springboot Qname
时间: 2024-10-20 21:04:51 浏览: 33
`QName`在Java Spring Boot中通常指的是命名空间URI(Namespace URI)和本地名称(Local Name)的组合,它在处理XML文档时特别重要,特别是在使用Spring框架中的`@XmlElement`、`@XmlType`等注解时。
在Spring MVC中,特别是当处理SOAP或RESTful Web Services以及基于XML配置时,`QName`用于表示XML元素的完整标识符,包括命名空间前缀和元素名。例如,`<person xmlns="http://www.example.com/ns" name="John Doe">` 中,`person`就是一个QName,其URI是"http://www.example.com/ns",本地名称是"name"。
当你在Java代码中创建`QName`实例,比如:
```java
import javax.xml.namespace.QName;
QName personName = new QName("http://www.example.com/ns", "person");
```
这表明了元素在命名空间"http://www.example.com/ns"下的名字是"person"。
相关问题
springboot cxf 客户端
您好!关于使用Spring Boot和Apache CXF开发客户端的问题,我可以为您提供一些指导。
首先,您需要在您的Spring Boot项目中添加CXF的依赖。可以在您的pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-spring-boot-starter-jaxws</artifactId>
<version>3.4.1</version>
</dependency>
```
接下来,您可以创建一个CXF客户端来调用远程WebService。您可以使用`@WebServiceClient`注解来生成客户端代码。例如,假设您要调用一个名为"HelloWorldService"的WebService:
```java
@WebServiceClient(name = "HelloWorldService",
targetNamespace = "http://example.com/",
wsdlLocation = "http://example.com/HelloWorldService?wsdl")
public class HelloWorldServiceClient extends Service {
public HelloWorldServiceClient(URL wsdlLocation, QName serviceName) {
super(wsdlLocation, serviceName);
}
public HelloWorldService getHelloWorldServicePort() {
return super.getPort(HelloWorldService.class);
}
}
```
在上面的代码中,`wsdlLocation`参数指定了远程WebService的WSDL地址。`getHelloWorldServicePort()`方法返回了一个用于调用WebService方法的接口。
接下来,您可以在您的Spring Boot应用程序中使用这个客户端。您可以将它注入到您的服务或控制器中,并使用它来调用远程WebService方法。例如:
```java
@Service
public class MyService {
@Autowired
private HelloWorldServiceClient helloWorldServiceClient;
public void invokeRemoteService() {
HelloWorldService helloWorldService = helloWorldServiceClient.getHelloWorldServicePort();
String result = helloWorldService.sayHello("World");
System.out.println(result);
}
}
```
在上面的代码中,通过`helloWorldServiceClient.getHelloWorldServicePort()`方法获取到了远程WebService的接口实例,然后可以调用其中的方法。
这就是使用Spring Boot和CXF开发客户端的基本步骤。您可以根据您的具体需求进一步进行配置和调整。希望对您有所帮助!如果您有更多问题,请随时提问。
springboot 调用SOAP接口
在Spring Boot中调用SOAP接口通常涉及到使用JAX-WS(Java API for XML Web Services)或Apache CXF这样的库。以下是一个简单的步骤指南:
1. 添加依赖:首先,在你的`pom.xml`文件中添加相关的依赖。如果你选择JAX-WS,可以添加以下依赖:
```xml
<dependencies>
...
<dependency>
<groupId>javax.xml.ws</groupId>
<artifactId>jaxws-api</artifactId>
<version>2.3.0</version>
</dependency>
...
</dependencies>
```
2. 创建客户端:创建一个`@WebServiceClient`注解的类,它将用于访问SOAP服务。例如:
```java
@WebServiceClient(targetNamespace = "http://yourwebservice.com", wsdlLocation = "file:///path/to/your/wsdl")
public class YourSoapService {
private final Service service;
// 使用JAXB创建一个代理对象
public YourSoapService() {
service = new JaxWsServiceFactoryBean().create(new QName("http://yourwebservice.com", "YourSoapService"));
}
// 定义访问某个SOAP操作的方法
public YourResponseType yourOperation(YourRequestType request) {
return service.getPort(YourPort.class).yourMethod(request);
}
}
```
3. 实现请求和响应类型:定义对应的请求和响应数据类,它们通常是从WSDL文档生成的。
4. 调用服务:现在你可以像调用普通方法一样调用你的SOAP服务了。
5. 错误处理:别忘了处理可能出现的异常,比如`WebServiceException`。
阅读全文
相关推荐
















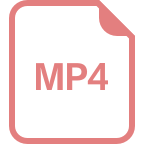