// ...
}
}
```
需要注意的是,`mapActions` 函数接收一个数组作为参数,数组中包含要映射的 actions 名称。在这个示例中,我们只映射了单个 action,也可以同时映射多个 action,例如:
```javascript
const { myAction1, myAction2 } = mapActions(['myAction1', 'myAction2'])
```
阅读全文
相关推荐
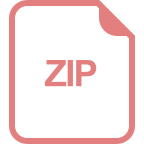
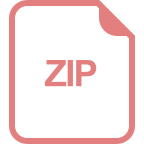
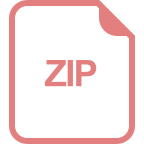













