请使用c++线性表的形式实现上述问题,注意程序输出:孩子们出列的次序
时间: 2024-09-15 12:01:51 浏览: 29
在C++中,线性表可以通过数组或者链表来实现。这里提供一个使用链表实现的简单例子,用于表示孩子们出队的次序。
首先定义一个孩子类,包含孩子的编号和指向下一个孩子的指针。然后创建一个链表,通过尾插法添加孩子节点。最后,使用循环遍历链表并输出每个孩子的编号,模拟孩子们出列的次序。
以下是C++代码实现:
```cpp
#include <iostream>
// 定义孩子节点结构体
struct Node {
int number; // 孩子的编号
Node* next; // 指向下一个孩子节点的指针
Node(int num) : number(num), next(nullptr) {}
};
// 定义线性表类
class LinearList {
private:
Node* head; // 指向链表头节点的指针
public:
LinearList() : head(nullptr) {}
// 添加孩子节点到链表尾部
void append(int number) {
Node* newNode = new Node(number);
if (!head) {
head = newNode;
} else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// 输出所有孩子出列的次序
void display() {
Node* current = head;
while (current) {
std::cout << current->number << " ";
Node* temp = current;
current = current->next;
delete temp; // 释放内存
}
std::cout << std::endl;
}
// 析构函数,用于释放链表内存
~LinearList() {
while (head) {
Node* temp = head;
head = head->next;
delete temp;
}
}
};
int main() {
LinearList children;
// 假设有5个孩子,编号为1到5
for (int i = 1; i <= 5; ++i) {
children.append(i);
}
// 输出孩子们出列的次序
children.display();
return 0;
}
```
该程序将创建一个包含5个孩子节点的链表,并模拟出队的过程,输出孩子们的编号。这个例子假设孩子按照编号顺序进队,出队次序也是按照编号顺序。
相关推荐
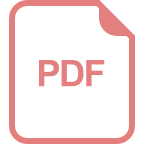
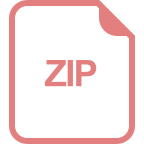
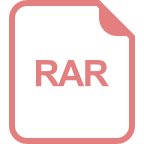
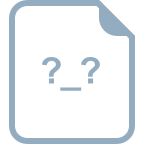
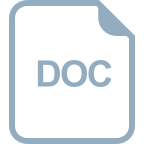
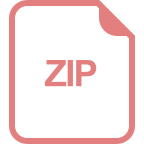
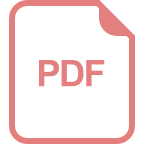
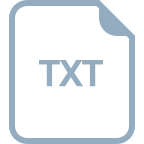
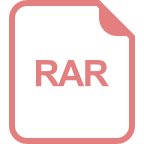
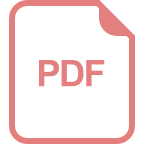
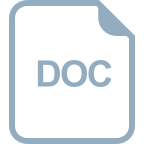
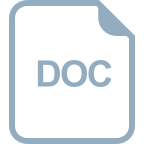
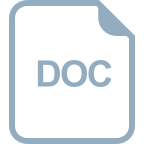
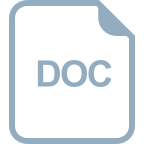