vue2.0中调用父组件的方法
时间: 2024-06-16 07:04:34 浏览: 64
在Vue 2.0中,可以通过自定义事件来调用父组件的方法。具体步骤如下:
1. 在子组件中,使用`$emit`方法触发一个自定义事件,并传递需要传递给父组件的数据。例如:
```javascript
this.$emit('customEvent', data);
```
2. 在父组件中,使用`v-on`指令监听子组件触发的自定义事件,并在对应的方法中处理数据。例如:
```html
<child-component v-on:customEvent="handleCustomEvent"></child-component>
```
```javascript
methods: {
handleCustomEvent(data) {
// 在这里处理从子组件传递过来的数据
}
}
```
这样,当子组件触发自定义事件时,父组件就会调用相应的方法,并传递子组件传递过来的数据。
相关问题
vue2.0 父组件调用子组件方法及获取子组件的值
在Vue 2.0中,父组件可以通过使用`ref`属性来获取子组件的实例,并且可以通过该实例调用子组件的方法和获取子组件的值。
首先,在父组件中给子组件添加一个`ref`属性,例如:
```html
<template>
<div>
<child-component ref="child"></child-component>
</div>
</template>
```
然后,在父组件的JavaScript代码中,可以通过`this.$refs`来访问子组件的实例,从而调用子组件的方法和获取子组件的值。例如:
```javascript
<script>
export default {
mounted() {
// 调用子组件的方法
this.$refs.child.childMethod();
// 获取子组件的值
const childValue = this.$refs.child.childValue;
console.log(childValue);
}
}
</script>
```
需要注意的是,父组件只能在子组件被挂载后才能访问到子组件的实例,所以在父组件的生命周期钩子函数`mounted`中进行操作是比较安全的。
Vue2.0主页调用子组件
在Vue2.0中,调用子组件需要在父组件中注册子组件,并在模板中使用标签来引用子组件。
步骤如下:
1.在父组件中注册子组件:
```javascript
import ChildComponent from './ChildComponent.vue'
export default {
components: {
'child-component': ChildComponent
},
//...
}
```
这里使用`import`语句导入子组件,并通过`components`选项将其注册为`child-component`。
2.在父组件模板中使用子组件:
```html
<template>
<div>
<child-component></child-component>
</div>
</template>
```
这里使用`<child-component>`标签来引用子组件。
注意:子组件的名称需要使用短横线分隔命名,而不是驼峰式命名。例如上述代码中的`child-component`而不是`ChildComponent`。
当父组件被渲染时,子组件也会被渲染,并显示在父组件中。
阅读全文
相关推荐
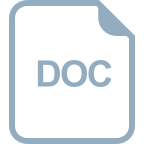
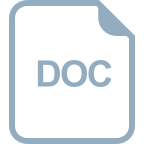
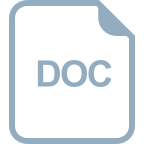
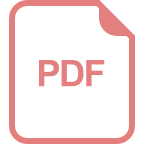
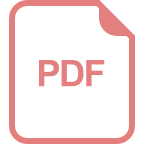
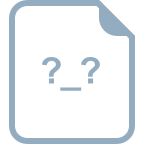
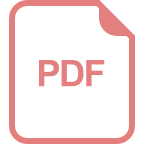
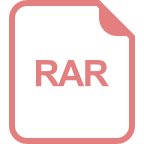
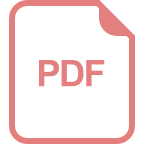
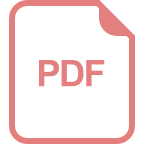
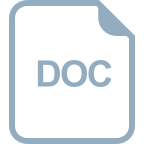
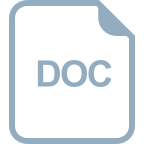
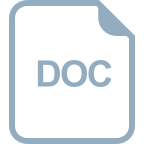
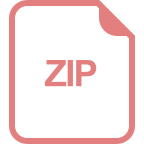
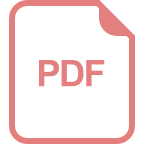
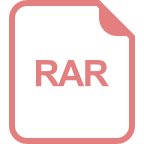