vue3 setup中使用mapActions
时间: 2024-04-07 11:10:29 浏览: 174
在 Vue3 的 setup 函数中,可以通过 `useStore` 函数获取 Vuex 的 store 对象,然后通过 `mapActions` 函数将 actions 映射到当前组件的作用域内,以便在模板中调用。
以下是一个示例:
```javascript
import { useStore, mapActions } from 'vuex'
export default {
setup() {
const store = useStore()
const { myAction } = mapActions(['myAction'])
// 在组件中调用 myAction
myAction()
// ...
}
}
```
需要注意的是,`mapActions` 函数接收一个数组作为参数,数组中包含要映射的 actions 名称。在这个示例中,我们只映射了单个 action,也可以同时映射多个 action,例如:
```javascript
const { myAction1, myAction2 } = mapActions(['myAction1', 'myAction2'])
```
相关问题
vue3里如何使用mapActions
在 Vue3 中,使用 `mapActions` 可以通过 `import` 直接引入,无需再从 `vuex` 实例中引入。具体步骤如下:
1. 在组件中引入 `mapActions` 方法:
```javascript
import { mapActions } from 'vuex';
```
2. 在 `setup` 函数中使用 `mapActions` 方法:
```javascript
setup() {
const { someAction } = mapActions(['someAction']);
return {
someAction,
};
}
```
3. 在组件中使用 `someAction` 方法:
```javascript
methods: {
handleClick() {
this.someAction();
},
},
```
这样就可以在 Vue3 中使用 `mapActions` 方法了。
vue3setup方式怎么用vuex存储教师信息,并对教师的薪水进行修改,对教师进行增删
在Vue3中使用Vuex需要先安装Vuex:
```
npm install vuex@next --save
```
然后,在Vue项目的入口文件`main.js`中引入Vuex并创建一个store:
```javascript
import { createStore } from 'vuex'
const store = createStore({
state() {
return {
teachers: []
}
},
mutations: {
addTeacher(state, teacher) {
state.teachers.push(teacher)
},
deleteTeacher(state, index) {
state.teachers.splice(index, 1)
},
updateTeacherSalary(state, { index, salary }) {
state.teachers[index].salary = salary
}
},
actions: {
addTeacher({ commit }, teacher) {
commit('addTeacher', teacher)
},
deleteTeacher({ commit }, index) {
commit('deleteTeacher', index)
},
updateTeacherSalary({ commit }, { index, salary }) {
commit('updateTeacherSalary', { index, salary })
}
}
})
```
在这个例子中,我们定义了一个状态`teachers`,它是一个教师列表。我们还定义了三个mutation:`addTeacher`用于增加教师,`deleteTeacher`用于删除教师,`updateTeacherSalary`用于修改教师的薪水。我们还定义了三个action,它们分别调用对应的mutation。
对于增加教师、删除教师和修改教师的薪水,我们可以在组件中使用以下代码来分别调用对应的action:
```javascript
<template>
<div>
<ul>
<li v-for="(teacher, index) in teachers" :key="index">
<span>{{ teacher.name }}</span>
<span>{{ teacher.salary }}</span>
<button @click="updateSalary(index, teacher.salary + 1000)">增加薪资</button>
<button @click="deleteTeacher(index)">删除</button>
</li>
</ul>
<form @submit.prevent="addTeacher">
<input type="text" v-model="newTeacher.name">
<input type="number" v-model="newTeacher.salary">
<button type="submit">添加</button>
</form>
</div>
</template>
<script>
import { mapState, mapActions } from 'vuex'
export default {
computed: {
...mapState(['teachers'])
},
methods: {
...mapActions(['addTeacher', 'deleteTeacher', 'updateTeacherSalary']),
updateSalary(index, salary) {
this.updateTeacherSalary({ index, salary })
},
deleteTeacher(index) {
this.deleteTeacher(index)
},
addTeacher() {
this.addTeacher(this.newTeacher)
this.newTeacher = { name: '', salary: 0 }
}
},
data() {
return {
newTeacher: { name: '', salary: 0 }
}
}
}
</script>
```
在这个例子中,我们使用了`mapState`和`mapActions`函数来简化代码。`mapState`函数将state映射到组件的计算属性中,`mapActions`函数将action映射到组件的methods中。在模板中,我们使用`v-for`指令遍历教师列表,并使用`v-model`指令实现双向绑定。在修改教师薪水和删除教师时,我们调用对应的action。在添加教师时,我们调用`addTeacher`action,并清空表单。
阅读全文
相关推荐
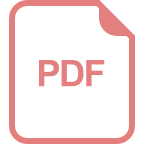
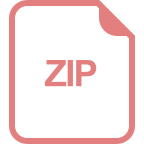
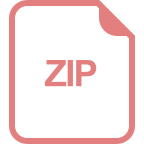
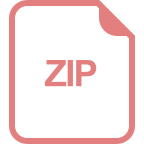
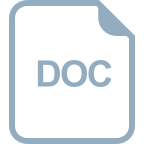
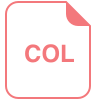










