c++ 有3个泊位,每个泊位最多能停3辆车,如何设计数据结构来记录每个泊位的车辆数
时间: 2024-09-20 07:16:27 浏览: 47
在C++中,你可以使用数组或向量(vector)结合自定义结构体或类来设计数据结构,以记录每个泊位的车辆数。这里我们先创建一个简单的结构体:
```cpp
#include <iostream>
#include <vector>
// 定义一个表示停车位的结构体
struct ParkingSpot {
int capacity; // 泊车位的最大容量
int vehicles; // 当前停车数量
};
class ParkingLot {
private:
std::vector<ParkingSpot> spots; // 泊车区域,用向量存储
public:
void addVehicle(int spotIndex, int vehicle) {
if (spots[spotIndex].vehicles + vehicle <= spots[spotIndex].capacity) {
spots[spotIndex].vehicles += vehicle;
std::cout << "Added " << vehicle << " vehicles to spot " << spotIndex + 1 << ". Total: " << spots[spotIndex].vehicles << std::endl;
} else {
std::cout << "Spot " << spotIndex + 1 << " is full. Cannot add more vehicles." << std::endl;
}
}
void removeVehicle(int spotIndex, int vehicle) {
if (vehicle > spots[spotIndex].vehicles) {
std::cout << "Not enough vehicles in spot " << spotIndex + 1 << ". Skipping removal." << std::endl;
} else {
spots[spotIndex].vehicles -= vehicle;
std::cout << "Removed " << vehicle << " vehicles from spot " << spotIndex + 1 << ". Total: " << spots[spotIndex].vehicles << std::endl;
}
}
// 其他方法如查询、遍历等
};
int main() {
ParkingLot parking;
parking.addVehicle(0, 2); // 假设第一个泊位停了两辆车
parking.addVehicle(1, 1); // 第二个泊位停了一辆车
return 0;
}
```
在这个例子中,`ParkingLot` 类包含一个`spots` 向量,其中的每个元素是一个`ParkingSpot` 结构体,分别代表一个泊位。类提供了添加和移除车辆的方法,这些方法会检查泊位是否有足够的空间容纳额外的车辆或移出指定数量的车辆。
相关问题:
1. 如何在C++中动态分配多个泊车位?
2. 如果要支持泊位满的情况提示,如何修改代码逻辑?
3. 如何扩展这个数据结构以支持更复杂的泊车规则?
阅读全文
相关推荐






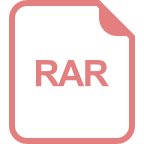
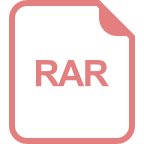









