vue3不同页面间传值
时间: 2024-08-09 19:01:35 浏览: 172
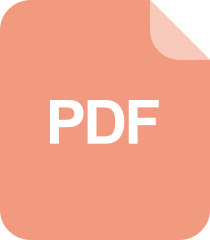
在vue中实现简单页面逆传值的方法
Vue3的不同页面之间传递数据通常涉及使用 Vue Router 进行路由管理,并利用 Vuex 或者 Composition API 的上下文提供全局状态管理。下面是这两种方法的具体说明:
### 使用 Vuex
**原理及步骤**:
1. **安装 Vuex**: 首先需要安装 Vuex,通过 `npm install vuex` 或 `yarn add vuex` 来安装。
2. **创建 Vuex Store**: 创建 Vuex store 文件(例如 `store/index.js`),并在其中定义 state、mutations、actions 和 getters。
```javascript
import { createStore } from 'vuex';
export default createStore({
state: {
sharedData: '',
},
mutations: {
setSharedData(state, payload) {
state.sharedData = payload;
},
},
actions: {
updateSharedData({ commit }, payload) {
commit('setSharedData', payload);
},
},
getters: {
getSharedData: (state) => state.sharedData,
},
});
```
3. **配置路由并挂载 Vuex**: 在 `router/index.js` 中引入 Vuex 实例并将其作为全局服务使用。
```javascript
import Vue from 'vue';
import Vuex from 'vuex';
import router from './router';
// 其他导入...
Vue.use(Vuex);
const store = new Vuex.Store({
// Vuex store 定义...
});
new Vue({
router,
store,
render: (h) => h(App),
}).$mount('#app');
```
4. **在组件间传递数据**:
- 在接收数据的组件中,可以监听 Vuex store 的变化,或者直接访问 store 中的数据。
- 在更新数据的组件中,使用 `this.$store.dispatch` 调用 action 来触发 Vuex 中的操作,然后由 action 触发 mutation 来修改 state。
5. **使用 computed 或者 methods 访问数据**: 可以在组件内使用 Vuex store 的 getters 获取共享数据。
### 使用 Composition API
**原理及步骤**:
1. **引入 Composables**:
引入 Vuex 依赖项 `useStore` 以及 `defineStore` 函数来简化状态管理。
2. **创建 Composable**:
使用 `createStore` 函数定义 store,然后在组件中使用 `useStore` 提供的 Composable 函数来获取或设置共享数据。
```javascript
import { defineStore } from "pinia";
const useGlobalState = defineStore("globalState", {
state: () => ({
sharedData: "",
}),
getters: {
getData: (state) => state.sharedData,
},
actions: {
setData(data) {
this.state.sharedData = data;
},
},
});
```
3. **在组件中使用 Composable**:
在需要使用共享数据的组件中,使用 `useStore()` 函数引用 store,并通过 `mapGetters` 或 `mapActions` 来映射 getters 和 actions 到组件的计算属性或方法。
```html
<template>
<!-- 使用 computed 属性 -->
{{ globalState.getData }}
<!-- 使用方法 -->
<button @click="updateData">Update Data</button>
</template>
<script setup lang="ts">
import { useGlobalState } from "../stores/globalState";
import { onMounted } from "vue";
const globalState = useGlobalState();
const updateData = () => {
globalState.setData("new value");
};
onMounted(() => {
console.log(globalState.getData); // 初始化时调用 getters 获取数据
});
</script>
```
以上就是 Vue3 不同页面间传递数据的基本方法,无论是通过 Vuex 还是使用 Composition API,都可以有效地实现在应用中的全局状态管理和数据通信。在选择使用哪种方法时,可以根据项目的大小、复杂度和个人偏好来决定。
阅读全文
相关推荐
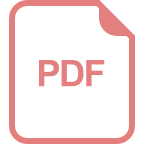
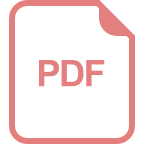


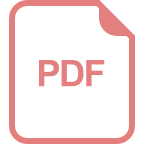
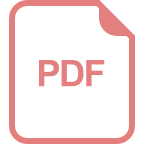
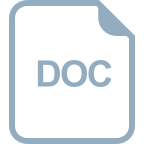










